Question
VIRTUAL MACHINE Task 1: Write a program that will read in numbers until the value -1 is entered on the keyboard. Call this program project1.asm
VIRTUAL MACHINE
Task 1:
Write a program that will read in numbers until the value -1 is entered on the keyboard. Call this
program project1.asm
Task 2:
Make a copy of your project1.asm file called project2.asm using cp project1.asm project2.asm on the shell
command line. This program should read in numbers until -1 is entered and then display the sum of all
single digit numbers that were entered.
Task 3:
Make a copy of project2.asm and call it project3.asm. This final version should read in all values and AFTER
the -1 is entered, it should display all of the numbers entered followed by printing out the sum of these
numbers. The stack must be used.
VIRTUAL MACHINE INSTRUCTIONS
Instructions
ADD (1, ACC = ACC +arg)
BR (1, jump to arg)
BRNEG (1, jump to arg if ACC<0)
BRZNEG (1, jump to arg if ACC<= 0)
BRPOS (1, jump to arg if ACC>0)
BRZPOS (1, jump to arg if ACC>= 0)
BRZERO (1, jump to arg if ACC == 0)
COPY (2, arg1 = arg2)
DIV (1, ACC = ACC / arg)
MULT (1, ACC = ACC * arg)
READ (1, arg=input integer)
WRITE (1, put arg to output as integer)
STOP (0, stop program)
STORE (1, arg = ACC)
SUB (1, ACC = ACC - arg)
NOOP (0, nothing)
LOAD (1, ACC=arg)
ADD, DIV, MULT, WRITE, LOAD, SUB can take either variable or immediate value
as the arg: immediate value is positive integer or negative integer
- PUSH (0, tos++)
- POP (0, tos)
- STACKW (1,stack[tos-arg]=ACC)
- STACKR (1,ACC=stack[tos-arg])
- PUSH/POP are only means to reserve/delete automatic storage.
- STACKW/STACKR n - these are stack write/read instructions. n must be a non-negative number, and the access is to nth element down from TOS, top of stack.
NOTE: tos points to the topmost element on the stack
Storage directives
XXX val
XXX is a name
val is the initial value
all storage and ACC size are signed 2 bytes
Storage name and label are all names starting with latter and following with letters and digits up to eight total
Assumptions
1. any proper format within line, tokens separated by BS, blank space
2. all storage directives are listed following the last STOP
3. all names start with letters and contain more letters or digits
3 Invocation
>v i r t M a c h //read from s t d i n
>v i r t M a c h f i l e . asm //read from f i l e . asm
EXAMPLE:
Example:
sumOf3.asm
reads 3 arguments and returns the sum using a stack
READ X
PUSH
LOAD X
STACKW 0
READ X
PUSH
LOAD X
STACKW 0
READ X
STACKR 1
ADD X
STORE X
STACKR 0
ADD X
STORE X
WRITE X
POP
POP
STOP
X 0
Example:
sum3nostack.asm
Step by Step Solution
There are 3 Steps involved in it
Step: 1
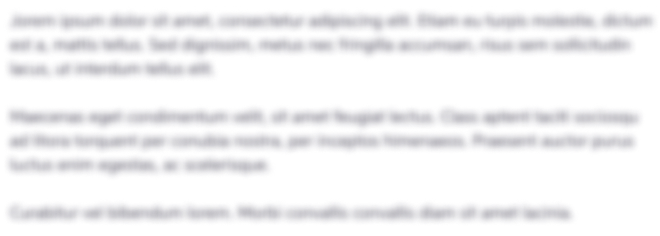
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started