Question
Well I am trying to code my arrays into methods. But im getting an error in my output. getTotal . This method should accept a
Well I am trying to code my arrays into methods. But im getting an error in my output.
- getTotal. This method should accept a one-dimensional array (here it ismyArray) as it's argument and return the total of the values in the array.
- getAverage. This method should accept a one-dimensional array (here it ismyArray) as it's argument and return the average of the values in the array.
- getHighest. This method should accept a one-dimensional array (here it ismyArray) as it's argument and return the highest value in the array.
- getLowest. This method should accept a one-dimensional array (here it ismyArray) as it's argument and return the lowest value in the array.
My code:
public class ArrayOperations2
{
public static int getTotal( int [] myArray ){
int total = 0;
for( int currentNumbersIndex = 0; currentNumbersIndex
total+=myArray[currentNumbersIndex];
}
return total;
}
public static int getAverage( int [] myArray ){
int numbersTotal = getTotal( myArray );
int numberOfItemsInNumbersArray = myArray.length;
int average = numbersTotal / numberOfItemsInNumbersArray;
return average;
}
public static int getHighest( int [] myArray ){
int highestNumber = myArray[0];
for( int currentNumbersIndex = 0; currentNumbersIndex
if( myArray[ currentNumbersIndex ] >highestNumber ){
highestNumber = myArray[ currentNumbersIndex ];
}
}
return highestNumber;
}
public static int getLowest( int [] myArray ){
int lowestNumber = myArray[0];
for (int currentNumbersIndex = 0; currentNumbersIndex
if( myArray[ currentNumbersIndex ]
lowestNumber = myArray[ currentNumbersIndex ];
}
}
return lowestNumber;
}
public static void main(String[] args)
{
int[] myArray = {45, 38, 27, 46, 81, 72, 56, 61, 20, 48, 76, 91, 57, 35, 78};
System.out.println("Total: "+ getTotal( myArray )+" "+
"Average: "+ String.format("%.2f", getAverage( myArray ))+" "+
"Highest: "+ getHighest( myArray )+" "+
"Lowest: "+ getLowest( myArray ));
}
}
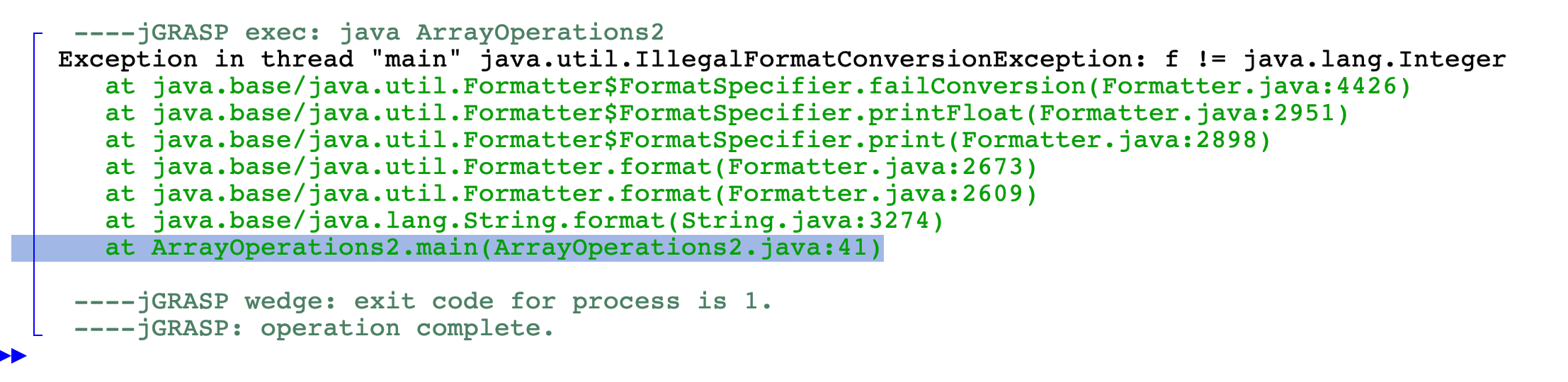
Step by Step Solution
There are 3 Steps involved in it
Step: 1
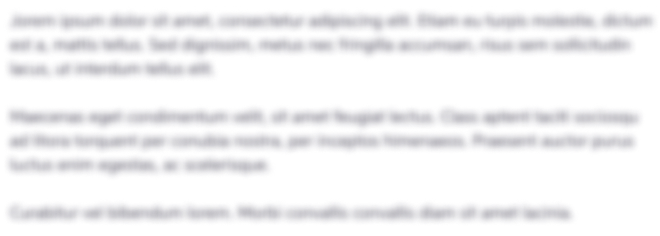
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started