Question
/** * * void addFront( double ) A method to add a new element at the front of the sequence and make it the current
/** * * void addFront( double ) A method to add a new element at the front of the sequence and make it the current element. */ public void addFront(double){ //write code } /** * A method to remove the element at the front of the sequence. If there is only one element, remove it and make the current element null, otherwise make the current element the new front element. Throw an IllegalStateException if the sequence is empty when the method is called. */ public void removeFront(){ //write code } /** * * * void setCurrent ( int n ) * A method that makes the nth element become the current element. * Note: This n value is not an index value. If n equals one, then the current element is at location zero. * Throw an IllegalStateException if the sequence is empty. * Throw an IllegalArgumentException if n does not represent a valid location in the array. */ public void setCurrent ( int n ){ //write code } /** * * double getElement ( int n ) A method that returns the nth element of the sequence. Make current element this nth element. NOTE: n is not the index value. (if the value of n that is passed in is 4, that means index 3 in the array) Throw an IllegalStateException if the sequence is empty. Throw an IllegalArgumentException if n is greater than the sequence size, or if n is zero or negative. */ public double getElement ( int n ){ //write code } /** * * boolean equals ( Object ) * Method that returns true if sequence is the same length and order and data. (The current element could be different) * Use the proper format for the equals method shown in the book, we are overriding the equals method from the Object class. */ public boolean equals (Object){ //write code } /** * * String toString ( ) * creates a string of all elements in order separated by a space. * Use start( ), advance( ) and getCurrent( ) to progress through data * Throw an IllegalStateException if the sequence is empty. * IMPORTANT: The methods above will change the value of the current element, but the current element should be changed by this method. Make sure to reset to its original value by the end of this method. * Remember : toString does not output to screen, it returns a string */ public String toString( ){ //write code }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
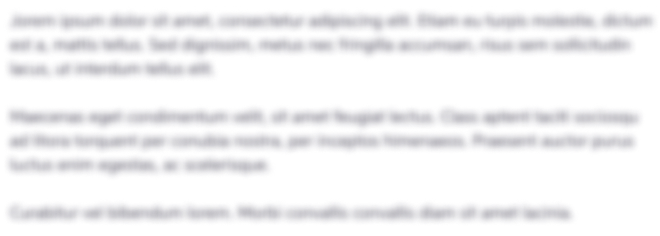
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started