Question
Write a C++ program to implement Shorted Job First algorithm. Make sure to use Vectors! Here is the code of FCFS Process Scheduling. I need
Write a C++ program to implement Shorted Job First algorithm. Make sure to use
Vectors!
Here is the code of FCFS Process Scheduling. I need Shortest Job First using the vectors
#include
#include
using namespace std;
struct process{
int no;
int burst;
int wait;
int arrival;
int turn_around;
};
void FCFS(process*, int);
void SJF(process*, int);
int main()
{
int n;
cout<<"Enter total number of processes: ";
cin>>n;
process p[n],temp[n];
for (int i=0;i { p[i].no=i+1; cout< cout<<"Enter arrival time and burst time: "; cin>>p[i].arrival; cin>>p[i].burst; p[i].wait=0; p[i].turn_around=0; } FCFS(p,n); SJF(p,n); return 0; } } void FCFS(process p[],int n){ //creating vector of processes vector //creating a temporary structure which will take in values //coming from main() and push back into a vector. process temp1; cout<<" \t\tFirst Come First Serve \t\t**********************"; for(int i=0;i { temp1.no=p[i].no; temp1.arrival=p[i].arrival; temp1.wait=p[i].wait; temp1.burst=p[i].burst; temp1.turn_around=p[i].turn_around; temp.push_back(temp1); } //calculating waiting time and turn around time for(int i=0;i if (i!=0){ temp[i].wait=temp[i-1].turn_around- temp[i].arrival+temp[i-1].arrival; temp[i].turn_around=temp[i].wait+temp[i].burst; } else{ temp[i].turn_around=temp[i].burst; } } //calcuating average of both for each process. int sum_waiting_time = 0, sum_turnaround_time=0; for(int i=0; i sum_waiting_time += temp[i].wait; sum_turnaround_time += temp[i].turn_around; } cout< printf("Total Waiting Time : %-2d ", sum_waiting_time); printf("Average Waiting Time : %-2.2lf ", (double)sum_waiting_time / (double) n); printf("Total Turnaround Time : %-2d ", sum_turnaround_time); printf("Average Turnaround Time : %-2.2lf ", (double)sum_turnaround_time / (double) n); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
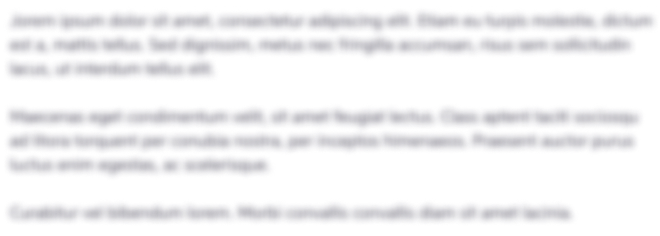
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started