Question
Would you please show me easy and simple code for java? Selection sort is a sorting algorithm that treats the input as two parts, a
Would you please show me easy and simple code for java?
Selection sort is a sorting algorithm that treats the input as two parts, a sorted part and an unsorted part, and repeatedly selects the proper next value to move from the unsorted part to the end of the sorted part.
The array is divided into two parts, the sorted part being at the left end and the unsorted part at the right end. The sorted part is initially empty and the full array is initially the unsorted part. The smallest element from the unsorted part is chosen and swapped with the furthest left element in the unsorted part, essentially making that smallest element part of the sorted array, as the 'boundary' between the sorted and unsorted parts is incremented by one each time this swap is performed.
A visual aid for selection sort can be seen here.
public class Sorting { public static void main(String[] args) { // Example input. int numbers[] = {10, 2, 78, 4, 45, 32, 7, 11}; int i;
int numbersSize = numbers.length; // Print array pre sorting. System.out.print("UNSORTED: "); for (i = 0; i < numbersSize; i++) { System.out.print(numbers[i] + " "); } System.out.println();
// Run Selection Sort. selectionSort(numbers);
// Print array post sorting. System.out.print("SORTED: "); for (i = 0; i < numbersSize; i++) { System.out.print(numbers[i] + " "); } System.out.println(); }
public static void selectionSort(int numbers[]) { // FIXME } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
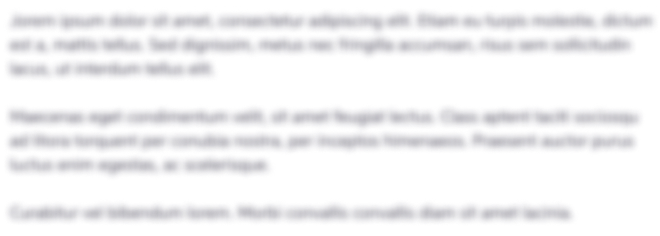
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started