Question
What I have so far from the previous project: ********************************************************************************* Song.java ********************************************************************************* public class Song implements Comparable { private String title; private String artist; private
What I have so far from the previous project: ********************************************************************************* Song.java *********************************************************************************
public class Song implements Comparable
public Song() { setTitle(""); setArtist(""); setAlbum(""); }
public Song(String title, String artist, String album) { setTitle(title); setArtist(artist); setAlbum(album); }
//Gets the song title.
public String getTitle() { return title; }
// Set and trim the song title.
public void setTitle(String ti) { try{ title = ti.trim(); } catch(NullPointerException nullArg) { title = ""; } }
// Gets the song artist.
public String getArtist() { return artist; }
//Set and trim the song artist.
public void setArtist(String ar) { try{ artist = ar.trim(); } catch(NullPointerException nullArg) { artist = ""; } }
//Gets the song album.
public String getAlbum() { return album; }
// Sets and trims the song album.
public void setAlbum(String al) { try{ album = al.trim(); } catch(NullPointerException nullArg) { album = ""; } }
// Checks if this song's title, artist, and album match that of the argument song.
@Override public boolean equals(Object arg) { if(arg == null) { return false; } else if(this == arg) { return true; } else if(!(arg instanceof Song)) { return false; } Song other = (Song) arg;
if(this.getTitle().equalsIgnoreCase(other.getTitle()) && this.getAlbum().equalsIgnoreCase(other.getAlbum()) && this.getArtist().equalsIgnoreCase(other.getArtist())) { return true; } return false; }
/// Converts the song to a string by combining the title, artist, and album.
@Override public String toString() { return "Title: " + getTitle() + " Artist: " + getArtist() + " Album: " + getAlbum() + " "; }
// Compares this song's album and title lexicographically to those of the argument.
@Override public int compareTo(Song arg) throws NullPointerException { if(arg == null) { throw new NullPointerException(); }
int albumCompResult = this.getAlbum().compareToIgnoreCase(arg.getAlbum());
if(albumCompResult != 0){ return albumCompResult; } else { // albums are equal int titleCompResult = this.getTitle().compareToIgnoreCase(arg.getTitle());
if (titleCompResult != 0) { return titleCompResult; } else { // titles are equal return this.getArtist().compareToIgnoreCase(arg.getArtist()); } } } }
********************************************************************************* SongReader.java *********************************************************************************
import java.util.Scanner; import java.io.File; import java.util.EmptyStackException; import java.util.NoSuchElementException; import java.io.FileNotFoundException; import java.lang.IllegalArgumentException;
public class SongReader {
private static LinkedStack
public static void main(String[] args) { printHeading();
try{ Scanner fileReader = openFile();
String nextLine = fileReader.nextLine().trim(); String previousLine = "";
while(fileReader.hasNextLine()) { if (previousLine.startsWith("") && !(previousLine.startsWith("")) ) { try { addToTagStack(previousLine, nextLine); } catch(IllegalArgumentException nullArgument) { while(!previousLine.equalsIgnoreCase("")) { // Iterates until end of current song previousLine = fileReader.nextLine().trim(); // Moves the scanner down the file } // end of while } // end of catch catch(NullPointerException e) { } } // end of if
if(previousLine.startsWith("") && previousLine.endsWith(">")) { // Checks if previous line was a closing tag closeTag(previousLine); }
previousLine = nextLine; // Saves the value of the current line so it can be used in future iterations try{ nextLine = fileReader.nextLine().trim(); } catch(NoSuchElementException e){ } } // end of while loop } // end of try catch(FileNotFoundException noFile){ System.out.println("There was an error opening or reading from the file."); } }
//Adds the parameter to the stack of tags if it denotes a title, artist, or album, private static void addToTagStack(String previousLine, String nextLine) throws IllegalArgumentException { if(previousLine.equalsIgnoreCase("
if(nextLine.equals("") || nextLine.equalsIgnoreCase("
if(nextLine.equals("") || nextLine.equalsIgnoreCase("
if(nextLine.equals("") || nextLine.equalsIgnoreCase("
//Removes the previous tag from the top of the stack if it matches the song, title, artist, or album tag parameter.
