Question
Write a C program, polynomial.h, containing the following polynomial operation functions: 1- float horner(float p[], int n, float x), which computes and returns the value
Write a C program, polynomial.h, containing the following polynomial operation functions:
1- float horner(float p[], int n, float x), which computes and returns the value of the following (n-1)-th degree polynomial p(x) of coefficients p[0], , p[n-1]. p(x) = p[0]xn-1 + p[1]xn-2 ++ p[n-2]x1 + p[n-1]x0
It is required to use Horners algorithm (supplementary link).
2- void derivative(float p[], int n, float d[]), which computes the derivative of input (n-1)-th degree polynomial by p[], output the derivative of (n-2)-th degree polynomial to array d[]. The derivative of the above polynomial p(x) is as follows. p(x) = (n-1)* p[0]xn-2+ (n-2)p[1]xn-3 ++ p[n-2]x0
3- float newton(float p[], int n, float x0), which finds an approximate real root x of polynomial p(x) using the Newtons method with start position x0. Use the fault tolerant 1e-6 (or 0.000001) as a stop condition, i.e., if r is the actual root, stop the iteration if |x-r|<1e-6 or |p(x)| < 1e-6.
Use the provided the main function program polynomial_main.c to test the above functions. The output should be like the following.
Public test
command: gcc polynomial_main.c -o polynomial command: polynomial p(0.00)=1.00*0.00^3+2.00*0.00^2+3.00*0.00^1+4.00*0.00^0=4.00 p(1.00)=1.00*1.00^3+2.00*1.00^2+3.00*1.00^1+4.00*1.00^0=10.00 p(10.00)=1.00*10.00^3+2.00*10.00^2+3.00*10.00^1+4.00*10.00^0=1234.00 d(0.00)=3.00*0.00^2+4.00*0.00^1+3.00*0.00^0=3.00 d(1.00)=3.00*1.00^2+4.00*1.00^1+3.00*1.00^0=10.00 d(10.00)=3.00*10.00^2+4.00*10.00^1+3.00*10.00^0=343.00 root=-1.65 p(-1.65)=0.00
polynomial_main.c:
*/ #include
void display_polynomial(float p[], int n, float x) { int i; for (i=0; i return 0; } polynomial.h:/* * your program signature */ #ifndef POLYNOMIAL_H #define POLYNOMIAL_H #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
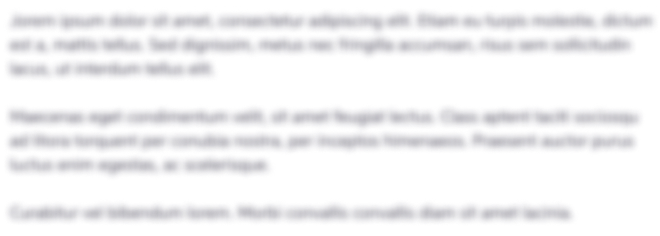
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started