Question
write a code that: 1) Add an entry at the end of the list 2) Add an entry at a specified position in the list
write a code that:
1) Add an entry at the end of the list
2) Add an entry at a specified position in the list
3) Remove an entry from the list
4) Show the contents of a specified list position
5) Show the hobby for a specified individual
6) Show the number of entries in the list, its current maximum size, and its contents
7) Exit the program
you will need:
public interface ListInterface {
public boolean add(Object newEntry);
//adds a new entry to the end of the list
public boolean add(int newPosition, Object newEntry);
//adds a new entry at a specified position within the list
public Object remove(int givenPosition);
//removes the entry at a given position from the list
public void clear();
//removes all entries from the list
public boolean replace(int givenPosition, Object newEntry);
//replaces the entry at a given position in the list
public Object getEntry(int givenPosition);
//retrieves the entry at a given position in the list
public boolean contains(Object anEntry);
//determines whether the list contains a given entry
public int getLength();
//returns the length of the list
public boolean isEmpty();
//determines if the list is empty
public boolean isFull();
//determine if the list is full
public void display();
//display all entries that are in the list
}
public class DynamicArrayList implements ListInterface {
private Object[] entry;
private int length;
private static final int INITIAL_SIZE = 50;
//*****************************************************************************
public DynamicArrayList() {
length = 0;
entry = new Object[INITIAL_SIZE];
}
//*****************************************************************************
public DynamicArrayList(int initialSize) {
length = 0;
entry = new Object[initialSize];
}
//*****************************************************************************
public boolean add(Object newEntry) {
if (isArrayFull())
doubleArray();
entry[length] = newEntry;
length++;
return true;
}
//*****************************************************************************
public boolean add(int newPosition, Object newEntry) {
boolean isSuccessful = true;
if ((newPosition >= 1) && (newPosition <= length+1)) {
if (isArrayFull())
doubleArray();
makeRoom(newPosition);
entry[newPosition-1] = newEntry;
length++;
}
else
isSuccessful = false;
return isSuccessful;
}
//*****************************************************************************
public Object remove(int givenPosition) {
Object result = null;
if ((givenPosition >= 1) && (givenPosition <= length)) {
result = entry[givenPosition-1];
if (givenPosition < length)
removeGap(givenPosition);
length--;
}
return result;
}
//*****************************************************************************
public void clear() {
length = 0;
}
//*****************************************************************************
public boolean replace(int givenPosition, Object newEntry) {
boolean isSuccessful = true;
if ((givenPosition >= 1) && (givenPosition <= length))
entry[givenPosition-1] = newEntry;
else
isSuccessful = false;
return isSuccessful;
}
//*****************************************************************************
public Object getEntry(int givenPosition) {
Object result = null;
if ((givenPosition >= 1) && (givenPosition <= length))
result = entry[givenPosition-1];
return result;
}
//*****************************************************************************
public boolean contains(Object anEntry) {
boolean found = false;
for (int index = 0; !found && (index < length); index++) {
if (anEntry.equals(entry[index]))
found = true;
}
return found;
}
//*****************************************************************************
public int getLength() {
return length;
}
//*****************************************************************************
public boolean isEmpty() {
return length == 0;
}
//*****************************************************************************
public boolean isFull() {
return false;
}
//*****************************************************************************
public void display() {
for (int index = 0; index < length; index++)
System.out.println(entry[index]);
}
//*****************************************************************************
private boolean isArrayFull() {
return length == entry.length;
}
//*****************************************************************************
private void doubleArray() {
Object[] oldList = entry;
int oldSize = oldList.length;
entry = new Object[2*oldSize];
for (int index = 0; index < oldSize; index++)
entry[index] = oldList[index];
}
//*****************************************************************************
private void makeRoom(int newPosition) {
