Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a C program to implement the following requirement: Input: The program will read data from a text file named DATA.IN which has 3
Write a C program to implement the following requirement:
Input: The program will read data from a text file named "DATA.IN which has lines:
The first line consists of a string C either string LT or string GT and a float number D
which are separated by a single white space
The second line contains student data, where each student's information is separated by
white spaces. Each student's data includes their date of birth in the format MMDDYYYY
followed by their GPA with a dash used to separate the date of birth and GPA parts.
The date of birth and GPA are surrounded by nondigit characters.
The third line also contains student data in the same format as the second line.
For example, given a string S 'abcdassabvcpldl the extracted date would be
and the extracted GPA would be
Requirement: Complete the functions provided in the attached template to accomplish the
following tasks:
Read student data from DATAIN Store each students data as a node in a linked list.
There are linked lists. The first list consists of the data of students on line The
second list consists of the data of students on line
Merge two linked lists into a single list third list
Filter the third list based on GPA criteria.
a If C is LT filter the students with a GPA less than D
b If C is GT filter the students with a GPA greater than D
Reverse the third list.
Print each linked list to stdout, where each node is represented as MMDDYYYYABC
separated by space and print each linked list on one line.
Template:
PART : FIXED code
DON'T CHANGE THIS PART
#include
#include
#define MAXWORDCHAR
#define GPA "GPA"
#define DATE "DATE"
struct Date
int year;
int month;
int day;
;
typedef struct Date Date;
struct Node
Date dob;
float gpa;
struct Node next;
;
typedef struct Node Node;
void readDataFILE char float Node Node ;
Node mergeListNode Node ;
Node filterListNode char float;
Node reverseListNode ;
void printListNode ;
int main
FILE fp fopenDATAINr;
Node headL NULL;
Node headL NULL;
char controlString;
float targetGPA;
readDatafp &controlString, &targetGPA, &headL &headL;
printListheadL;
printf
;
printListheadL;
printf
;
Node head mergeListheadL headL;
head filterListhead controlString, targetGPA;
head reverseListhead;
printListhead;
fclosefp;
PART : Student works
Define supported functions here.
void readDataFILE fp char controlString float targetGPA Node headL Node headL
TODO: read data with file pointer fp:
LINE: controlString targetGPA
LT or GTfloat number
LINE: headL is the head of list
LINE: headL is the head of list
Node mergeListNode headL Node headL
TODO: add list into the end of list return the head of result list
Node filterListNode head char controlString float targetGPA
TODO: Filter the third list based on GPA criteria.
If controlString is LT filter the students with a GPA less than targetGPA.
If controlString is GT filter the students with a GPA greater than targetGPA.
return head of filtered list
Node reverseListNode head
TODO: reverse the list
return the new head
void printListNode head
TODO: print the linked list to stdout
GT
abcdkkdsdaa dfgrg
afd xekdldw
SAMPLE OUTPUT
SAMPLE INPUT
LT
asbva crfqxzdsdf
sd defdcsqde
SAMPLE OUTPUT
Step by Step Solution
There are 3 Steps involved in it
Step: 1
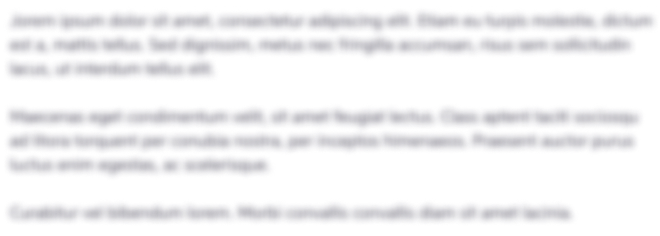
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started