Answered step by step
Verified Expert Solution
Question
1 Approved Answer
using this================================= /** * Node class used for implementing your LinkedDeque. * * DO NOT MODIFY THIS FILE!! * */ public class LinkedNode { private
using this=================================
/** * Node class used for implementing your LinkedDeque. * * DO NOT MODIFY THIS FILE!! * */ public class LinkedNode{ private T data; private LinkedNode previous; private LinkedNode next; /** * Creates a new LinkedNode with the given T object and node references. * * @param previous the previous node in the list * @param data the data stored in the new node * @param next the next node in the list */ public LinkedNode(LinkedNode previous, T data, LinkedNode next) { this.previous = previous; this.data = data; this.next = next; } /** * Creates a new LinkedNode with only the given T object. * * @param data the data stored in the new node */ public LinkedNode(T data) { this(null, data, null); } /** * Gets the data stored in the node. * * @return the data in this node */ public T getData() { return data; } /** * Gets the next node. * * @return the next node */ public LinkedNode getNext() { return next; } /** * Sets the next node. * * @param next the new next node */ public void setNext(LinkedNode next) { this.next = next; } /** * Gets the previous node. * * @return the previous node */ public LinkedNode getPrevious() { return previous; } /** * Sets the previous node. * * @param previous the new previous node */ public void setPrevious(LinkedNode previous) { this.previous = previous; } @Override public String toString() { return "Node containing: " + data; } }
please complete this==========================
public class ArrayDeque{ /** * The initial capacity of the ArrayDeque. */ public static final int INITIAL_CAPACITY = 11; // Do not add new instance variables. private T[] backingArray; private int front; private int back; private int size; /** * Constructs a new ArrayDeque with an initial capacity of * the {@code INITIAL_CAPACITY} constant above. */ public ArrayDeque() { } /** * Adds the data to the front of the deque. * * If sufficient space is not available in the backing array, you should * regrow it to double the current capacity. If a regrow is necessary, * you should copy elements to the beginning of the new array and reset * front to the beginning of the array (and move {@code back} * appropriately). After the regrow, the new data should be at index 0 of * the array. * * This method must run in amortized O(1) time. * * @param data the data to add to the deque * @throws java.lang.IllegalArgumentException if data is null */ public void addFirst(T data) { } /** * Adds the data to the back of the deque. * * If sufficient space is not available in the backing array, you should * regrow it to double the current capacity. If a regrow is necessary, * you should copy elements to the front of the new array and reset * front to the beginning of the array (and move {@code back} * appropriately). * * This method must run in amortized O(1) time. * * @param data the data to add to the deque * @throws java.lang.IllegalArgumentException if data is null */ public void addLast(T data) { } /** * Removes the data at the front of the deque. * * Do not shrink the backing array. * * If the deque becomes empty as a result of this call, you should * explicitly reset front and back to the beginning of the array. * * You should replace any spots that you remove from with null. Failure to * do so will result in a major loss of points. * * This method must run in O(1) time. * * @return the data formerly at the front of the deque * @throws java.util.NoSuchElementException if the deque is empty */ public T removeFirst() { } /** * Removes the data at the back of the deque. * * Do not shrink the backing array. * * If the deque becomes empty as a result of this call, you should * explicitly reset front and back to the beginning of the array. * * You should replace any spots that you remove from with null. Failure to * do so will result in a major loss of points. * * This method must run in O(1) time. * * @return the data formerly at the back of the deque * @throws java.util.NoSuchElementException if the deque is empty */ public T removeLast() { } /** * Returns the smallest non-negative remainder when dividing {@code index} * by {@code modulo}. So, for example, if modulo is 5, then this method will * return either 0, 1, 2, 3, or 4, depending on what the remainder is. * * This differs from using the % operator in that the % operator returns * the smallest answer with the same sign as the dividend. So, for example, * (-5) % 6 => -5, but with this method, mod(-5, 6) = 1. * * Examples: * mod(-3, 5) => 2 * mod(11, 6) => 5 * mod(-7, 7) => 0 * * DO NOT MODIFY THIS METHOD. This helper method is here to make the math * part of the circular behavior easier to work with. * * @param index the number to take the remainder of * @param modulo the divisor to divide by * @return the remainder in its smallest non-negative form * @throws java.lang.IllegalArgumentException if the modulo is non-positive */ private static int mod(int index, int modulo) { // DO NOT MODIFY! if (modulo <= 0) { throw new IllegalArgumentException("The modulo must be positive."); } else { int newIndex = index % modulo; return newIndex >= 0 ? newIndex : newIndex + modulo; } } /** * Returns the number of elements in the deque. * * Runs in O(1) for all cases. * * DO NOT USE THIS METHOD IN YOUR CODE. * * @return the size of the list */ public int size() { // DO NOT MODIFY! return size; } /** * Returns the backing array of this deque. * Normally, you would not do this, but we need it for grading your work. * * DO NOT USE THIS METHOD IN YOUR CODE. * * @return the backing array */ public T[] getBackingArray() { // DO NOT MODIFY THIS METHOD! return backingArray; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
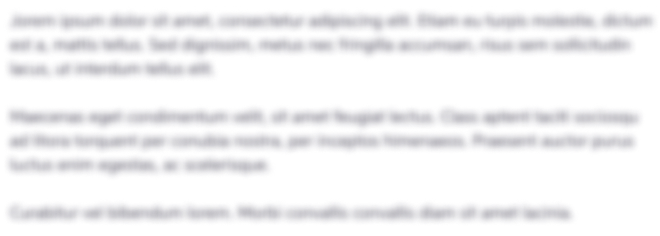
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started