Question
Using the Java code below, Please help me create an implementation that passes the tests provided. Main public class SequenceSearchImpl extends SequenceSearch { public SequenceSearchImpl(final
Using the Java code below, Please help me create an implementation that passes the tests provided.
Main
public class SequenceSearchImpl extends SequenceSearch {
public SequenceSearchImpl(final String content, final String start, final String end) { super(content, start, end); }
@Override public String[] getAllTaggedSequences() { return null; }
@Override public String getLongestTaggedSequence() {
return null; }
@Override public String displayStringArray() { return null; }
@Override public String toString() { return null; }
}
public abstract class SequenceSearch implements Presentable {
final String startTag; final String endTag; final String content;
/** * The one and only constructor * @param content {@link String} content with the some tags inside * @param startTag {@link String} * @param endTag {@link String} */ public SequenceSearch(final String content, final String startTag, final String endTag) { if (content == null || startTag == null || endTag == null) { throw new IllegalArgumentException("Values for neither Content nor Start nor End can be null."); } if (!startTag.equals(endTag)) { // which is ok ... if (startTag.contains(endTag) || endTag.contains(startTag)) { // .. but it's not OK that the startTag contains the endtag or vice versa. throw new IllegalArgumentException("Start and end tag are allowed to be the same, but otherwise must not contain each other."); } } this.content = content; this.startTag = startTag; this.endTag = endTag; }
/** * Static helper method, "adds" the given Strings s a String Array, by creating a new longer StringArray. * * @param a {@link String[]} source string array * @param s {@link String} that needs to be added * @return {@link String[]} newly created string array, containing all the elements from the provided array, plus the new string. */ static String[] adds(final String[] a, final String s) { final String[] sa; if (a!=null && 0 sa = new String[a.length + 1]; System.arraycopy(a, 0, sa, 0, a.length); sa[a.length] = s; } else { sa = new String[]{s}; } return sa; }
/** * @return {@link String[]} of all sub-string, within the content, which are enclosed, in startTag and endTag. * If no tagged sub-strings are found, an empty String[] is returned. */ public abstract String[] getAllTaggedSequences();
/** * @return {@link String} the longest sub-string within the content, which is enclosed, in startTag and endTag. * If unlcear, the substring that appears last in the content will be returned. * If no tagged sequence was found, null is returned. */ public abstract String getLongestTaggedSequence();
}
public interface Presentable {
String displayStringArray(); }
Test Code
import org.junit.Test;
import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertNull; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue;
public class SequenceSearchTest {
private String s0 = "%sJava%s is a %sprogramming language%s created by %sJames Gosling%s from %sSun Microsystems%s " + "(Sun) in 1991. The target of Java is to write a program once and then run this program on multiple " + "operating systems. The first publicly available version of Java (Java 1.0) was released in 1995. " + "Sun Microsystems was acquired by the Oracle Corporation in 2010. " + "Oracle has now the steermanship for Java. In 2006 Sun started to make Java available under the " + "GNU General Public License (GPL). Oracle continues this project called %sOpenJDK.%s";
private String s1 = "Java is a %sprogramming language%s created by %sJames Gosling%s from %sSun Microsystems%s " + "(Sun) in 1991. The target of Java is to write a program once and then run this program on multiple " + "operating systems. The first publicly available version of Java (Java 1.0) was released in 1995. " + "Sun Microsystems was acquired by the Oracle Corporation in 2010. " + "Oracle has now the steermanship for Java. In 2006 Sun started to make Java available under the " + "GNU General Public License (GPL). Oracle continues this project called %sOpenJDK.%s";
private String s2 = "Java is a %sprogramming language%s created by %sJames Gosling%s from %sSun Microsystems%s " + "(Sun) in 1991. The target of Java is to write a program once and then run this program on multiple " + "operating systems. The first publicly available version of Java (Java 1.0) was released in 1995. " + "Sun Microsystems was acquired by the Oracle Corporation in 2010. " + "Oracle has now the steermanship for Java. In 2006 Sun started to make Java available under the " + "GNU General Public License (GPL). Oracle continues this project called OpenJDK.";
private String s3 = "%sJava%s is a programming language created by James Gosling from Sun Microsystems " + "(Sun) in 1991. The target of Java is to write a program once and then run this program on multiple " + "operating systems. The first publicly available version of Java (Java 1.0) was released in 1995. " + "Sun Microsystems was acquired by the Oracle Corporation in %s2010%s. " + "Oracle has now the steermanship for Java. In 2006 Sun started to make Java available under the " + "GNU General Public License (GPL). Oracle continues this project called OpenJDK.";
