Question
What I need done is using the code below, edit and change it to create the different type of program scheduling. The below code does
What I need done is using the code below, edit and change it to create the different type of program scheduling. The below code does the FCFS version. And the other versions of scheduling need to be created. The current code below also only has up to 5 workers instead of 6-10. The project will consist of creating a simulation of an operating system scheduler handling multiple threads or processes. The student will create a program that launches 6 - 10 worker threads simulating a processor bound, I/O bound and an intermediate of the two types. The program will schedule the tasks using the First-Come, First-Served (FCFS), Shortest Job First, and Round Robin scheduling algorithms. The Program should be written in Java and the Round Robin algorithm should employ the suspend() and resume() thread methods (yes I know the methods are depreciated. Included as an attachment is a sample). The student will take statistics and will write a paper describing what they found.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Below you will see an example that does somethign similar(This already does the FCFS method, now the First-come, shortest Job First, and Round Robin need to be created), it needs more working threads added(currently only does 5) plus needs to make sure all algorithms can be used so data can be collected.
________________________________________________________________________________________________________________________________________________________ CODE WRITTEN ALREADY(Does FCFS):
1.Wait time is
Time to get CPU (Time between , time when Thread.start is called and
time when "run" method is started (means thread get CPU)
Time for IO wait
Only applicable for IO thread
Time waited for System.out.printl to complete (not accurate)
2.We can't calculate exact wait time
3. I created following variable in each type of Thread class
long startTime,stopTime,runningTime , waitTime , startExecutionTime; startTime When called Thread.Start startExecutionTime when run method started execution (get the CPU) stopTime is when run() method completed waitTime is Time Difference between startTime,startExecutionTime + wait for IO
4. Please check comments in Code
5. startIOThreads ,startComputationThreads methods are used to start certain type of threads
We can re arrange calling these methods to start required threds first
for example if we want start IOThreads first the
startIOThreads()// First statement
startComputationThreads() //next
you can comment the requied method if you don't want to start any kind of threads
comment calling of startIOThreads()if you don't want to start IO threads
Code
ThreadIO.java :-
-------------------------
/** * * @author This thread perform the IO operation * */ public class ThreadIO extends Thread { //Variable for Start and Stop time... /** * startTime When called Thread.Start * startExecutionTime when run method started execution (get the CPU) * stopTime is when run() method completed * waitTime is Time Difference between startTime,startExecutionTime + wait for IO */ long startTime,stopTime,runningTime , waitTime , startExecutionTime; @Override public synchronized void start() { // set Start time to current System time startTime = System.currentTimeMillis(); // TODO Auto-generated method stub super.start(); } @Override // define the body which need to be executed by the thread public void run() { // set Start Execution time to current System time startExecutionTime = System.currentTimeMillis(); for (int i = 0; i < 1000; i++){ //Calculate approximate wait start time for IO , Exact time can't be calculated long longStartIowait = System.currentTimeMillis(); System.out.println(getName() + " currently running"); //Calculate approximate wait stop time for IO , Exact time can't be calculated long longStopIowait = System.currentTimeMillis(); //Add to Wait Time waitTime += (longStopIowait - longStartIowait); } // set Stop time to current System time stopTime = System.currentTimeMillis(); //Calculate wait time waitTime += startExecutionTime - startTime; //Calculate Running time runningTime = stopTime - startTime; //Print Wait Time //System.out.println("Wait Time of Thread "+getName() + "in MilliSecs is " + waitTime); //Print Running Time //System.out.println("Running Time of Thread "+getName() + "in MilliSecs is " + runningTime); }
}
ComputationThread.java
------------------------------------------
public class ComputationThread extends Thread {
int a = 3; int b = 6; //Variable for Start and Stop time... /** * startTime When called Thread.Start * startExecutionTime when run method started execution (get the CPU) * stopTime is when run() method completed * waitTime is Time Difference between startTime,startExecutionTime */ long startTime,stopTime,runningTime , waitTime , startExecutionTime; @Override public synchronized void start() { // set Start time to current System time startTime = System.currentTimeMillis(); // TODO Auto-generated method stub super.start(); }
