Question
Write a c code and use the following structure: struct student { int id; char name[20]; }; Complete a function that scans an array of
Write a c code and use the following structure:
struct student {
int id;
char name[20];
};
Complete a function that scans an array of students for a student with a given ID. If the student was located, the function should return true and the string (f_s_name) should be updated to include the student's name. If the list contains no students with that ID, the function should return false. In the worst case, your function must run in O(n) time, and in the best case, it must run in O(1) time, where n represents the number of student records in the array. // f_id(id, arr, n, f_s_name) looks for a student in arr with the provided id; // returns true if such a student is found and f_s_name is updated to // hold the student's name; otherwise returns false // requires: arr has length n // each student in arr has a unique id // f_s_name points to enough memory to hold a name bool find_id(int id, struct student arr[], int n, char * f_s_name);
bool find_id(int id, struct student arr[], int n, char * f_s_name){
}
Next, create an implementation for an "opposite" search, which is a feature that looks for students with a certain name. (It's possible that two students have the same name.) In the worst-case scenario, the function must run in O(n) time.
// f_name(name , arr, n, ids) looks for a student(s) in arr with the provided name; // returns the number of students found and the array ids is updated to // contain the id numbers of those students // requires: arr has length n // each student in arr has a unique id // s_id points to enough memory to hold the found student ids int f_name(char * name , struct student arr[], int n, int s_id[]);
int f_name(char * name , struct student arr[], int n, int s_id[]){
}
Finally, complete implementation for the following prototype of a function that changes the name of a given student. name_new // change_name(s, name_new) renames the student s to have the name given by name_new // requires: s points to a valid student that can be modified // name_new points to a valid string of length at most 19 void change_name(struct student * s, char * name_new);
Now for testing, use assert and strcmp to test your functions. A number of student records have already been provided to you below in order to help facilitate your testing. Provide enough test coverage to be sure that your functions are working correctly in every possible case. int main(void) { struct student 1s = {5679364 , "Bob"}; struct student 2s = {1112453 , "Joshua"}; struct student 3s = {9879989 , "Curt"}; }
Give an explanation of the function implementations. Your clarification should be brief, to-the-point, and demonstrate how your implementations operate. Explain why f_id and f_name running times are within the required limits. In the best case, clarify how long f_name takes to run. Your interpretation should be based on the assumption that each student's name is stored in a maximum of 20 characters.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
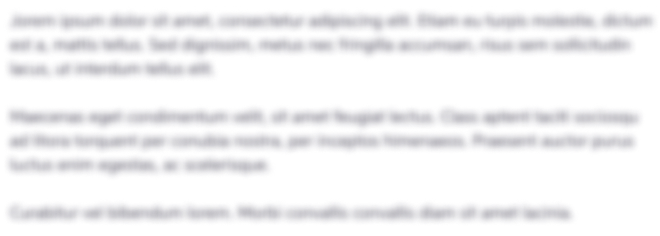
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started