private static void closeTag(String previousLine) { if(previousLine.equalsIgnoreCase("")) { endSong(); } try { if(previousLine.equalsIgnoreCase("")) { if (tagStack.peek().equalsIgnoreCase("
//Prints the current song object and resets it for any future songs.
private static void endSong() { try { System.out.println(currentSong.toString());
if (!tagStack.pop().equalsIgnoreCase("
assert (tagStack.isEmpty());
Song blankSong = new Song(); //Resets song data between songs currentSong = blankSong; }
// Prompts the user for a filepath, and scans the console for a response.
private static String promptForFilePath() { System.out.println("Enter the file path: "); Scanner input = new Scanner(System.in); String f = input.nextLine();
input.close(); return f; }
//Verify that the file exists, then creates a scanner to read it.
private static Scanner openFile() throws FileNotFoundException { File file = new File(""); file = new File(promptForFilePath());
return new Scanner(file); }
// Prints a heading about the program to the console.
private static void printHeading() { System.out.println("Song Reader"); }
}
********************************************************************************* LinkedStack *********************************************************************************
import java.util.EmptyStackException;
public final class LinkedStack
public LinkedStack() { topNode = null; } // end default constructor
public void push(T newEntry) { topNode = new Node(newEntry, topNode); // Node newNode = new Node(newEntry, topNode); // topNode = newNode; } // end push
public T peek() { if (isEmpty()) throw new EmptyStackException(); else return topNode.getData(); } // end peek
public T pop() { T top = peek(); // Might throw EmptyStackException assert (topNode != null); topNode = topNode.getNextNode();
return top; } // end pop
public boolean isEmpty() { return topNode == null; } // end isEmpty
public void clear() { topNode = null; // Causes deallocation of nodes in the chain } // end clear
private class Node { private T data; // Entry in stack private Node next; // Link to next node
private Node(T dataPortion) { this(dataPortion, null); } // end constructor
private Node(T dataPortion, Node linkPortion) { data = dataPortion; next = linkPortion; } // end constructor
private T getData() { return data; } // end getData
// private void setData(T newData) // { // data = newData; // } // end setData
private Node getNextNode() { return next; } // end getNextNode
// private void setNextNode(Node nextNode) // { // next = nextNode; // } // end setNextNode } // end Node } // end LinkedStack
****************************************************************** Stack Interface ******************************************************************
public interface StackInterface
public void push(T newEntry);
//Removes and returns this stack's top entry.
public T pop();
// Retrieves this stack's top entry.
public T peek();
// Detects whether this stack is empty.
public boolean isEmpty();
// Removes all entries from this stack. public void clear(); } // end StackInterface
Programming Assignment 5 Note: When you turn in an assignment to be graded in this class you are making the claim that you neither gave nor received assistance on the work you turned in (except, of course, assistance from the instructor) Programming Project 5 builds on the work you completed in Programming Project 4. Begin by writing a subclass of your Song class and call it MySong. The MySong class includes an additional data field, playcount. If the playcount is not present in the input data file, then assign a value of 0 to the instance variable Refactor the songReader class to read Mysong data from the input file. Write the class MusicManager that contains the main method and instantiates a SongReader object and call the methods of the SongReader class to read in the input data file The MusicManager class will provide a variety of functions based on input to the program from command line arguments. The arguments have a very specific ordering. The first argument is the name of the file that contains all of the song data. The format of this file is the same as described for Project 4 with an additional tag for the playcount,
Step by Step Solution
There are 3 Steps involved in it
Step: 1
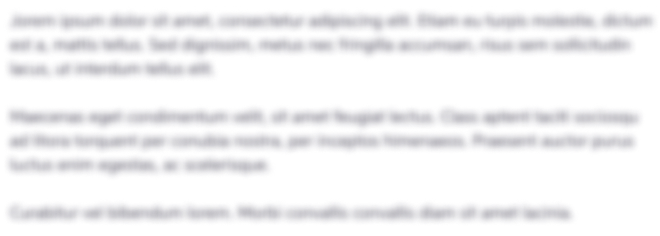
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started