Question
why my tests are all failing ? how to fix? public class GuessMe{ /* Here you will be creating your instance variables. When making instance
why my tests are all failing ? how to fix?
public class GuessMe{
/* Here you will be creating your instance variables. When making instance variables, we think * about what information we need to store between method calls. The info we will need to store * is: * * 1) the word the user is trying to guess. We will need a String instance variable called 'word' for that. * 2) the number of guesses the user has left. We will need an int called 'remainingGuesses' for that. * 3) we need to know if the word has been found. We will need a boolean called 'wordFound' for that. * 4) we need a random number generator. We will need a Random object called 'rand' for that. * * Be sure to use the suggested variable names exactly, or you won't pass many of the tests. */ //Instance variables here: private String word; private int remainingGuesses; private boolean wordFound; private Random rand;
/* This is the first of two constructors. This one is used in the main method. We initialize each * of our instance variables here. The player will start off with 10 guesses, the word obviously * has not been found yet. You will need to initialize 'rand' before you can generate the word, and * you will want to use the generateWord() helper method at the bottom of this file to initialize * 'word' that way you don't have to write the same code a ton of times. */ public GuessMe(){ remainingGuesses = 10; wordFound = false; rand=new Random(); word = generateWord(); } public GuessMe(int seed){ remainingGuesses = 10; wordFound = false; word = generateWord(); rand= new Random(seed); } /* This is our play method. It takes in a String called 'str' and compares it to the word the * player is trying to guess 'word'. It returns an int representing the number of letters which * are in the exact same place in both words. For example, if the word to find were "ABCD" play * would return: * * 1 on "AAAA" * 2 on "AAAD" * 4 on "ABCD" * 4 on "abcd" * NOTE these values are ONLY for the example word "ABCD" and will not be the same for your random * word. Looping and using charAt() on the strings would be very useful here. Remember we do not * care about upper/lower case here, so be sure to account for that. * We also want to decrement the user's remaining guesses, and in the event they guess the word * we will also update wordFound to true before returning 4. */ public int play(String str){
int matches = 0; if(word.charAt(0) == str.charAt(0)) matches+=1; if(word.charAt(1) == str.charAt(1)) matches+=1; if(word.charAt(2) == str.charAt(2)) matches+=1; if(word.charAt(3) == str.charAt(3)) matches+=1; remainingGuesses -= 1; if(matches==4){ wordFound=true; } return matches; } /* isOver simply returns true if the game is over, which is either when the player runs * out of guesses or when they have found the correct word. It returns false otherwise. */ public boolean isOver(){ return remainingGuesses == 0 || wordFound; } /* isWin returns true if the player has correctly guessed the word and false otherwise. */ public boolean isWin(){ return wordFound == true; } public int getRemainingGuesses(){ return remainingGuesses; } /* reset returns nothing but resets the game. 'remainingGuesses' and 'wordFound' go back to * their default values and a new word is generated with generateWord(). */ public void reset(){ word=generateWord(); remainingGuesses = 10; wordFound = false; } public String getWord(){ return word; } * We will go about writing this by using our Random object. We will call nextInt(4) on our * Random object a total of four times to generate four different ints. Specifying 4 in the * method call forces it to generate an int between 0 and 4, including 0 but not including 4. * The ints correspond to a letter to add to our word as such: * 0: 'A' * 1: 'B' * 2: 'C' * 3: 'D' * We can build our String by starting with local variable which is an empty String ("") which we can * call retStr. Using String concatination (+=), we can add letters to our String based on the int * generated. Once we have done this 4 times, we can return the String. We do not use any instance * variables in this method. It may be helpful to use a loop here. */ private String generateWord(){ String aRandomWord = ""; for (int i = 0; i < 4; i++) { int randomNumber = rand.nextInt(4); if (randomNumber == 0) { aRandomWord += "A"; } else if (randomNumber == 1) { aRandomWord += "B"; } else if (randomNumber == 2) { aRandomWord += "C"; } else if (randomNumber == 3) { aRandomWord += "D"; } } return aRandomWord; } }
