Question
While calculating LCOM4, we ignore constructors and destructors. Constructors and destructors frequently set and clear all variables in the class, making all methods connected through
While calculating LCOM4, we ignore constructors and destructors. Constructors and destructors frequently set and clear all variables in the class, making all methods connected through these variables, which increases cohesion artificially.
LCOM4 = 1 indicates a cohesive class, which is the "good" class.
LCOM4 >= 2 indicates a problem. The class should be split into so many smaller classes.
LCOM4 = 0 happens when there are no methods in a class. This is also a "bad" class.
Calculate the value of the LCOM4 measurement for the following code:
#include "src/route.h"
Route::Route(std::string name, Stop ** stops, double * distances,
int num_stops, PassengerGenerator * generator) {
//Constructors ignored in LCOM4 calculation
}
void Route::Update() {
GenerateNewPassengers();
for (std::list
it != stops_.end(); it++) {
(*it)->Update();
}
}
bool Route::IsAtEnd() const {
return destination_stop_index_ >= num_stops_;
}
void Route::NextStop() {
destination_stop_index_++;
if (destination_stop_index_ < num_stops_) {
std::list
std::advance(iter, destination_stop_index_);
destination_stop_ = *iter;
} else {
destination_stop_ = (*stops_.end());
}
}
Stop * Route::GetDestinationStop() const {
return destination_stop_;
}
double Route::GetTotalRouteDistance() const {
int total_distance = 0;
for (std::list
iter != distances_between_.end();
iter++) {
total_distance += *iter;
}
return total_distance;
}
double Route::GetNextStopDistance() const {
std::list
std::advance(iter, destination_stop_index_-1);
return *iter; // resolving the iterator gets you the Stop * from the list
}
int Route::GenerateNewPassengers() {
// returning number of passengers added by generator
return generator_->GeneratePassengers();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
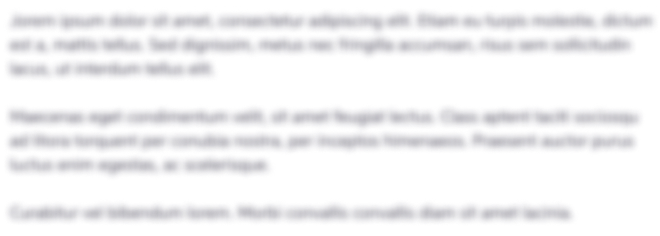
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started