for (int index = length; index >= newPosition; index--)
entry[index] = entry[index-1];
}
//*****************************************************************************
private void removeGap(int givenPosition) {
for (int index = givenPosition; index < length; index++)
entry[index-1] = entry[index];
}
//*****************************************************************************
} // end DynamicArrayList
public String getKeyboardInput(String prompt) { String inputString=""; System.out.println(prompt); try { InputStreamReader reader=new InputStreamReader(System.in); BufferedReader buffer=new BufferedReader(reader); inputString=buffer.readLine(); } catch (Exception e) {} return inputString; }
public char getCharacter(boolean validateInput, char defaultResult, String validEntries, int caseConversionMode, String prompt) { if (validateInput) { if (caseConversionMode == 1) { validEntries = validEntries.toUpperCase(); defaultResult = Character.toUpperCase(defaultResult); } else if (caseConversionMode == 2) { validEntries = validEntries.toLowerCase(); defaultResult = Character.toLowerCase(defaultResult); } if ((validEntries.indexOf(defaultResult) < 0)) //if default not in validEntries validEntries = (new Character(defaultResult)).toString() + validEntries;//then add it } String inputString=""; char result = defaultResult; boolean entryAccepted = false; while (!entryAccepted) { result = defaultResult; entryAccepted = true; inputString = getKeyboardInput(prompt); if (inputString.length() > 0) { result = (inputString.charAt(0)); if (caseConversionMode == 1) result = Character.toUpperCase(result); else if (caseConversionMode == 2) result = Character.toLowerCase(result); } if (validateInput) if (validEntries.indexOf(result) < 0) { entryAccepted = false; System.out.println("Invalid entry. Select an entry from the characters shown in brackets: [" + validEntries + "]"); } } return result;
public int getInteger(boolean validateInput, int defaultResult, int minAllowableResult, int maxAllowableResult, String prompt) { String inputString = ""; int result = defaultResult; boolean entryAccepted = false; while (!entryAccepted) { result = defaultResult; entryAccepted = true; inputString = getKeyboardInput(prompt); if (inputString.length() > 0) { try { result = Integer.parseInt(inputString); } catch (Exception e) { entryAccepted = false; System.out.println("Invalid entry..."); } } if (entryAccepted && validateInput) { if ((result != defaultResult) && ((result < minAllowableResult) || (result > maxAllowableResult))) { entryAccepted = false; System.out.println("Invalid entry. Allowable range is " + minAllowableResult + "..." + maxAllowableResult + " (default = " + defaultResult + ")."); } } } return result; }
public long getLong(boolean validateInput, long defaultResult, long minAllowableResult, long maxAllowableResult, String prompt) { String inputString = ""; long result = defaultResult; boolean entryAccepted = false; while (!entryAccepted) { result = defaultResult; entryAccepted = true; inputString = getKeyboardInput(prompt); if (inputString.length() > 0) { try { result = Long.parseLong(inputString); } catch (Exception e) { entryAccepted = false; System.out.println("Invalid entry..."); } } if (entryAccepted && validateInput) { if ((result != defaultResult) && ((result < minAllowableResult) || (result > maxAllowableResult))) { entryAccepted = false; System.out.println("Invalid entry. Allowable range is " + minAllowableResult + "..." + maxAllowableResult + " (default = " + defaultResult + ")."); } } } return result; }
public double getDouble(boolean validateInput, double defaultResult, double minAllowableResult, double maxAllowableResult, String prompt) { String inputString = ""; double result = defaultResult; boolean entryAccepted = false; while (!entryAccepted) { result = defaultResult; entryAccepted = true; inputString = getKeyboardInput(prompt); if (inputString.length() > 0) { try { result = Double.parseDouble(inputString); } catch (Exception e) { entryAccepted = false; System.out.println("Invalid entry..."); } } if (entryAccepted && validateInput) { if ((result != defaultResult) && ((result < minAllowableResult) || (result > maxAllowableResult))) { entryAccepted = false; System.out.println("Invalid entry. Allowable range is " + minAllowableResult + "..." + maxAllowableResult + " (default = " + defaultResult + ")."); } } } return result; }
public String getString(String defaultResult, String prompt) { String result = getKeyboardInput(prompt); if (result.length() == 0) result = defaultResult; return result; }
To call back on for the program to run
Step by Step Solution
There are 3 Steps involved in it
Step: 1
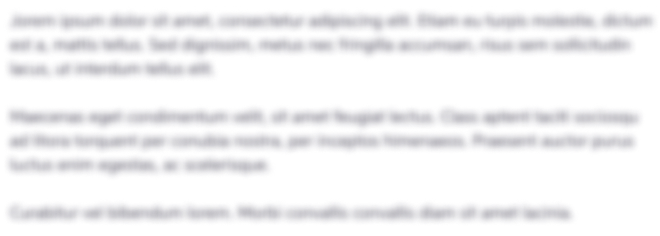
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started