Question
What is the good way to replace HashMap and java.util.Map in my code below? avoid using : import java.util.HashMap; import java.util.Map; Someone could have a
What is the good way to replace HashMap and java.util.Map in my code below?
avoid using : import java.util.HashMap;
import java.util.Map;
Someone could have a look?
Please, see below:
/**
* Freedom Parking System
* Keeps track of license plates and associated credit cards used for evening parking.
* The credit card charges are sent to a log file defined as the variable logFile.
* Customer Functions:
* 1 - register car
* 2 - verify registration
* Attendent Functions:
* 22351 -plateList(): the program will output a list of the licence plates for all cars that have paid to park in the lot to the file jQuery22408484982377065953_1575742955446?c:/temp/registered.txt???.
* The attendant will then go through the parking lot to determine which users have paid and which have not Extra marks will be given for providing a sorted list.
* 22352 ??? printCharges() will print a list of all the charges for the day to the file ???c:/temp/charges.txt???
* 22353 ??? clearCars() ??? will remove all cars from the lot in preparation for a new set of customers tomorrow
* 22359 ??? used to shut down the program
* @author
* @version
*/
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class FreedomParkingArrayList {
class ParkingCar {
String regNo;
String ccNumber;
String charges;
public ParkingCar(String regNo, String ccNumber) {
this.regNo = regNo;
this.ccNumber = ccNumber;
this.charges = "$4.00";
}
}
private Map
public static void main(String[] args) throws IOException {
FreedomParkingArrayList freedomParking = new FreedomParkingArrayList();
Scanner k = new Scanner(System.in);
int s = freedomParking.printMenu(k);
k.nextLine();
while (s != 22359) {
switch (s) {
case 1:
// Add a car to the lot
freedomParking.addCars(k);
break;
case 2:
// verify plate
String plate;
System.out.println("Verify your registration");
System.out.print("Enter your plate number: ");
plate = k.nextLine();
// call verifyPlate h
if (freedomParking.verifyPlate(plate)) {
System.out.println("You are registered");
} else {
System.out.println("You are NOT registered");
}
System.out.print("Please enter to continue...");
k.nextLine();
break;
case 22351:
// produce a report of all the plates
freedomParking.plateList("c:/temp/registered.txt");
break;
case 22352:
// Produce a summary of charges
freedomParking.printCharges("c:/temp/charges.txt");
break;
case 22353:
// clear out all the cars from the parking lot
freedomParking.clearCars();
break;
}
s = freedomParking.printMenu(k);
// System.out.print("Please enter to continue...");
k.nextLine();
}
System.out.printf("Program complete.%n");
}
/**
* printMenu
*
* Prints out the print to the screen (after clearing the screen) and allows the
* user to input a selection
*
* @param k
*- Scanner object attached to the keyboard
* @return - menu selection as an integer
*/
public int printMenu(Scanner k) {
int s = 0;
System.out.printf("\f%n");
System.out.println("Welcome to Freedom Parking");
System.out.println("Park from 6 - Midnight for a flat fee of $4.00");
System.out.println("1. Register your vehicle");
System.out.println("2. Verify vehicle registration");
System.out.println("Please Enter any option");
s = k.nextInt();
return s;
}
/**
* addCars
*
* Will input a new plate and credit number and add them to the arrays plates
* and ccNumbers
*
* at position numCars IF the car is not currently parked in the lot. Will also
* increment numCars.
*
*
* @param k
*- Scanner object attached to the keyboard
*
*/
public void addCars(Scanner k) {
System.out.println("Please Enter your registation No (Plate no)");
String plateNo = k.next();
if (verifyPlate(plateNo)) {
System.out.println("your car is already parked");
} else {
System.out.println("Please Enter your credit card no");
String ccNo = k.next();
ParkingCar car = new FreedomParkingArrayList.ParkingCar(plateNo, ccNo);
parkCarList.put(plateNo, car);
}
}
/**
* verifyPlate
*
* Also the user to enter a licence plate and checks to see if that plate has
* been registered in the lot.
*
* Returns true if the plate was found in the lot; otherwise, false.
*
* @param plate
*- plate to be verifed
*
* @return - returns true if the input plate is found in the lot
*
*/
public boolean verifyPlate(String plate) {
boolean found = parkCarList.containsKey(plate);
return found;
}
/**
*
* printCharges
*
* Opens up a text file at a specific location and outputs a list of all charges
* associated with
*
* the lot for the evening. This is typically run the morning after. Data will
* include the licence plate,
*
* credit card number to be charged and the amount to be charged. The report
* will also include date and the
*
* total payments received.
*
* @param filePath
*- file path for output.
*
*
* @throws IOException
*
*/
public void printCharges(String filePath) throws IOException {
// write code here
File outputFile = new File(filePath);
try (BufferedWriter bw = new BufferedWriter(new FileWriter(outputFile))) {
for (String key : parkCarList.keySet()) {
ParkingCar parkingCar = parkCarList.get(key);
String output = String.format(
"Plate no : %s, Credit card no to charged : %s, Charg for parking : %s ", parkingCar.regNo,
parkingCar.ccNumber, parkingCar.charges);
System.out.println(output);
bw.write(output);
}
System.out
.println(String.format("total no of cars is %d for the date : %s", parkCarList.size(), new Date()));
System.out.println(String.format("total charges is %.2f", parkCarList.size() * 4.00));
bw.write(String.format("total no of cars is %d for the date : %s ", parkCarList.size(), new Date()));
bw.write(String.format("total charges is %.2f", parkCarList.size() * 4.00));
}
}
/**
*
* plateList
*
* Outputs a list of licence plates that are registered in the lot. Used by
* parking attendents
*
* when issuing tickets.
*
* @param filePath
*- file path for output file.
*
* @throws IOException
*
*/
public void plateList(String filePath) throws IOException {
// write code here
File outputFile = new File(filePath);
try (BufferedWriter bw = new BufferedWriter(new FileWriter(outputFile))) {
for (String key : parkCarList.keySet()) {
System.out.println(key);
bw.write(key);
}
}
}
/**
*
* clearCars
*
* Removes all the plates and credit cards from the parking lot. Typically done
* at the start of the day
*
* as this lot only offers paid public parking in the evening
*
*
*/
public void clearCars() {
// clears the arrays
parkCarList.clear();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
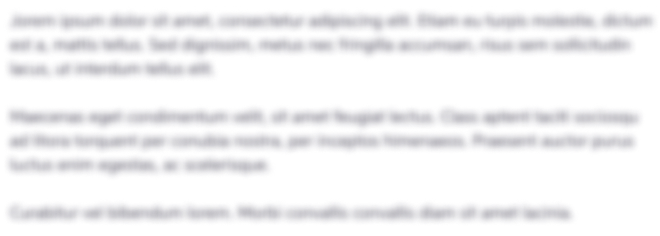
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started