Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using the RPG combat code, add the following functionality: Create a class called Potion Potions are used to heal a character The potion should have
Using the RPG combat code, add the following functionality:
Create a class called "Potion"
Potions are used to heal a character
The potion should have 2 private members: count and healingAmount
count holds the number of potions the player has available
healingAmount holds the amount of health recovered by the character
The potion constructor should allow the programmer to specify how many potions are available
The class should have a member function called "use"
the use function should be passed a character pointer or reference for the character being healed
the function should invoke the heal member function of the character using the potion
when the use function is called, the potion count should drop by 1
if the count value is 0 or lower, no healing should be performed
The class should have an accesser function called getCount() which returns the number of potions available
The combat code should now prompt the user whether they want to attack the creature or use a potion
if the user selects to use a potion, the npc/enemy character should still attack them
if the playerCharacter is slower than the npc/enemy character, they should be attacked before they get the chance to heal
you may collect user input to select attack or use potion however you want (collect a 1 or 2 from the user, etc.)
Add a name string member to the Character class
Add a string called "name" to the character class
Create an accesser function called getName() to the Character class
Allow the name to be set in the constructor for Character
(BONUS!) Add some flavor text to describe the premise for how the encounter began (some short story as to how the player ended up fighting the enemy character)
1-#include "character.h"
using namespace std;
// Constructors
Character::Character(){
healthPoints = 0;
strength = 0;
defense = 0;
speed = 0;
maxHp = 0;
}
Character::Character(int hp, int str, int def, int spd){
if(hp < 0) healthPoints = 0;
else healthPoints = hp;
if(str < 0) strength = 0;
else strength = str;
if(def < 0) defense = 0;
else defense = def;
if(spd < 0) speed = 0;
else speed = spd;
maxHp = healthPoints;
}
// Action Member Functions
int Character::attack(Character & c){
c.defend(strength);
}
void Character::defend(int damage){
int damageReceived = damage - defense;
if(damageReceived < 0)
damageReceived = 0;
healthPoints = healthPoints - damageReceived;
if(healthPoints < 0)
healthPoints = 0;
}
void Character::heal(int healing){
healthPoints += healing;
if(healthPoints > maxHp)
healPoints = maxHp;
}
// Status Member Functions:
bool Character::isDead(void){
if(healthPoints <= 0)
return true;
else
return false;
}
int Character::getSpeed(){
return speed;
}
}
2-#include
class Character{
// Private data members (these are only accessible from inside the class)
private:
int maxHp;
int healthPoints;
int strength;
int defense;
int speed;
// Public data members and member functions (these are accessible anywhere)
public:
Character(void);
Character(int, int, int, int);
int attack(void);
void defend(int);
void heal(int);
bool isDead(void);
int getSpeed(void);
};
3-#include
#include "character.h"
using namespace std;
int main(int argc, char * argv[]){
Character playerCharacter(10, 5, 3, 5);
Character npc(10, 4, 4, 3);
Potion p(3);
while(!playerCharacter.isDead() && !npc.isDead()){
if(playerCharacter.getSpeed() > npc.getSpeed()){
// player character attack
npc.defend(playerCharacter.attack());
if(!npc.isDead())
playerCharacter.defend(npc.attack());
} else {
// npc attack
playerCharacter.defend(npc.attack());
if(!playerCharacter.isDead())
npc.defend(playerCharacter.attack());
}
}
if(!playerCharacter.isDead())
cout << "Player wins!" << endl;
else
cout << "Computer wins!" << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
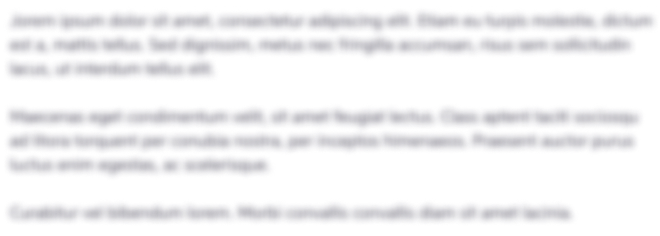
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started