private String s4 = "Java is a programming language created by James Gosling from Sun Microsystems " + "(Sun) in 1991. The target of Java is to write a program once and then run this program on multiple " + "operating systems. The first publicly available version of Java (Java 1.0) was released in 1995. " + "Sun Microsystems was acquired by the Oracle Corporation in 2010. " + "Oracle has now the steermanship for Java. In 2006 Sun started to make Java available under the " + "GNU General Public License (GPL). Oracle continues this project called OpenJDK.";
// The here tested implementation is in the abstract class and this test is shown here, only for clarification @Test public void constructionTest() { String s = "abc def ghi"; // tags can have different length; SequenceSearch s1 = new SequenceSearchImpl(s, "a", "bc"); // tags can be the same SequenceSearch s2 = new SequenceSearchImpl(s, "a", "bc"); // tags cannot contain tags try { SequenceSearch s3 = new SequenceSearchImpl(s, "a", "ab"); assertTrue(false); } catch (Exception e) { assertTrue(true); } try { SequenceSearch s4 = new SequenceSearchImpl(s, "ac", "c"); assertTrue(false); } catch (Exception e) { assertTrue(true); } }
// Finding all text sequences that are enclosed in beginning and end tags. @Test public void getAllTaggedSequences() throws Exception {
final String d0 = "<"; final String d1 = "/>";
String s = String.format(s0, d0, d1, d0, d1, d0, d1, d0, d1, d0, d1); String[] sa = new SequenceSearchImpl(s, d0, d1).getAllTaggedSequences(); assertEquals("Java", sa[0]); assertEquals("programming language", sa[1]); assertEquals("James Gosling", sa[2]); assertEquals("Sun Microsystems", sa[3]); assertEquals("OpenJDK.", sa[4]);
s = String.format(s1, d0, d1, d0, d1, d0, d1, d0, d1); sa = new SequenceSearchImpl(s, d0, d1).getAllTaggedSequences(); assertEquals("programming language", sa[0]); assertEquals("James Gosling", sa[1]); assertEquals("Sun Microsystems", sa[2]); assertEquals("OpenJDK.", sa[3]);
s = String.format(s2, d0, d1, d0, d1, d0, d1); sa = new SequenceSearchImpl(s, d0, d1).getAllTaggedSequences(); assertEquals("programming language", sa[0]); assertEquals("James Gosling", sa[1]); assertEquals("Sun Microsystems", sa[2]); }
// Finding the longest of all tagged sequences. @Test public void getLongestTaggedSequence() throws Exception { String d0 = "{{"; String d1 = "}}";
String s = String.format(s2, d0, d1, d0, d1, d0, d1); String t = new SequenceSearchImpl(s, d0, d1).getLongestTaggedSequence();
assertEquals("programming language", t); // find the later one, if same length s = String.format(s3, d0, d1, d0, d1); t = new SequenceSearchImpl(s, d0, d1).getLongestTaggedSequence();
assertEquals("2010", t);
// no tags in content whatsoever. s = String.format(s4, d0, d1); assertEquals(0, new SequenceSearchImpl(s, d0, d1).getAllTaggedSequences().length);
t = new SequenceSearchImpl(s, d0, d1).getLongestTaggedSequence(); assertNull(t); }
// Making sure that all sequences are found, even if start and end tags look the same. @Test public void getAllTaggedSequencesEqualDelimiters() throws Exception {
String d = "#";
String s = String.format(s0, d, d, d, d, d, d, d, d, d, d); String[] sa = new SequenceSearchImpl(s, d, d).getAllTaggedSequences(); assertEquals("Java", sa[0]); assertEquals("programming language", sa[1]); assertEquals("James Gosling", sa[2]); assertEquals("Sun Microsystems", sa[3]); assertEquals("OpenJDK.", sa[4]);
d = "##"; s = String.format(s1, d, d, d, d, d, d, d, d); sa = new SequenceSearchImpl(s, d, d).getAllTaggedSequences(); assertEquals("programming language", sa[0]); assertEquals("James Gosling", sa[1]); assertEquals("Sun Microsystems", sa[2]); assertEquals("OpenJDK.", sa[3]);
d = "###"; s = String.format(s2, d, d, d, d, d, d); sa = new SequenceSearchImpl(s, d, d).getAllTaggedSequences(); assertEquals("programming language", sa[0]); assertEquals("James Gosling", sa[1]); assertEquals("Sun Microsystems", sa[2]); }
// Interface Implementation @Test public void testPresentable() { String d = "###"; String s = String.format(s2, d, d, d, d, d, d); String p = new SequenceSearchImpl(s, d, d).displayStringArray(); assertTrue(p.startsWith("programming language : 20 ")); assertTrue(p.endsWith("Sun Microsystems : 16 ")); }
// ToString prints the content, with all tags removed. @Test public void testToString() { String d0 = "<"; String d1 = "/>"; String s = String.format(s2, d0, d1, d0, d1, d0, d1); String p = new SequenceSearchImpl(s, d0, d1).toString(); assertFalse(p.contains(d0)); assertFalse(p.contains(d1)); assertTrue(p.startsWith("Java is a programming language created by James Gosling")); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
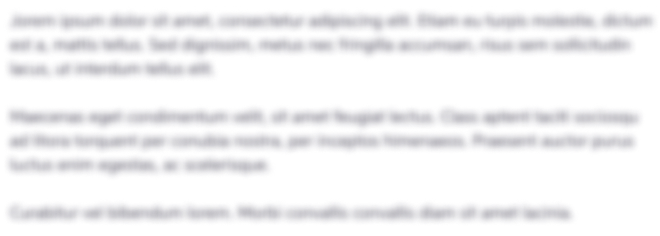
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started