Question
Write a class named BitWord that holds an unsigned int and implements the following interface: class BitWord { public: explicit BitWord(unsigned int n = 0);
Write a class named BitWord that holds an unsigned int and implements the following interface:
class BitWord
{
public:
explicit BitWord(unsigned int n = 0);
void set(size_t pos);
void reset(size_t pos);
void flip(size_t pos);
bool test(size_t pos) const;
unsigned int extract(size_t m, size_t n) const;
operator unsigned int () const;
friend ostream& operator<<(ostream& os, const BitWord& b);
};
The conversion operator to unsigned int just returns the contained integer. The extract function returns the numeric value of a range of bits, as we did with exp above. The type size_t is an unsigned integer type defined in .
Bellow is some other helpful information:
Bitwise operations are needed when processing bit flags in operating systems calls and when packing Boolean values into integers to conserve space. The bitwise operators are:
<< shift left
>> shift right
| bitwise OR
& bitwise AND
^ bitwise XOR (exclusive-or)
~ bitwise NOT (flip all bits)
These operators apply to integers and return new integer results. There are also associated assignment operators that modify their left operand: <<=, >>=, |=, &=, ^=, ~=.
The shift operators take the integer to be processed as a left operand and the number of bit positions to shift as a right operand. The left operand is usually an unsigned integer, because a shift-right replicates the sign bit when processing a signed integer; with unsigned integers zeroes are introduced.
To determine whether a particular bit is set or not, construct a mask, a like-sized integer with a 1 in the position of interest and zeroes elsewhere. Then AND that mask with the original integer. If the result is non-zero, the bit is set. To illustrate, suppose we want to know if the bit in position 4 is set in the integer n. Since bits are numbered right-to-left from 0, the needed mask is
00000000000000000000000000010000
This happens to be the hex integer 0x00000010, but we dont need to know that, because we can construct the mask with the left-shift operator:
unsigned int mask = 1u << 4;
unsigned int n = 100;
bool status = n & mask;
cout << status << endl; // 0
Since the number 100 has the bit representation 0000000000000000000000001100100, bit-4 is a zero, so a 0 is printed. If you wanted bit-5 instead, n & (1u << 5) would return 32 (= 100000), which, converted to a bool, prints 1. If you want to use the result as an integer (not a bool) containing either a 1 or a 0, you need to use the double-bang idiom:
unsigned int mask = 1u << 5;
unsigned int n = 100;
int status = !!(n & mask);
cout << status << endl; // 1
The value for status is !!(32) = !(0) = 1. The logical-not operator (!) converts a non-zero to zero and a 0 to 1.
To set a bit, OR the mask with the number:
mask = 1u << 4;
n |= mask; // Set bit-4
status = n & mask;
cout << status << endl; // 1
To reset (i.e., set to 0) a bit, AND the number with a mask that has a 0 in the position of interest and 1s everywhere else:
n &= ~mask;
status = n & mask;
cout << status << endl; // 0
To flip a bit, XOR the 1-mask with it:
n ^= mask;
status = n & mask;
cout << status << endl; // 1
n ^= mask;
status = n & mask;
cout << status << endl; // 0
To determine the value in a range of m bits, construct a mask with m 1s in the m least-significant bits and zeroes everywhere else. Then shift the number to the right so the rightmost bit of the range occupies bit-0, and AND it with the mask. For example, the number 6.5f, being a 32-bit IEEE floating-point number, has the following bit layout:
0 10000001 10100000000000000000000
(Spaces have been added to indicate the components.) The first bit is the sign bit. The next eight bits are the exponent offset by 127, which in this case is 129, since the exponent of 6.5f in binary is 2:
6.5 = 1.101 x 22
Lets extract the exponent and see if we get 129.
float x = 6.5f;
n = *reinterpret_cast(&x);
mask = (1u << 8) - 1;
unsigned int exp = (n >> 23) & mask;
cout << exp << endl; // 129
The expression (1u << 8) gives 100000000. Subtracting 1 gives 11111111, the eight ones we needed for the mask.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
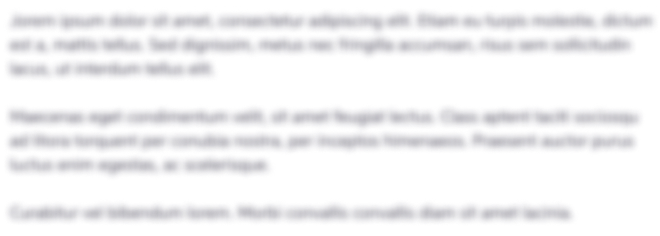
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started