Question
When I run the code, it works, BUT, when I shut down the app all data get erased, it is not in memory. Can you
When I run the code, it works, BUT, when I shut down the app all data get erased, it is not in memory. Can you help my to see a problem? I would like to save data for next run, because it's like a contact so users already know who they added.
File 1: PhoneBook.java
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Scanner;
public class PhoneBook {
static Scanner scnr = new Scanner(System.in);
// Declare a variable "contactInformation" and initializes it to an empty ArrayList
static List
public static void main(String[] args) {
System.out.println("Hello! Welcome to Phone Book.");
//Display the Phone Book menu
String choice;
do {
System.out.println(); //Skip a line
System.out.println("Choose an option: ");
System.out.println("1. Add contact");
System.out.println("2. Delete contact");
System.out.println("3. Search contacts");
System.out.println("4. List contacts");
System.out.println("5. Fix contact.");
System.out.println("6. Exit");
System.out.print("Enter your choice: ");
choice = scnr.nextLine();
//a switch statement based on the user's inpu
switch (choice) {
case "1":
addContact(); //calls addContact method
break;
case "2":
deleteContactByName(); //calls deleteContact method
break;
case "3":
searchContact(); // calls searchContact method
break;
case "4":
listContact(); //calls listContact method
break;
case "5":
fixContact(); //calls fixContact method
break;
case "6":
System.out.println("Exiting...");
break;
default:
System.out.println("Invalid choice. Try again."); //no choice exist
break;
}
} while (!choice.equals("6"));
}
// Add contact's information into the PhoneBook
public static void addContact() {
System.out.print("Enter contact's first name: "); //Prompt the user to enter contact's first name.
String firstName = scnr.nextLine();
System.out.print("Enter contact's last name: "); //Prompt the user to enter contact's last name.
String lastName = scnr.nextLine();
String email; //Declare email
while (true) {
System.out.print("Enter email (must contain @): "); //Prompt the user to enter email.
email = scnr.nextLine();
if (email.contains("@")) { //checks if the user enter email contains @
break;
}
else {
System.out.println("Invalid email address. Please enter an email address that contains '@'.");
}
}
String phoneNumber;
while (true) {
//Prompt the user to enter email.
System.out.print("Enter phone number (must follow format: xxx-xxx-xxxx): ");
phoneNumber = scnr.nextLine();
if (phoneNumber.matches("\\d{3}-\\d{3}-\\d{4}")) { // check if the phone number matches the format
break;
}
else {
System.out.println("Invalid phone number format. Please enter a phone number in the format of xxx-xxx-xxxx.");
}
}
ContactInformation newContact = new ContactInformation(firstName, lastName, email, phoneNumber);
contactInformation.add(newContact);
System.out.println(firstName + " " + lastName + "'s information has been added."); //Output the contact's first and last name that the user has been added.
}
// Delete contact's information from ContactInformation
public static void deleteContactByName() {
if (contactInformation.isEmpty()) {
System.out.println("No contacts to delete.");
return;
}
System.out.print("Enter the first name of the contact to delete: ");
String firstName = scnr.nextLine();
System.out.print("Enter the last name of the contact to delete: ");
String lastName = scnr.nextLine();
Iterator
boolean foundContact = false;
while (iter.hasNext()) {
ContactInformation contact = iter.next();
if (contact.getFirstName().equalsIgnoreCase(firstName) &&
contact.getLastName().equalsIgnoreCase(lastName)) {
//Display contact's information
System.out.println("Name: " + contact.getFirstName() + " " + contact.getLastName() +
", Phone Number: " + contact.getPhoneNumber() +
", Email: " + contact.getEmail());
System.out.print("Do you want to delete this contact?(Yes/No): ");
String answer = scnr.nextLine();
if(answer.equalsIgnoreCase("Yes") || answer.equalsIgnoreCase("y")){
//Delete the contact
iter.remove();
System.out.println(contact.getFirstName() + " " + contact.getLastName() + " has been removed from the PhoneBook.");
}
else if(answer.equalsIgnoreCase("No") || answer.equalsIgnoreCase("n")){
System.out.println("Contact not deleted.");
}
else{
System.out.println("Invalid input. Contact not deleted.");
}
foundContact = true;
}
}
if (!foundContact) {
System.out.println("No contact found with the name " + firstName + " " + lastName);
}
}
// Search an entry
public static void searchContact() {
System.out.print("Enter search term: ");
String searchTerm = scnr.nextLine();
List
for (ContactInformation contact : contactInformation) {
if (contact.getFirstName().toLowerCase().contains(searchTerm.toLowerCase()) ||
contact.getLastName().toLowerCase().contains(searchTerm.toLowerCase()) ||
contact.getEmail().toLowerCase().contains(searchTerm.toLowerCase()) ||
contact.getPhoneNumber().toLowerCase().contains(searchTerm.toLowerCase())) {
matchingContacts.add(contact);
}
}
if (matchingContacts.size() == 0) {
System.out.println("No matching contacts found");
}
else {
System.out.println("Matching contacts: ");
for (ContactInformation contact : matchingContacts) {
System.out.println(contact);
}
}
}
// List contacts informations currently in PhoneBook.