public class GuessMeTest { private GuessMe game;
/** Fixture initialization (common initialization * for all tests). **/ @Before public void setUp() { game = new GuessMe(1234); } /** Ensures word variable exists **/ @Test public void instanceExistsTest1() { String word = ""; try{ Field wordField = GuessMe.class.getDeclaredField("word"); wordField.setAccessible(true); word = (String)wordField.get(game); Assert.assertEquals("word instance variable exists", 1, 1); } catch (Exception e){ Assert.fail("word instance variable doesn't exist"); } } /** Ensures remainingGuesses variable exists **/ @Test public void instanceExistsTest2() { int remainingGuesses = -1; try{ Field intField = GuessMe.class.getDeclaredField("remainingGuesses"); intField.setAccessible(true); remainingGuesses = (Integer)intField.get(game); Assert.assertEquals("remainingGuesses instance variable exists", 1, 1); } catch (Exception e){ Assert.fail("remainingGuesses instance variable does not exist"); } } /** Ensures constuctor initializes word correctly **/ @Test public void constructorTest1(){ String word = ""; try{ Field wordField = GuessMe.class.getDeclaredField("word"); wordField.setAccessible(true); word = (String)wordField.get(game); } catch (Exception e){} Assert.assertEquals("constructor initializes word correctly", "CBDA", word); } /** Ensures constuctor initializes remainingGuesses correctly **/ @Test public void constructorTest2(){ int remainingGuesses = -1; try{ Field intField = GuessMe.class.getDeclaredField("remainingGuesses"); intField.setAccessible(true); remainingGuesses = (Integer)intField.get(game); } catch (Exception e){} Assert.assertEquals("constructor initializes word correctly", 10, remainingGuesses); } /** Ensures constuctor initializes rand correctly **/ @Test public void constructorTest4(){ Random rand = null; try{ Field randField = GuessMe.class.getDeclaredField("rand"); randField.setAccessible(true); rand = (Random)randField.get(game); } catch (Exception e){} Assert.assertNotNull("Constructor initializes rand", rand); int i = rand.nextInt(); Assert.assertEquals("Constructor initializes rand correctly", -611652875, i); } /** Ensures reset resets remainingGuesses correctly **/ @Test public void resetTest2(){ game.reset(); int remainingGuesses = -1; try{ Field intField = GuessMe.class.getDeclaredField("remainingGuesses"); intField.setAccessible(true); remainingGuesses = (Integer)intField.get(game); } catch (Exception e){} Assert.assertEquals("reset resets remainingGuesses correctly", 10, remainingGuesses); } /** tests isOver on remainingGuesses **/ @Test public void isOverTest1(){ for(int i = 0; i < 10; i++){ game.play("AAAA"); } Assert.assertEquals("isOver works when remaining guesses are zero", true, game.isOver()); } /** tests isOver on wordFound **/ @Test public void isOverTest2(){ game.play("CBDA"); Assert.assertEquals("isOver works when word has been found", true, game.isOver()); } @Test public void isWinTest2(){ game.play("aaaa"); Assert.assertEquals("isWin works when word has not been found, but is implemented", false, game.isWin()); game.play("cbda"); Assert.assertEquals("isWin works when word has not been found, but is implemented", true, game.isWin()); } @Test public void getRemainingGuessesTest1(){ game.play("aaaa"); game.play("bbbb"); game.play("cccc"); game.play("dddd"); Assert.assertEquals("getRemainingGuess returns correct value", 6, game.getRemainingGuesses()); } @Test public void playTest1(){ Assert.assertEquals("play returns correct value on win", 4, game.play("CBDA")); } /** tests play on win lower case **/ @Test public void playTest2(){ Assert.assertEquals("play returns correct value on lower case", 4, game.play("cBda")); } @Test public void playTest3(){ Assert.assertEquals("play returns correct value on non win", 1, game.play("AAAA")); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
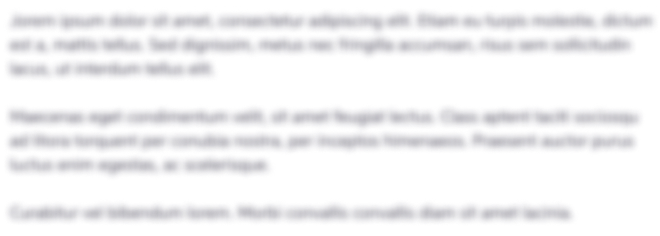
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started