Question
Visual Studio Console app - Interfaces (Active Server Pages) - URGENT ASSISTANCE NEEDED 1. Create a class called Student a. Create auto-implemented properties for FirstName
Visual Studio Console app - Interfaces (Active Server Pages) - URGENT ASSISTANCE NEEDED
1. Create a class called Student
a. Create auto-implemented properties for FirstName and LastName
b. In Main, create 2 instances of this class called student1 and student2, and set the names to: Bob Willis and Wilma Atkinson, respectively. Try this way of passing values to public properties in your class, since you dont have a constructor that takes 2 arguments: Widget w = new Widget() {prop1 = value1, prop2 = value2}; Note: if prop1 and prop2 are read-only, or are private, this method wont work, and you would have to create a constructor.
c. Next, write an if statement to compare the 2 objects: if( student1 > student2 ) Console.WriteLine(Student 1 is greater); else if( student 2 > student1 ) Console.WriteLine(Student 2 is greater); else Console.WriteLine(Both students are the same);
d. Notice the error in the if condition: we cant compare Student objects with relational operators (without some extra code that is beyond the scope of this assignment).
e.. Modify the if condition to: if ( student1.LastName > student2.LastName )
2. The CompareTo method
The String class has a method called CompareTo. It is built to handle >, <, and ==. If we can determine these 3 things, then we can also determine >=, <=, and !=.
The CompareTo method returns an integer number. If the returned number is greater than 0, then the object calling CompareTo is larger. If the returned number is less than 0, then the object calling CompareTo is smaller. If the returned number is equal to 0, then the object calling CompareTo is the same as the object being compared.
public int CompareTo(String anotherString) { if(this.value > anotherString.value) return 1; else if(this.value < anotherString.value) return -1; else return 0; }
Then a call to the method would look like this:
string s1 = Hello; string s2 = World; if( s1.CompareTo( s2 ) > 0 ) Console.WriteLine(s1 is greater);
So, the rule is to compare the return value to 0 using the same relational operator you would logically use to compare the 2 strings:
s1 < s2 is equivalent to s1.CompareTo(s2) < 0 s1 != s2 is equivalent to s1.CompareTo(s2) != 0
Modify your previous Main method to use the CompareTo method of the String class. Dont forget that you are comparing the LastName properties, NOT the entire Student objects (yet). Also, dont forget that the CompareTo method returns an integer, NOT a boolean value. You must compare it to 0.
3. DuoArray Class
Now well create a class that can store exactly 2 strings, and can sort them. Our class will store 2 items just to keep it simple, but the ideas are extendable to more useful classes.
Create a class called DuoArray.
a. Add 2 auto-implemented string properties called Value1 and Value2
b. Create a constructor that takes 2 string parameters, and assigns them to the above properties
c. Create a private swap method that swaps the contents of the properties. It takes no parameters, and returns nothing. No if logic involved...just swap the values in Value1 and Value2. Create a temporary, local string variable to do the swap.
d. Create a public sort method that evaluates Value1 and Value2. The method takes no arguments, and returns nothing. If Value1 is greater than Value2, call the swap method. Since they are strings, use the CompareTo method to do this.
e. In Main, erase the whole if block that compares the 2 last names and the output of which was greater. Keep the 2 student objects.
f. At the top of Main, above the 2 Student object declarations, have the user input 2 string values and store them in appropriate string variables (perhaps string1 and string2).
g. Create an instance of the DuoArray object called strings. In the constructor, pass it the 2 string variables from the previous step.
h . Right after the declaration, call the sort method of the DuoArray strings object.
i. After the sort method, output the strings objects Value1 and Value2 properties (IN THAT ORDER) in a message like: The 2 strings in order are
Run the program. Your inputted values should output in alphabetical order. Try 2 strings that need to be swapped to be in order, and 2 strings that are already in order. Verify it works.
Since our DuoArray class should be able to store and sort any type of object, well store 2 students in a DuoArray object.
j. In Main, after the 2 Student objects you created, create a new DuoArray object called students, and pass it the 2 student objects in the DuoArray constructor.
We want the DuoArray to work with Students, as well as other types, in addition to strings.
You may have used a List object to store integers using a statement like: List
k . After the DuoArray class declaration, place
public class DuoArray
l. Replace, either manually or using Find...Replace, all occurrences of string with T inside the DuoArray class ONLY. Note: If a string inside this class will store something other than the Value1 and Value2 properties, then it would stay a string. Remember, this T parameter will often be something other than a string, so if an actual string is needed, keep it a string. In our example, no strings are used that store anything other than Value1 or Value2.
