Question
using the given code to define the method removeAllOccurrences for the class LinkedBag that removes all occurrences of the given entries from a bag. Your
using the given code to define the method removeAllOccurrences for the class LinkedBag that removes all occurrences of the given entries from a bag. Your programs output must be identical to this output:
===========================BagInterface.java===================================
package asmt02part1;
/** * An interface that describes the operations of a bag of objects. * * **************************** PLEASE DO NOT CHANGE THIS FILE *********** * * @param */ public interface BagInterface {
/** * Gets the current number of entries in this bag. * * @return The integer number of entries currently in the bag. */ public int getCurrentSize();
/** * Sees whether this bag is empty. * * @return True if the bag is empty, or false if not. */ public boolean isEmpty();
/** * Adds a new entry to this bag. * * @param newEntry The object to be added as a new entry. * @return True if the addition is successful, or false if not. */ public boolean add(T newEntry);
/** * Removes all occurrences of the given entries * * @param entries */ public void removeAllOccurences(T[][] entries);
/** * Retrieves all entries that are in this bag. * * @return A newly allocated array of all the entries in the bag. Note: If * the bag is empty, the returned array is empty. */ public T[] toArray();
} // end BagInterface
=================================LinkedBag.java================================
package asmt02part1;
import java.util.Arrays;
/**
* A class of bags whose entries are stored in a chain of linked nodes. The bag
* is never full.
*
* @param
*/
public final class LinkedBag implements BagInterface {
private Node firstNode; // Reference to first node
private int numberOfEntries;
public LinkedBag() {
firstNode = null;
numberOfEntries = 0;
} // end default constructor
/**
* Gets the number of entries currently in this bag.
*
* @return The integer number of entries currently in this bag.
*/
@Override
public int getCurrentSize() {
return numberOfEntries;
} // end getCurrentSize
/**
* Sees whether this bag is empty.
*
* @return True if this bag is empty, or false if not.
*/
@Override
public boolean isEmpty() {
return numberOfEntries == 0;
} // end isEmpty
/**
* Adds a new entry to this bag.
*
* @param newEntry The object to be added as a new entry
* @return True if the addition is successful, or false if not.
*/
@Override
public boolean add(T newEntry) // OutOfMemoryError possible
{
// Add to beginning of chain:
Node newNode = new Node(newEntry);
newNode.next = firstNode; // Make new node reference rest of chain
// (firstNode is null if chain is empty)
firstNode = newNode; // New node is at beginning of chain
numberOfEntries++;
return true;
} // end add
/**
* Retrieves all entries that are in this bag.
*
* @return A newly allocated array of all the entries in this bag.
*/
@Override
public T[] toArray() {
// The cast is safe because the new array contains null entries
@SuppressWarnings("unchecked")
T[] result = (T[]) new Object[numberOfEntries]; // Unchecked cast
int index = 0;
Node currentNode = firstNode;
while ((index
result[index] = currentNode.data;
index++;
currentNode = currentNode.next;
} // end while
return result;
} // end toArray
// Locates a given entry within this bag.
// Returns a reference to the node containing the entry, if located,
// or null otherwise.
private Node getReferenceTo(T anEntry) {
boolean found = false;
Node currentNode = firstNode;
while (!found && (currentNode != null)) {
if (anEntry.equals(currentNode.data)) {
found = true;
} else {
currentNode = currentNode.next;
}
} // end while
return currentNode;
} // end getReferenceTo
/**
* Removes all occurrences of the given entries
*
*/
@Override
public void removeAllOccurences(T[][] entries) {
// Convert 2D array to 1D array
// Remove duplicates in array
// Remove all occurences of given items
} // end removeAllOccurences
private class Node {
private T data; // Entry in bag
private Node next; // Link to next node
private Node(T dataPortion) {
this(dataPortion, null);
} // end constructor
private Node(T dataPortion, Node nextNode) {
data = dataPortion;
next = nextNode;
} // end constructor
} // end Node
} // end LinkedBag
==================================LinkedBagTester.java==========================
package asmt02part1;
/** * A demonstration of the class LinkedBag. * * **************************** PLEASE DO NOT CHANGE THIS FILE *********** * */ public class LinkedBagTester {
public static void main(String[] args) { System.out.println("[+] Creating an empty bag..."); BagInterface aBag = new LinkedBag(); displayBag(aBag);
// Adding strings System.out.println("[+] Creating bag items..."); String[] contentsOfBag = {"A", " ", " ", "G", "Bb", "A", " ", "u", "n", "o", "A", "o", "d", "Bb", "A", "A", "l", "l"}; testAdd(aBag, contentsOfBag);
// Removing all occurence of the given entries from a bag System.out.println("[+] Creating a 2D testArray... \t"); String[][] testArray = { {"A", "A", "A", "A", "A", "A"}, {"B", "Bb", "Bb"}, {"C", " "}, {"n", "u", "l", "l"} }; for (String[] row : testArray) { System.out.print("\t\t\t\t\t"); for (String col : row) { System.out.print(col + " "); } System.out.println(""); }
System.out.println(""); System.out.println("[+] Removing testArray items from the bag..."); aBag.removeAllOccurences(testArray); displayBag(aBag);
} // end main
// Tests the method add. private static void testAdd(BagInterface aBag, String[] content) { System.out.print("[+] Adding the bag items to the bag: \t"); for (String content1 : content) { aBag.add(content1); System.out.print(content1 + " "); } // end for System.out.println();
displayBag(aBag); } // end testAdd
// Tests the method toArray while displaying the bag. private static void displayBag(BagInterface aBag) { System.out.print("- The bag now contains " + aBag.getCurrentSize() + " string(s): \t"); Object[] bagArray = aBag.toArray(); for (Object bagArray1 : bagArray) { System.out.print(bagArray1 + " "); } // end for
System.out.println(" "); } // end displayBag
} // end LinkedBagDemo
Define the method removeA110ccurrences for the class LinkedBag that removes all occurrences of the giver entries from a bag. Your program's output must be identical to this output: | [+] creating an empty - The bag now contains 0 string (s) bag [+1 Creating bag items... [+ Adding the bag items to the bag: A G Bb A un o A o d Bb A A 1 1 - The bag now contains 18 string (s)1 1 A A Bb d o Aonu A Bb G A +1 Creating a 2D te stArray... B Bb Bb n ui i [+] Removing testArray items from the bag. .. - The bag now contains 4 string (s) Go o d Define the method removeA110ccurrences for the class LinkedBag that removes all occurrences of the giver entries from a bag. Your program's output must be identical to this output: | [+] creating an empty - The bag now contains 0 string (s) bag [+1 Creating bag items... [+ Adding the bag items to the bag: A G Bb A un o A o d Bb A A 1 1 - The bag now contains 18 string (s)1 1 A A Bb d o Aonu A Bb G A +1 Creating a 2D te stArray... B Bb Bb n ui i [+] Removing testArray items from the bag. .. - The bag now contains 4 string (s) Go o dStep by Step Solution
There are 3 Steps involved in it
Step: 1
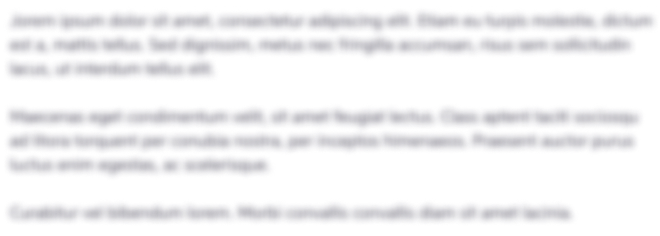
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started