Question
// Using the startup code, implement the following: // 1-Create List L1 of 10 elements (task IDs), e.g. L1 = 1, 2, 3, 4, 5,
// Using the startup code, implement the following: // 1-Create List L1 of 10 elements (task IDs), e.g. L1 = 1, 2, 3, 4, 5, 6, 7, 8, 9, 10; // // 2-Insert a new list element in between (at every other element), such as: 1,100, 2,200, 3,300, 4,400, ... 10,1000; // Simulate a new CPU core assignment: // // 3-Create another List L2, and extract the newly inserted elements into this new list L2 // i.e. L1 should go back to the original list // L2 should be: 100, 200, 300, 400, 500, 600, 700, 800, 900, 1000 // 4-Create a Queue Q1 by extracting L1, and inserting L2 elements: i.e. Q should start with: 1,2,3, ... 10, and (10, 20 ... 100) will be inserted // Q1=1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 100, 200, 300, 400, 500, 600, 700, 800, 900, 1000 // Label tasks as finished with the possibility of revisiting a task, such as: // 5-Create a Stack S1 which by deleting the elements of Q1 and inserting them in L1 // 6-Pop out all elements of the Stack, i.e. should end up with an empty stack // Extra credit: Simulate a re-executed task, i.e. Pop out a task out of the Stack and reinsert, this simulate a failed task
Lab3.cpp:
template
class Stack { public: bool isEmpty() const { return theList.empty(); } const Object& top() const { return theList.front(); } void push(const Object& x) { theList.push_front(x); } void pop(Object& x) { x = theList.front(); theList.pop_front(); } private: List
template
int main() { int i; const int N = 10;
List
List
// List Section example: //Q1: Create List L1 of 10 elements, e.g. L1 = 1, 2, 3, 4, 5, 6, 7, 8, 9, 10; // create the list array as a starting point: for (i = N; i > 0; --i) { L1.push_front(i); } // show L1 with an iterator cout << "List L1 is:" << endl; for (auto it = L1.begin(); it != L1.end(); ++it) { cout << *it << ' '; } // Stack to be created per the above assignment requirment cout << " Stack S1 is:" << endl;; for (i = N - 1; i >= 0; --i) { S1.push(i); cout << S1.top() << " " << endl; } while (!S1.isEmpty()) { cout << S1.top() << endl; S1.pop(i); } // Queue section, to be created per the above assignment requirment
return 0; } List.h
Please answer with C++ code thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
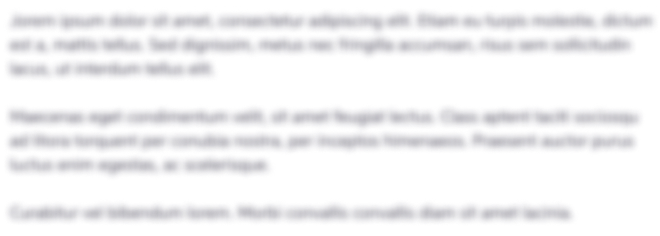
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started