Question
Why is my code not printing anything? The indicators.py file should be printing charts but not a single one is printing and no errors are
Why is my code not printing anything? The indicators.py file should be printing charts but not a single one is printing and no errors are popping up. I also need the testproject.py file to be able to run everything in the project and gain the same results. its almost like a shortcut instead of having to go and run every file individually. Please help with this.
indicators.py
import pandas as pd
import matplotlib.pyplot as plt
def bollinger_bands_signals(df, window=20):
rolling_mean = df['Close'].rolling(window=window).mean()
rolling_std = df['Close'].rolling(window=window).std()
upper_band = rolling_mean + (2 * rolling_std)
lower_band = rolling_mean - (2 * rolling_std)
# Calculate the Bollinger Bands indicator
bb_indicator = (df['Close'] - rolling_mean) / (2 * rolling_std)
return upper_band, lower_band, bb_indicator
def relative_strength_index_signals(df, window=14):
# Calculate RSI and add signals
delta = df['Close'].diff(1)
gain = delta.where(delta > 0, 0)
loss = -delta.where(delta < 0, 0)
avg_gain = gain.rolling(window=window).mean()
avg_loss = loss.rolling(window=window).mean()
rs = avg_gain / avg_loss
rsi = 100 - (100 / (1 + rs))
# Calculate RSI signals
df['RSI Buy'] = (rsi > 30).astype(int)
df['RSI Sell'] = (rsi < 70).astype(int)
def moving_average_convergence_divergence_signals(df, short_window=12, long_window=26):
# Calculate MACD and add signals
short_ema = df['Close'].ewm(span=short_window, adjust=False).mean()
long_ema = df['Close'].ewm(span=long_window, adjust=False).mean()
macd = short_ema - long_ema
signal = macd.ewm(span=9, adjust=False).mean()
df['MACD'] = macd
df['MACD Buy'] = (macd > signal).astype(int)
df['MACD Sell'] = (macd < signal).astype(int)
def stochastic_oscillator_signals(df, window=14):
# Calculate Stochastic Oscillator and add signals
high = df['High'].rolling(window=window).max()
low = df['Low'].rolling(window=window).min()
stochastic_osc = (df['Close'] - low) / (high - low) * 100
df['Stochastic Oscillator'] = stochastic_osc
df['Stochastic Buy'] = (stochastic_osc > 20).astype(int)
df['Stochastic Sell'] = (stochastic_osc < 80).astype(int)
def percentage_price_indicator_signals(df, window=20):
# Calculate Percentage Price Indicator and add signals
rolling_max = df['Close'].rolling(window=window).max()
rolling_min = df['Close'].rolling(window=window).min()
ppi = (df['Close'] - rolling_min) / (rolling_max - rolling_min)
df['Percentage Price Indicator'] = ppi
df['PPI Buy'] = (ppi > 0.8).astype(int)
df['PPI Sell'] = (ppi < 0.2).astype(int)
if __name__ == "__main":
# Load price data using util.py or from a CSV file
df = pd.read_csv('../data/JPM.csv', parse_dates=True, usecols=['Date', 'Open', 'High', 'Low', 'Close', 'Volume', 'Adj Close'])
# Calculate Bollinger Bands
upper_band, lower_band, bb_indicator = bollinger_bands_signals(df)
# chart for Bollinger Bands
plt.figure(figsize=(12, 4))
plt.plot(df['Date'], df['Close'], label='Close Price', color='blue')
plt.plot(df['Date'], upper_band, label='Upper Bollinger Band', color='green')
plt.plot(df['Date'], lower_band, label='Lower Bollinger Band', color='red')
plt.plot(df['Date'], bb_indicator, label='Bollinger Bands Indicator', color='purple')
plt.title('Bollinger Bands')
plt.legend()
plt.grid()
plt.show()
# Calculate RSI
relative_strength_index_signals(df)
# chart for RSI
plt.figure(figsize=(12, 4))
plt.plot(df['Date'], df['RSI'], label='RSI', color='blue')
plt.axhline(y=70, color='red', linestyle='--', label='Overbought (70)')
plt.axhline(y=30, color='green', linestyle='--', label='Oversold (30)')
plt.title('Relative Strength Index (RSI)')
plt.legend()
plt.grid()
plt.show()
# Calculate MACD
moving_average_convergence_divergence_signals(df)
# chart for MACD
plt.figure(figsize=(12, 4))
