Question
why do I have this error in my Junit Test file? its in my Junit test file GpsTest.java . I have files Gps.java and GpsTest.java
why do I have this error in my Junit Test file? its in my Junit test file GpsTest.java . I have files Gps.java and GpsTest.java to work with it. What would be the code fix for it? not sure what i am missing. I have that error on every line similar in the GpsTest.java which is the Junit test. The Junit test file gpsTest.java cannot be changed because i am supposed to make gps.java to work with gpsTest.java file. The error says the constructor Gps(GpsPosition) is not defined.
package gps;
import java.util.ArrayList; import java.util.Random;
public class Gps { private ArrayList route; public void gps(GpsPosition route) { this.route = new ArrayList(); this.route.add(route); }
/** * @return the route * - returns the array of GpsPositions */ public ArrayList getRoute() { return route; } public void update(GpsPosition position) { route.add(position); } public void randomUpdate() { Random rand = new Random(); GpsPosition randomPosition = new GpsPosition( route.get(route.size()-1).getLatitude() + rand.nextDouble()-.5, route.get(route.size()-1).getLongitude() + rand.nextDouble()-.5, route.get(route.size()-1).getElevation()); route.add(randomPosition); } /** * METHOD position - gets the current position from the gps array */ public GpsPosition position(){ return route.get(route.size()-1); } /** * METHOD distanceTraveled - Calculates the distance traveled as the sum of the distances between each point in the array */ public double distanceTraveled(){ double calculatedDistance = 0; for (int i = 1; i
private double distance(GpsPosition from, GpsPosition to){ final int R = 6371; // radius of the earth double longDist = Math.toRadians(to.getLongitude() - from.getLongitude()); double latDist = Math.toRadians(to.getLatitude() - from.getLatitude()); double a = Math.pow(Math.sin(latDist / 2), 2) + Math.cos(Math.toRadians(from.getLatitude())) * Math.cos(Math.toRadians(to.getLatitude())) * Math.pow(Math.sin(longDist /2), 2); double distance = R * 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a)); // elevation is in meters so we need to divide by 1000 to convert to Kilometers double height = (from.getElevation() - to.getElevation()) / 1000; return Math.sqrt(Math.pow(distance, 2) + Math.pow(height, 2)); }
public void reset(){ GpsPosition position = route.get(route.size()-1); route.clear(); route.add(position); } }
package gps;
public class GpsPosition { private double latitude; private double longitude; private double elevation; /** * @param latitude * @param longitude * @param elevation */ public GpsPosition(double latitude, double longitude, double elevation) { super(); if (latitude 90 || longitude 180) { throw new IllegalArgumentException("Invalid Latitude or/and Longitude"); } else if (elevation
/** * @return the latitude */ public double getLatitude() { return latitude; }
/** * @return the longitude */ public double getLongitude() { //make static to test is this class's main return longitude; }
/** * @return the elevation */ public double getElevation() { return elevation; } @Override public String toString() { return String.format("%.6f, %.6f (%.1f)", latitude, longitude, elevation); } public static void main(String args[]) { //GpsPosition denver = new GpsPosition(39.7392541, -104.9847129, 1606.296); //System.out.println(GpsPosition.getLongitude()); //GpsPosition.toString(); } }
package gps;
import static org.junit.Assert.*;
import java.util.ArrayList;
import org.junit.Before; import org.junit.Test;
public class GpsTest { private final double deltaDistance = 0.1; // deviations up to 100m are acceptable private final GpsPosition slc = new GpsPosition(40.760671, -111.891122, 1299.8 ); private final GpsPosition moab = new GpsPosition(38.573645, -109.546389, 1227.1); private final GpsPosition denver = new GpsPosition(39.7392541, -104.9847129, 1606.296); private final GpsPosition sf = new GpsPosition(37.808715, -122.409821, 5); private Gps gps; @Before public void setUp() throws Exception { gps = new Gps(slc); }
@Test public void testGps() { Gps gpsSF = new Gps(sf); assertEquals(sf, gpsSF.position()); assertEquals(0d, gpsSF.distanceTraveled(), deltaDistance); }
@Test public void testGetRoute() { ArrayList expectedRoute = new ArrayList(); expectedRoute.add(slc); assertTrue(expectedRoute.equals(gps.getRoute())); }
@Test public void testUpdate() { gps.update(moab); assertEquals(moab, gps.position()); } @Test public void testUpdateMultipleTimes() { gps.update(moab); gps.update(denver); gps.update(sf); assertEquals(sf, gps.position()); }
@Test public void testRandomUpdate() { // update Salt Lake City 100 times and ensure that each time // the updated position is within the required range for (int i = 0; i = 40.2606711 && newPosition.getLongitude() = -112.391122 && newPosition.getElevation() == 1299.8 ); } }
@Test public void testPosition() { assertEquals(slc, gps.position()); }
@Test public void testPositionAfterUpdate() { gps.update(denver); gps.update(sf); assertEquals(sf, gps.position()); } @Test public void testDistanceTraveledNoTravel() { double expected = 0d; // no distance has been traveled yet assertEquals(expected, gps.distanceTraveled(), deltaDistance); } @Test public void testDistanceTraveledSlcToMoab() { gps.update(moab); double expected = 315.279; assertEquals(expected, gps.distanceTraveled(), deltaDistance); } @Test public void testDistanceTraveledMoabToDenver() { Gps gpsMoab = new Gps(moab); gpsMoab.update(denver); double expected = 414.0689; assertEquals(expected, gpsMoab.distanceTraveled(), deltaDistance); } @Test public void testDistanceTraveledSlcToDenver() { gps.update(moab); gps.update(denver); double expected = 729.348; assertEquals(expected, gps.distanceTraveled(), deltaDistance); } @Test public void testReset() { gps.reset(); ArrayList expectedRoute = new ArrayList(); expectedRoute.add(slc); assertTrue(expectedRoute.equals(gps.getRoute())); }
@Test public void testResetAfterUpdate() { gps.update(sf); gps.update(denver); gps.reset(); ArrayList expectedRoute = new ArrayList(); expectedRoute.add(denver); assertTrue(expectedRoute.equals(gps.getRoute())); } }
25 @Before 26 Public void setup() throws Exception f 27 28 29 30 @Test 1 public void testGps ) gps new Gps (sic); = 32 Gps gpsSF -new Gps (sf); assertEquals (sf, gpsSF.position)); assertEquals (0d, gpsSF.distanceTraveled(), deltaDistance); 34 25Step by Step Solution
There are 3 Steps involved in it
Step: 1
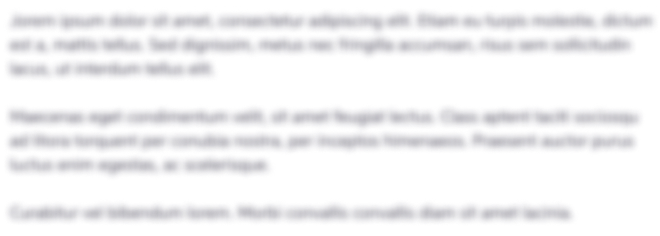
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started