Question
Write a class in C++ called Set that can hold 100 positive integer numbers from 0-99. You may implement this as an array or vector
Write a class in C++ called Set that can hold 100 positive integer numbers from 0-99.
You may implement this as an array or vector of boolean elements.
Add constructor(s), and the following methods:
1. void add( int i ) // add the number i to the set.
2. bool belongs(int i) // return true if i is a member of the set
3. void difference( Set B) // removes B from the set A where A is the current set so A= A-B
4. void union(Set B)// performs A U B // where A is the current set and the result is stored in A
5. void intersect (Set B) // performs A B // where A is the current set and the result is stored in A
6. string toString() //displays the set in roster notation {1,2,4} etc.
Write a client that instantiates two sets A and B. A = {2,4,6,8,10} B = {1,2,3,4}
Perform the operations in this order and call (Please include output statements before each call to explain your call as follows)
1. A.toString() //printing A
2. B.toString() //printing B
3. A.add(12) // adding 12 to A
4. A.toString() //printing A
5. A.belongs(4) // does 4 belong to A
6. A.belongs(11) // does 11 belong to A
7. A.difference(B) // remove B from A
8. A.toString() //printing A
9. A.union(B) // union of A and B
10. A.toString() // printing A
11 A.intersect(B) // intersecting A and B
12.A.toString()// printing A
I have the following so far:
//.Set.h
#ifndef __SET_H
#define __SET_H
#include
#include
using namespace std;
class Set
{
public:
//vector to store integers
vector
int len;
public:
Set()
{
len = 0;
}
void setAdd(int i)
{
//push integer to the vector and increment length
set.push_back(i);
len++;
}
bool belongs(int i)
{
//check existance of given value
int ind;
bool res = false;
for(ind = 0;ind
{
if(set[ind] == i)
{
res = true;
break;
}
}
return res;
}
void setDifference(Set B)
{
int i;
int removed = 0;
cout
for(i = 0;i { //find current set elements found in Set B //if yes remove it // then increment the removed //cause when we acecss using set[] we dont want to access removed element if(B.belongs(set[i - removed])) { cout set.erase(set.begin() + (i - removed)); removed++; } } //update lengthh len -= removed; } void setUnion(Set B) { //set union int i; int len2 = B.len; cout for(i = 0;i { //find set b elements are in current set if not add to the list if(belongs(B.set[i]) == false) { cout set.push_back(B.set[i]); len++; } } } void setIntersect(Set B) { int i; int removed = 0; cout for(i = 0;i { //find existance of current set in set B if(B.belongs(set[i - removed]) == false) { //remove the elements //increment removed count cout set.erase(set.begin() + (i - removed)); removed++ ; } } //update length len -= removed; } void toString() { //print set elements cout int i; for(i = 0;i { cout } cout } }; #endif #include "Set.h" #include int main() { /* * to generate random numbers and test srand(99999999); Set A,B; int i; for(i = 1;i { A.setAdd(rand()%100+1); } for(i = 1;i { B.setAdd(rand()%100 + 1); } A.toString(); B.toString(); A.setDifference(B); A.setUnion(B); A.setIntersect(B); * //print result after above operations,you may remove above operations and test individually A.toString(); */ int i; int n; int num; printf("Enter Number of elements in set A: "); scanf("%d",&n); Set A,B; for(i = 0;i { printf("Enter A[%d]: ",i); scanf("%d",&num); A.setAdd(num); } printf("Enter Number of elements in set B: "); scanf("%d",&n); for(i = 0;i { printf("Enter B[%d]: ",i); scanf("%d",&num); B.setAdd(num); } printf("- After Loading elements into Set A - "); A.toString(); printf(" - After Loading elements into Set B - "); B.toString(); printf(" - Set Difference - "); A.setDifference(B); A.toString(); printf(" - Set Union - "); A.setUnion(B); A.toString(); printf(" - Set Intersect - "); A.setIntersect(B); A.toString(); //print result after above operations,you may remove above operations and test individually return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
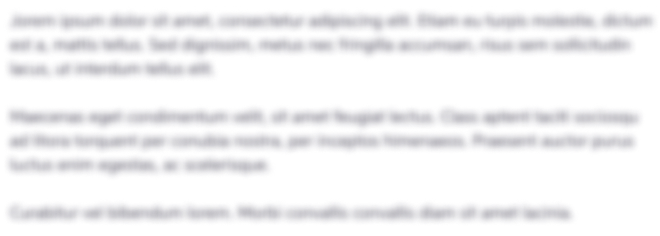
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started