plt.plot(df['Date'], df['Close'], label='Close Price', color='blue')
plt.plot(df['Date'], df['MACD'], label='MACD', color='orange')
plt.plot(df['Date'], df['MACD Signal'], label='MACD Signal', color='red')
plt.bar(df['Date'], df['MACD Histogram'], label='MACD Histogram', color='purple', alpha=0.5)
plt.title('Moving Average Convergence Divergence (MACD)')
# Plot buy and sell signals based on MACD crossovers
plt.plot(df['Date'][df['MACD Buy'] == 1], df['Close'][df['MACD Buy'] == 1], 'g^', markersize=8, label='MACD Buy Signal')
plt.plot(df['Date'][df['MACD Sell'] == 1], df['Close'][df['MACD Sell'] == 1], 'rv', markersize=8, label='MACD Sell Signal')
plt.legend()
plt.grid()
plt.show()
# Calculate Stochastic Oscillator
stochastic_oscillator_signals(df)
#a chart for Stochastic Oscillator
plt.figure(figsize=(12, 4))
plt.plot(df['Date'], df['Stochastic Oscillator'], label='Stochastic Oscillator', color='blue')
plt.axhline(y=80, color='red', linestyle='--', label='Upper Boundary (80)')
plt.axhline(y=20, color='green', linestyle='--', label='Lower Boundary (20)')
plt.title('Stochastic Oscillator')
plt.legend()
plt.grid()
plt.show()
# Calculate PPI
percentage_price_indicator_signals(df)
# chart for PPI
plt.figure(figsize=(12, 4))
plt.plot(df['Date'], df['Percentage Price Indicator'], label='PPI', color='blue')
plt.axhline(y=0.8, color='red', linestyle='--', label='Threshold (0.8)')
plt.axhline(y=0.2, color='green', linestyle='--', label='Threshold (0.2)')
plt.title('Percentage Price Indicator (PPI)')
# Plot buy and sell signals
plt.plot(df['Date'][df['PPI Buy'] == 1], df['Percentage Price Indicator'][df['PPI Buy'] == 1], 'g^', markersize=8, label='PPI Buy Signal')
plt.plot(df['Date'][df['PPI Sell'] == 1], df['Percentage Price Indicator'][df['PPI Sell'] == 1], 'rv', markersize=8, label='PPI Sell Signal')
plt.legend()
plt.grid()
plt.show()
TheoreticallyOptimalStrategy.py
import pandas as pd
class TheoreticallyOptimalStrategy:
def testPolicy(self, symbol, sd, ed, sv):
dates = pd.date_range(sd, ed)
trades = pd.DataFrame(index=dates)
trades[symbol] = 0
# Determine the number of trading days
num_trading_days = len(dates)
# Determine the maximum number of shares that can be bought/sold
max_shares = 1000
# Buy and sell alternatively each day (starting with buying)
for i in range(num_trading_days):
if i % 2 == 0:
trades.iloc[i] = max_shares
else:
trades.iloc[i] = -max_shares
return trades
testproject.py
import datetime as dt
import TheoreticallyOptimalStrategy as tos
import indicators as ind
import marketsimcode as ms
def calculate_portfolio_statistics(start_date, end_date, symbol, start_value):
tos_strategy = tos.TheoreticallyOptimalStrategy()
trades = tos_strategy.testPolicy(symbol, start_date, end_date, start_value)
portvals = ms.compute_portvals(trades, start_date, end_date, start_value)
daily_returns = portvals / portvals.shift(1) - 1
daily_returns.iloc[0] = 0
cumulative_return = portvals.iloc[-1] / portvals.iloc[0] - 1
stdev_daily_returns = daily_returns.std()
mean_daily_returns = daily_returns.mean()
return cumulative_return, stdev_daily_returns, mean_daily_returns
if __name__ == "__main":
symbol = "JPM"
start_date = dt.datetime(2008, 1, 1)
end_date = dt.datetime(2009, 12, 31)
start_value = 100000
cumulative_return, stdev_daily_returns, mean_daily_returns = calculate_portfolio_statistics(start_date, end_date, symbol, start_value)
print(f"Cumulative return: {cumulative_return:.6f}")
print(f"Stdev of daily returns: {stdev_daily_returns:.6f}")
print(f"Mean of daily returns: {mean_daily_returns:.6f}")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Based on the code you provided it seems that there are some indentation issues in your indicatorspy file Python relies heavily on proper indentation t...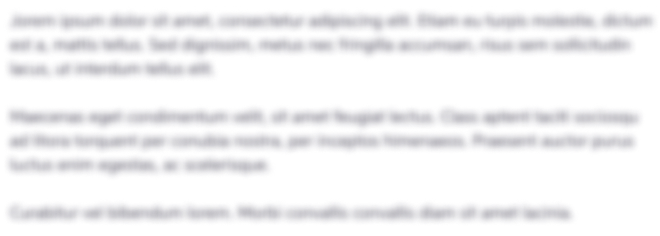
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started