Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using the two files included please build a main implentation only! queueAsArray.h queueADT.h //Header file QueueAsArray #ifndef H_QueueAsArray #define H_QueueAsArray #include #include #include queueADT.h using
Using the two files included please build a main implentation only!
queueAsArray.h queueADT.h
//Header file QueueAsArray #ifndef H_QueueAsArray #define H_QueueAsArray #include#include #include "queueADT.h" using namespace std; //************************************************************* // Author: D.S. Malik // // This class specifies the basic operation on a queue as an // array. //************************************************************* template class queueType : public queueADT { public: const queueType & operator=(const queueType &); //Overload the assignment operator. bool isEmptyQueue() const; //Function to determine whether the queue is empty. //Postcondition: Returns true if the queue is empty, // otherwise returns false. bool isFullQueue() const; //Function to determine whether the queue is full. //Postcondition: Returns true if the queue is full, // otherwise returns false. void initializeQueue(); //Function to initialize the queue to an empty state. //Postcondition: The queue is empty Type front() const; //Function to return the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the first element of the // queue is returned. Type back() const; //Function to return the last element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the last element of the queue // is returned. void addQueue(const Type& queueElement); //Function to add queueElement to the queue. //Precondition: The queue exists and is not full. //Postcondition: The queue is changed and queueElement is // added to the queue. void deleteQueue(); //Function to remove the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: The queue is changed and the first element // is removed from the queue. queueType(int queueSize = 100); //Constructor queueType(const queueType & otherQueue); //Copy constructor ~queueType(); //Destructor private: int maxQueueSize; //variable to store the maximum queue size int count; //variable to store the number of //elements in the queue int queueFront; //variable to point to the first //element of the queue int queueRear; //variable to point to the last //element of the queue Type *list; //pointer to the array that holds //the queue elements }; template bool queueType ::isEmptyQueue() const { return (count == 0); } //end isEmptyQueue template bool queueType ::isFullQueue() const { return (count == maxQueueSize); } //end isFullQueue template void queueType ::initializeQueue() { queueFront = 0; queueRear = maxQueueSize - 1; count = 0; } //end initializeQueue template Type queueType ::front() const { assert(!isEmptyQueue()); return list[queueFront]; } //end front template Type queueType ::back() const { assert(!isEmptyQueue()); return list[queueRear]; } //end back template void queueType ::addQueue(const Type& newElement) { if (!isFullQueue()) { queueRear = (queueRear + 1) % maxQueueSize; //use mod //operator to advance queueRear //because the array is circular count++; list[queueRear] = newElement; } else cout << "Cannot add to a full queue." << endl; } //end addQueue template void queueType ::deleteQueue() { if (!isEmptyQueue()) { count--; queueFront = (queueFront + 1) % maxQueueSize; //use the //mod operator to advance queueFront //because the array is circular } else cout << "Cannot remove from an empty queue" << endl; } //end deleteQueue //Constructor template queueType ::queueType(int queueSize) { if (queueSize <= 0) { cout << "Size of the array to hold the queue must " << "be positive." << endl; cout << "Creating an array of size 100." << endl; maxQueueSize = 100; } else maxQueueSize = queueSize; //set maxQueueSize to //queueSize queueFront = 0; //initialize queueFront queueRear = maxQueueSize - 1; //initialize queueRear count = 0; list = new Type[maxQueueSize]; //create the array to //hold the queue elements } //end constructor //Destructor template queueType ::~queueType() { delete[] list; } //end destructor template const queueType & queueType ::operator= (const queueType & otherQueue) { int j; if (this != &otherQueue) //avoid self-copy { maxQueueSize = otherQueue.maxQueueSize; queueFront = otherQueue.queueFront; queueRear = otherQueue.queueRear; count = otherQueue.count; delete[] list; list = new Type[maxQueueSize]; //copy other queue in this queue if (count != 0) for (j = queueFront; j <= queueRear; j = (j + 1) % maxQueueSize) list[j] = otherQueue.list[j]; } //end if return *this; } //end assignment operator template queueType ::queueType(const queueType & otherQueue) { maxQueueSize = otherQueue.maxQueueSize; queueFront = otherQueue.queueFront; queueRear = otherQueue.queueRear; count = otherQueue.count; list = new Type[maxQueueSize]; //copy other queue in this queue for (int j = queueFront; j <= queueRear; j = (j + 1) % maxQueueSize) list[j] = otherQueue.list[j]; } //end copy constructor #endif
//Header file: stackADT.h #ifndef H_queueADT #define H_queueADT //************************************************************* // Author: D.S. Malik // // This class specifies the basic operations on a queue. //************************************************************* templateclass queueADT { public: virtual bool isEmptyQueue() const = 0; //Function to determine whether the queue is empty. //Postcondition: Returns true if the queue is empty, // otherwise returns false. virtual bool isFullQueue() const = 0; //Function to determine whether the queue is full. //Postcondition: Returns true if the queue is full, // otherwise returns false. virtual void initializeQueue() = 0; //Function to initialize the queue to an empty state. //Postcondition: The queue is empty virtual Type front() const = 0; //Function to return the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the first element of the queue // is returned. virtual Type back() const = 0; //Function to return the last element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the last element of the queue // is returned. virtual void addQueue(const Type& queueElement) = 0; //Function to add queueElement to the queue. //Precondition: The queue exists and is not full. //Postcondition: The queue is changed and queueElement is // added to the queue. virtual void deleteQueue() = 0; //Function to remove the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: The queue is changed and the first element // is removed from the queue. }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
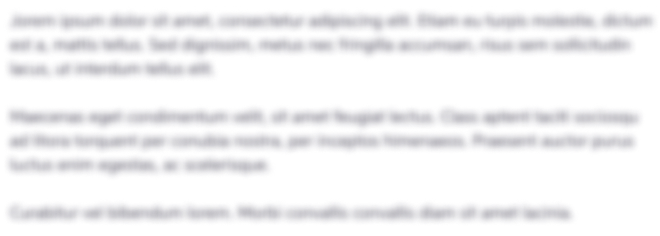
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started