You now should have 3 errors:
Inside the DuoArray class, the CompareTo method errs with something like, T does not contain a definition for CompareTo
In the Main method, the 2 statements that instantiate the DuoArray class each err with something like, Using the generic type DuoArray
24. In Main, on the first DuoArray instantiation, change the code to read:
DuoArray
Note: use the names you used for your string variables in place of the arguments string1 and string2.
m . Also in Main, modify the second DuoArray instantiation so the type parameter is the Student class.
n . Ensure that this fixes both of the syntax errors in Main. If not, find your mistakes and fix. Note: the DuoArray will still have an error, so your program still doesnt run.
The error in DuoArray is that the class knows NOTHING about the class/type of T. It is possible that the class of T has a CompareTo method. It is also equally possible that the class of T does not have a CompareTo method. Therefore, if we are going to use CompareTo in this generic method, we must ensure that T has a CompareTo method.
Interfaces
Here is what the IComparable interface looks like:
public interface IComparable { int CompareTo(Object anotherObject) }
What does this have to do with our program? We basically have 2 things to accomplish in order to make our program work:
We must ensure that the generic type T references only classes that implement IComparable
We must implement the IComparable interface in our Student class, which will also require us to implement a CompareTo method in the class.
In the DuoArray class, on the class declaration line, add the bold code public class DuoArray
This removes the error in DuoArray, because the where statement requires that any type T will implement the IComparable interface. Therefore, we are 100% certain that any objects stored in the DuoArray class will have a CompareTo method.
As you probably expected, this created an error elsewhere in our program. Back in Main, our DuoArray instantiation errs with something like, Cant convert from Student to IComparable. In short, we must implement the IComparable interface in Student, and then it will BE an IComparable-type class (in addition to being a Student-type class).
4. In the Student class, on the class declaration line, add the bold code: public class Student:IComparable
This change fixes the previous error in Main, but creates an error in Student. The error now says something like, Student does not implement the interface member CompareTo
a. Add the CompareTo method to the Student class. Its signature must match the method signature in the Icomparable interface.
Code should look like this: public int CompareTo(object objectToCompare) { }
Lets compare first by LastName, then by FirstName. If we have 2 students with the last name of Jones, then the FirstName will have to be the tie-breaker.
Because the parameter is of Object type, we have to cast it to a Student so we can see the LastName and FirstName properties. HOWEVER, the class cant know that the objectToCompare is a Student. The official specification of the CompareTo method says that it should throw an ArgumentException if the objects arent of the same type. So, well create an if statement that either throws an exception OR casts the object to a Student. That way were following the specification.
b. Type this code in the CompareTo method: if( this.GetType() != objectToCompare.GetType() ) { throw new ArgumentException(Object must be of type Student); } else { Student anotherStudent = objectToCompare as Student; }.
c. In a separate if...else if...else block, code your comparison using this.LastName and anotherStudent.LastName. Remember to use the String objects CompareTo method, and look for ==0, >0, and <0. When both last names are the same, check the first names. All paths should return an integer.
d. add 2 lines to the Main method to sort and display your DuoArray:
e. Call the sort method of your students DuoArray object.
the contents of the Value1 and Value2 properties of the students object with a message like: The 2 students in order are
Run the program. The output says The 2 students in order are Student and Student. Remember that ToString method in the Object class from the beginning of this assignment? Since there is no ToString method in the Student class, well add it. Because a version already exists in Object, we must override the method. Its signature looks like this:
public string ToString()
5. In the Student class, add the following method: public override string ToString() { return ${this.LastName}, {this.FirstName}; }
Run the program. Finally, we have output. Try typing in names with different last names. Try hard-coding the student data. Try the same last names, but different first names, as well as other combinations.
To prove our DuoArray actually works with other .NET types, in Main, instantiate a DuoArray called integers with 2 hard-coded integer numbers.
Call integers.sort()
Display the integers objects properties Value1 and Value2 as before with the strings and Students.
Try different integers, and see if they display in order.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
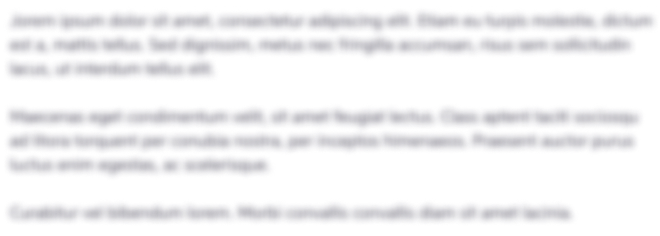
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started