public static void listContact() {
if (contactInformation.isEmpty()) {
System.out.println("Phone book is empty.");
}
else {
for (ContactInformation contact : contactInformation) {
System.out.println(contact);
}
}
}
public static void fixContact() {
System.out.print("Enter contact's first name: ");
String firstName = scnr.nextLine();
System.out.print("Enter contact's last name: ");
String lastName = scnr.nextLine();
Iterator
while (iter.hasNext()) {
ContactInformation contact = iter.next();
if (contact.getFirstName().equals(firstName) && contact.getLastName().equals(lastName)) {
System.out.println("Enter field to fix:");
System.out.println("1. First name");
System.out.println("2. Last name");
System.out.println("3. Phone number");
System.out.println("4. Email");
System.out.print("Enter your choice: ");
String choice = scnr.nextLine();
switch (choice) {
case "1":
System.out.print("Enter new first name: ");
String newFirstName = scnr.nextLine();
contact.firstName = newFirstName;
System.out.println("First name updated.");
break;
case "2":
System.out.print("Enter new last name: ");
String newLastName = scnr.nextLine();
contact.lastName = newLastName;
System.out.println("Last name updated.");
break;
case "3":
String newPhoneNumber;
while (true) {
System.out.print("Enter new phone number (must follow format: xxx-xxx-xxxx): ");
newPhoneNumber = scnr.nextLine();
if (newPhoneNumber.matches("\\d{3}-\\d{3}-\\d{4}")) { // check if the phone number matches the format
break;
}
else {
System.out.println("Invalid phone number format. Please enter a phone number in the format of xxx-xxx-xxxx.");
}
}
contact.phoneNumber = newPhoneNumber;
System.out.println("Phone number updated.");
break;
case "4":
String newEmail; //Declare newEmail
while (true) {
System.out.print("Enter new email (must contain @): "); //Prompt the user to enter new email.
newEmail = scnr.nextLine();
if (newEmail.contains("@")) { //checks if the user enter email contains @
break;
}
else {
System.out.println("Invalid email address. Please enter an email address that contains '@'.");
}
}
contact.email = newEmail;
System.out.println("Email updated.");
break;
default:
System.out.println("Invalid choice. Try again.");
break;
}
return;
}
}
System.out.println("Contact not found.");
}
}
File 2: ContactInformation.java
import java.io.FileOutputStream;
import java.io.ObjectOutputStream;
import java.io.FileInputStream;
import java.io.ObjectInputStream;
public class ContactInformation {
public String firstName;
public String lastName;
public String email;
public String phoneNumber;
public ContactInformation(String firstName, String lastName, String email, String phoneNumber) {
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.phoneNumber = phoneNumber;
}
//getter methods
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getEmail() {
return email;
}
public String getPhoneNumber() {
return phoneNumber;
}
@Override
public String toString() {
return "Name: " + firstName + " " + lastName + ", Phone number: " + phoneNumber + ", Email: " + email;
}
public static void saveData() {
try{
FileWriter fileWriter = new FileWriter("phonebook.txt");
PrintWriter printWriter = new PrintWriter(fileWriter);
for (ContactInformation contact : contactInformation) {
printWriter.println(contact.getFirstName() + "," + contact.getLastName() + "," +
contact.getEmail() + "," + contact.getPhoneNumber());
}
printWriter.close();
fileWriter.close();
System.out.println("Data saved successfully.");
}
catch (IOException e){
System.out.println("An error occurred while saving the data.");
e.printStackTrace();
}
}
public static void LoadData () {
try {
File file = new File("phonebook.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] fields = line.split(",");
String firstName = fields[0];
String lastName = fields[1];
String email = fields[2];
String phoneNumber = fields[3];
ContactInformation contact = new ContactInformation(firstName, lastName, email, phoneNumber);
contactInformation.add(contact);
}
scanner.close();
System.out.println("Data loaded successfully.");
}
catch (FileNotFoundException e) {
System.out.println("The file was not found.");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
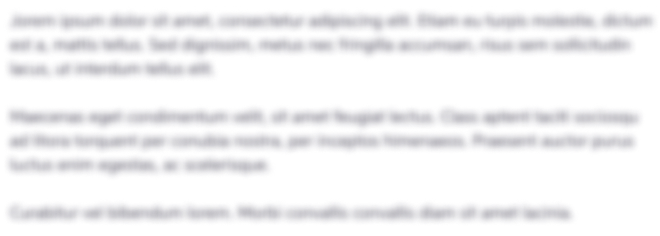
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started