Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Would it be possible for help in filling out the student to write functions REVERSI.H #ifndef REVERSI_HPP #define REVERSI_HPP #include #include /*******************************************************/ /* Add destructors,
Would it be possible for help in filling out the "student to write" functions REVERSI.H #ifndef REVERSI_HPP #define REVERSI_HPP #include#include /*******************************************************/ /* Add destructors, copy constructors, and assignment */ /* operators to any class that requires one. */ /*******************************************************/ /** * Square class models a location in Reversi * that can either be white, black, or free * using an enumerated type. */ struct Square { /** * Enumeration values act as named integer constants. * So Square::FREE acts as an integral constant 0, * Square::WHITE acts as an integral constant 1, and * Square::BLACK acts as an integral constant 2. */ enum SquareValue { FREE = 0, WHITE, BLACK }; // Data member SquareValue value_; /** * Constructor using default arguments to allow * no initial value that will default to FREE */ Square(SquareValue value = FREE) : value_(value) { } /*------------------- STUDENT TO WRITE -----------------*/ /** * Assigns the internal SquareValue enumeration * to the provided input argument. * Note: The input is a SquareValue not a full Square */ Square& operator=(SquareValue value); /*------------------- STUDENT TO WRITE -----------------*/ /** * Comparison operators to compare a Square * to a SquareValue enumeration value (i.e. * Square::FREE, Square::WHITE, Square::BLACK) * Note: We are comparing a SquareValue not a full Square */ bool operator==(SquareValue value) const; bool operator!=(SquareValue value) const; /** * Flip the current SquareValue from WHITE to BLACK or * BLACK to WHITE */ void flip(); }; /*------------------- STUDENT TO WRITE -----------------*/ /** * ostream operator for a Square object that outputs * '-' for FREE, 'B' for BLACK, and 'W' for WHITE. * Note: Since data members in the Square struct are public * we do not need to make this a friend function. */ std::ostream& operator history_; }; #endif
REVERSI.CPP
#include#include #include "reversi.h" using namespace std; void Square::flip() { switch (value_) { case WHITE: value_ = BLACK; break; case BLACK: value_ = WHITE; break; default: break; } } Square::SquareValue opposite_color(Square::SquareValue value) { switch (value) { case Square::WHITE: return Square::BLACK; case Square::BLACK: return Square::WHITE; default: throw invalid_argument("Illegal square value"); } } Square& Board::operator()(char row, size_t column) { if (!is_valid_location(row, column)) { throw out_of_range("Bad row index"); } size_t row_index = row_to_index(row); return squares_[row_index][column - 1]; } Square const& Board::operator()(char row, size_t column) const { if (!is_valid_location(row, column)) { throw out_of_range("Bad row index"); } size_t row_index = row_to_index(row); return squares_[row_index][column - 1]; } bool Board::is_legal_and_opposite_color( char row, size_t column, Square::SquareValue turn) const { if (is_valid_location(row, column)) { size_t row_index = row_to_index(row); return squares_[row_index][column - 1] != Square::FREE && squares_[row_index][column - 1] != turn; } return false; } bool Board::is_legal_and_same_color( char row, size_t column, Square::SquareValue turn) const { if (is_valid_location(row, column)) { size_t row_index = row_to_index(row); return squares_[row_index][column - 1] == turn; } return false; } bool Board::is_valid_location(char row, size_t column) const { size_t row_index = row_to_index(row); return row_index black_count) { cout
prompt
We will change a few rules from those listed on the above site. 1. The game ends when the current player does not have a legal move (rather than ending the game only when BOTH players do not have a legal move). The winner is still the one with the most pieces matching their color. 2. We will support boards of EVEN-number sizes from 4x4 to 26x26 (and not just 8x8). The user will specify the dimension of the board at the command lines (e.g. ./test-reversi 4 should create and play a 4x4 version of the game). o Any provided dimension that is ODD or outside the range of 4 to 26 should cause Invalid size to be printed and the program should exit by returning 1 from maino o If no command line argument is provided, default to a 4x4 game board. Additional features and game play: 1. Without a GUI, we will require simple console I/O. Thus, we will output the current board state before accepting each players selection on their turn. The logic to output the board is already provided by Board::print (though we will want to support the operator for storing the Board. You MAY use it to to store the checkpoints. 3. You may not allocate variable size arrays ON THE STACK. Recall, that in C/C++, arrays whose size depends on a runtime variable should be allocated on the heap. 4. You may not alter the general class structure provide or any of the given member function prototypes but may add additional global or private member functions. The only public member functions you may add are destructors, copy constructors, and/or assignment operators. 5. You may not alter any provided code given. If that code calls a specific function or uses an operator that is not defined, you must define that function. 6. std::stringstream is allowed. In addition, you MAY use functions fromand but nothing from except std::max() and std::min() 7. Read the comments/documentation in the provided skeleton code. They acts as REQUIREMENTS just as much as these instructions and will lead to deductions if not heeded. We will change a few rules from those listed on the above site. 1. The game ends when the current player does not have a legal move (rather than ending the game only when BOTH players do not have a legal move). The winner is still the one with the most pieces matching their color. 2. We will support boards of EVEN-number sizes from 4x4 to 26x26 (and not just 8x8). The user will specify the dimension of the board at the command lines (e.g. ./test-reversi 4 should create and play a 4x4 version of the game). o Any provided dimension that is ODD or outside the range of 4 to 26 should cause Invalid size to be printed and the program should exit by returning 1 from maino o If no command line argument is provided, default to a 4x4 game board. Additional features and game play: 1. Without a GUI, we will require simple console I/O. Thus, we will output the current board state before accepting each players selection on their turn. The logic to output the board is already provided by Board::print (though we will want to support the operator for storing the Board. You MAY use it to to store the checkpoints. 3. You may not allocate variable size arrays ON THE STACK. Recall, that in C/C++, arrays whose size depends on a runtime variable should be allocated on the heap. 4. You may not alter the general class structure provide or any of the given member function prototypes but may add additional global or private member functions. The only public member functions you may add are destructors, copy constructors, and/or assignment operators. 5. You may not alter any provided code given. If that code calls a specific function or uses an operator that is not defined, you must define that function. 6. std::stringstream is allowed. In addition, you MAY use functions from and but nothing from except std::max() and std::min() 7. Read the comments/documentation in the provided skeleton code. They acts as REQUIREMENTS just as much as these instructions and will lead to deductions if not heeded
Step by Step Solution
There are 3 Steps involved in it
Step: 1
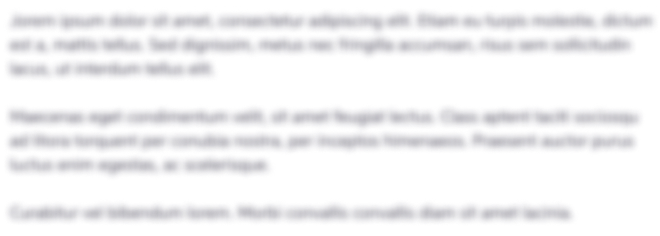
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started