@Override // define the body which need to be executed by the thread public void run() { // set Start Execution time to current System time startExecutionTime = System.currentTimeMillis(); //Computation Thread should not do any I/O like System.out.println so remove for (int i = 0; i < 1000; i++){ //We do some Square root here to consume CPU Math.sqrt(i); } // set Stop time to current System time stopTime = System.currentTimeMillis(); //Calculate wait time waitTime = startExecutionTime - startTime; //Calculate Running time runningTime = stopTime - startTime; //Print Wait Time //System.out.println("Wait Time of Thread "+getName() + "in MilliSecs is " + waitTime); //Print Running Time //System.out.println("Running Time of Thread "+getName() + "in MilliSecs is " + runningTime); } }
ControllerThread.java
------------------------------------------
/** * * @author Controls all the threads * */ public class ControllerThread {
// number of objects of ThreadIo and computationThread class to be created static final int NUMBER_OF_THREADS = 5; // create an array of 5 objects of each ThreadIo and computationThread // class ThreadIO IOobjects[] = new ThreadIO[NUMBER_OF_THREADS]; ComputationThread computationThread[] = new ComputationThread[NUMBER_OF_THREADS]; //Method to initialize the threads public void initilaizeThread(){ for (int i = 0; i < NUMBER_OF_THREADS; i++) { // initializing the objects IOobjects[i] = new ThreadIO(); IOobjects[i].setName("IO Thread " + (i + 1)); computationThread[i] = new ComputationThread(); computationThread[i].setName("Computation Thread " + (i + 1));
} } //Method to start all IO threads public void startIOThreads(){ for (int j = 0; j < NUMBER_OF_THREADS; j++) { IOobjects[j].start(); // wait for the thread termination try { IOobjects[j].join(); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } //Method to start all Computation Threads public void startComputationThreads(){ for (int j = 0; j < NUMBER_OF_THREADS; j++) { computationThread[j].start(); // wait for the thread termination try { computationThread[j].join(); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } //Method to Print Results public void printResults(){ long totalExecutionTime = 0; long totalWaitTime = 0; System.out.println(); System.out.println(); System.out.println("******************************************************"); System.out.println("Computation time of IO Threads"); System.out.println("******************************************************"); for (int j = 0; j < NUMBER_OF_THREADS; j++) { if(IOobjects[j].startTime == 0){ continue; } totalExecutionTime += IOobjects[j].runningTime; totalWaitTime += IOobjects[j].waitTime; System.out.println("Wait Time of " + IOobjects[j].getName() + " :" + IOobjects[j].waitTime + " milliseconds"); System.out.println("Time taken by " + IOobjects[j].getName() + " to execute :" + IOobjects[j].runningTime + " milliseconds"); } System.out.println(); System.out.println(); System.out.println("******************************************************"); System.out.println("Computation time of Computational Threads"); System.out.println("******************************************************"); for (int j = 0; j < NUMBER_OF_THREADS; j++) { if(computationThread[j].startTime == 0){ continue; } totalExecutionTime += computationThread[j].runningTime; totalWaitTime += computationThread[j].waitTime; System.out.println("Wait Time of " + computationThread[j].getName() + " :" + computationThread[j].waitTime + " milliseconds"); System.out.println("Time taken by " + computationThread[j].getName() + " to execute :" + computationThread[j].runningTime + " milliseconds"); } System.out.println(); System.out.println(); System.out.println("******************************************************"); System.out.println("Total Execution Time is : "+totalExecutionTime); System.out.println("******************************************************"); System.out.println(); System.out.println(); System.out.println("******************************************************"); System.out.println("Total Wait Time is : "+totalWaitTime); System.out.println("******************************************************"); System.out.println(); System.out.println(); System.out.println("******************************************************"); System.out.println("Average Wait Time is : "+(totalWaitTime/NUMBER_OF_THREADS)); System.out.println("******************************************************"); System.out.println(); System.out.println(); } public static void main(String[] args) throws InterruptedException {
long scheduleStartTime = 0; long scheduleEndTime = 0; ControllerThread controller = new ControllerThread(); controller.initilaizeThread(); // record the start schedule Time scheduleStartTime = System.currentTimeMillis(); //Re Arrange of Execution of IO/Computation threads //If you don't want to start certain type of Thread then comment controller.startComputationThreads(); controller.startIOThreads();
// record the end time of the schedule scheduleEndTime = System.currentTimeMillis(); //Print Results controller.printResults(); System.out.println("******************************************************"); System.out.println("Computation time of Schedule all the Threads"); System.out.println("******************************************************"); System.out.println("Time Taken to schedule and run all the threads: "+(scheduleEndTime -scheduleStartTime)+ " milliseconds"); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
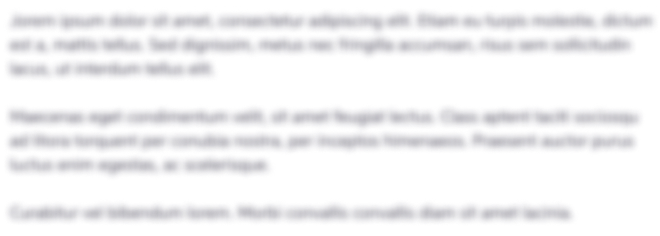
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started