Question
Write a C program, named sort.h, containing the following functions. Function void selection_sort(int *a, int left, int right), which sorts the elements of int array
Write a C program, named sort.h, containing the following functions.
- Function void selection_sort(int *a, int left, int right), which sorts the elements of int array *a from index left to right in increasing order, using the selection sort algorithm.
- Function void quick_sort(int *a, int left, int right), which sorts the elements of int array *a from index left to right in increasing order, using the quick sort algorithm.
- Auxiliary functions:
// this function displays array a[n] in format "%3d " and 10 numbers each line
void diaplay_array(int *a, int n);
// this function copies array a[n] to array b[n]
void copy_array(int *a, int *b, int n);
// this function tests if array a[n] is sorted in increasing order, returns 1 if true or 0 otherwise
int is_sorted(int *a, int n);
// this function swap the values in reference locations
void swap(int *x, int *y);
sort.h
#include
#include
void swap(int *first, int *second);
void display_array(int *a, int n);
void selection_sort(int *a, int left, int right);
void quick_sort(int *a, int left, int right);
void copy_array(int *a, int *b, int n);
int is_sorted(int *a, int n);
void display_array(int *a, int n)
{
int i;
for (i=0; i if (i%10 == 0) printf(" ");printf("%3d ", *(a+i));}printf(" ");}void swap(int *first, int *second){int temp = *first;*first = *second;*second = temp;}void copy_array(int *a, int *b, int n){// your implementation}int is_sorted(int *a, int n){// your implementation}void selection_sort(int *a, int left, int right){// your implementation}void quick_sort(int *a, int left, int right){// your implementation}
Use the provided main function program to test your functions in sort.h :
#include
#include "sort.h"
int main(int argc, char *args[])
{
int a[] = {3, 1, 4, 5, 2, 7, 6, 9, 8}; // input array for correctness testing
int n = sizeof(a)/sizeof(int); // array length
int b[n], i; // array to sort
printf("Algorithm correctness testing: ");
printf("Input array:");
display_array(a, n);
printf("Is sorted:%d ", is_sorted(a, n));
printf("Selection sort:");
copy_array(a, b, n);
selection_sort(b, 0, n-1);
display_array(b, n);
printf("Is sorted:%d ", is_sorted(b, n));
printf("Quick sort:");
copy_array(a, b, n);
quick_sort(b, 0, n-1);
display_array(b, n);
printf("Is sorted:%d ", is_sorted(b, n));
printf("Algorithm runtime testing: ");
//run time measurement
clock_t t1, t2;
int len = 2000;
int a1[len];
int b1[len];
//generate randomly an array of len elements more modular len
srand(time(NULL));
for (i=0;i
a1[i] = rand()% len;
}
//run time measuring for selection_sort
int m1 = 10;
t1=clock();
for (i=0; i< m1; i++) {
copy_array(a1, b1, len);
selection_sort(b1, 0, len-1);
if (!is_sorted(b1, len)) printf("not sorted:%d ");
}
t2=clock();
double time_span1 = (double) t2-t1;
printf("time_span(selection_sort(%d numbers) for %d times): %0.1f (ms) ", len, m1, time_span1);
//run time measuring for quick_sort
int m2 = 1000;
t1=clock();
for (i=0; i< m2; i++) {
copy_array(a1, b1, len);
quick_sort(b1, 0, len-1);
if (!is_sorted(b1, len)) printf("not sorted:%d ");
}
t2=clock();
double time_span2 = (double) t2-t1;
printf("time_span(quick_sort(%d numbers) for %d times): %0.1f (ms) ", len, m2, time_span2);
printf("time_span(selection_sort(%d numbers))/time_span(quick_sort(%d numbers)): %0.1f ", len, len,
(time_span1/time_span2)*(m2/m1));
return 0;
}
public test
Algorithm correctness testing:
Input array:
3 1 4 5 2 7 6 9 8
Is sorted:0
Selection sort:
1 2 3 4 5 6 7 8 9
Is sorted:1
Quick sort:
1 2 3 4 5 6 7 8 9
Is sorted:1
Algorithm runtime testing:
time_span(selection_sort(2000 numbers) for 10 times): 71.0 (ms)
time_span(quick_sort(2000 numbers) for 1000 times): 247.0 (ms)
time_span(selection_sort(2000 numbers))/time_span(quick_sort(2000 numbers)): 28.7
Step by Step Solution
There are 3 Steps involved in it
Step: 1
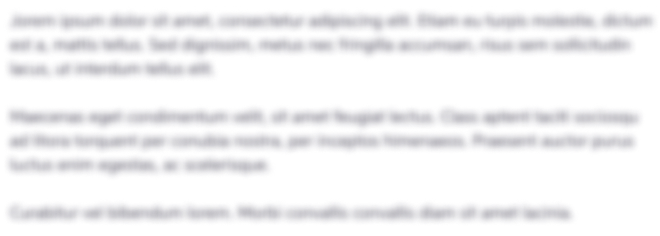
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started