Question
Write a code that respects the code standards of the C++ programming language regarding: Variables and functions names Space use Use of empty lines, Indentation
Write a code that respects the code standards of the C++ programming language regarding: Variables and functions names Space use Use of empty lines, Indentation . Your project is assessed based on the rubrics that you are encourage to download from the Moodle course page and keep it in mind while developing your code. In the .h file define a user-defined type Dyn2DArray that is a pointer to a dynamic 2D array of integer. This user-defined type must be used every time you have to deal with a dynamic 2D array of integer in side the code.
Define a Class Sudoku that has the following data members: 1. gridDimension: this integer data member represents the dimension of the square 2D array that is the initial grid of the sudoku game. 2. initialGrid: this pointer to a dynamic 2D array that represents the initial grid of the sudoku game. It must be generated by the constructor. 3. nbrOfErrors: this int data member represents the number of occurred errors committed by the player and initialize it to zero. An error occurs when the player adds a value that already exists in the same row, or same column or in the same block. When the player commits more errors than the number of allowed errors (nbrOfAllowedErrors), the game ends with failure. 4. nbrOfAllowedErrors: this int data member represents the number of errors allowed per game. We suggest to initialize it to 5. 5. emptyElementsArray: a pointer to a 2D array with elements having the value 0 if their corresponding elements are to be input by the user and the value 1 if their values are set already. The dynamic array pointed to by this pointer must be generated by the member function generateEmptyElementsArray. 6. nbrOfMissedDigits: this integer data member represents the number of digits to be guessed by the player. When its value becomes 0, the player wins the game. This variable is assigned by the member function countMissedValues. Member functions (for all these methods, row and col are between 0 and 8) 1. Define the constructor Sudoku that takes a pointer to a 2D array of integer that represents the initial grid of the sudoku game and its dimension. The function must check that the received dimension is a square number, otherwise the execution of the program must stop. 2. The private member function void displaySudoku() that displays the filled elements of initialGrid in a tabular format. Empty elements should be replaced by spaces. The output, of initial grid, given by this method should be the same as follows: col1 col2 col3 col4 col5 col6 col7 col8 col9 Row1 9 3 5 6 7 4 Row2 5 4 1 7 8 6 Row3 6 8 7 2 5 1 Row4 5 9 2 1 7 Row5 7 2 8 1 9 4 3 Row6 9 7 3 6 2 Row7 1 2 3 4 8 Row8 4 9 6 1 Row9 5 3 8 2 9 6 7 3. Define the private member function Dyn2DArray generateEmptyElementsArray() that generates and returns a dynamic 2D array has the elements equal to 1 if tis corresponding elements in the data member initialGrid is different from 0, otherwise equal to 0. Assume that the parameters values satisfy the constraints of values of Sudoku game: all values are between 0 and 9 and there is no repeated value in rows, columns and blocks. 4. Define the private member function int countMissedValues() that returns the number of the 0 values in the data member initialGrid. In fact, the returned value represents the number of values that must be guessed by the player. 5. Define the private member function int addValueInACell(int num, int row, int col) that receives a value num to be added and the coordinates row and col of the cell of initialGrid in which the value is to be stored. Assume that the received coordinates row and col are valid (between 0 and 8 and the value in the element with the same coordinates in the emptyElementsArray is 0. These tests must be done in the runGame member function. The member function addValueInACell calls the member functions existInRow, existInCol and existInBlock that check the unicity of this value in the related row and the related column and in the related block (3 3). If the value num doesnt exist in the same row and the same column and the same block, it is stored in the cell initialGrid [row][col], otherwise no change occurs in the array initialGrid. The returned value is: 0: if the value num is stored in a cell that wasnt assigned yet by the user. 1: if the value num is stored in a cell that was previously assigned by the user and that the user wants to change its value. 2: if the value num exists already in another cell of the same row. 3: if the value num exists already in another cell of the same column. 4: if the value num exists already in another cell of the same block. 6. Define the private member function boolean existInRow(int num, int row) that checks if its parameter num exists or not in the row row of the data member initialGrid. 7. Define the private member function boolean existInCol(int num, int col) that checks if its parameter num exists or not in the column col of the data member initialGrid. 8. Define the private member function boolean existInBlock(int num, int row, int col) that checks if its parameter num exists or not in the block related to the parameters row and col. This member function must calculate internally the coordinates of the upper-left corner of the block to witch the element belongs. (Hint: use the operator modulo %). 9. Define the public member function runGame that should do the following: Variables: 1. theValue: declare this variable of type int to read in it the value to be added to the grid. 2. rowNumber: declare this int variable that represents the row number entered by the user. The row number must be between 1 and 9. 3. colNumber: declare this int variable that represents the column number entered by the user. The column number must be between 1 and 9. 4. resultOfAddValueInACell: declare this int variable to store the return value of the method addValueInACell. After declaring the above mentioned variables, the member function runGame must: 1. call the method generateEmptyElementsArray and assign the reference emptyElementsArray. 2. call the method countMissedValues and assign the variable nbrOfMissingDigits. 3. display the array initialGrid, 4. in a loop, prompt the user to 4.1. enter the row number until a valid value between 1 and 9 is entered. 4.2. enter the column number until a valid value between 1 and 9 is entered. 4.3. check that the cell is not already assigned in the initial grid. If it is, repeat steps 4.1 and 4.2 must be repeated until valid values are entered. 4.4. enter the value to be added to the grid until a valid one between 1 and 9 is entered. 4.5. use the entered values to call the member function addValueInACell 4.6. act according the returned value of the member function addValueInACell to either update the variable(s) that need to be updated or display an appropriate message that shows the user his error (same value in the same row or column or block) and update the variable(s) that need to be updated. 4.7. test if the number of committed errors is greater than the number of allowed errors, or all the missed values are added in the right way and display the appropriate message.
The class Sudoku must be tested in a tester as follows: 1. Open the file named sudokudata.txt and read the first integer from it. 2. Check that the read value is a square number 2.1. If its a square, get its square root and Allocate a dynamic 2D array that has the calculated square root as dimensions. 2.2. If not, end the program 3. Read the remaining data from the file and assign the read values to its elements row by row. 4. Use this dynamic array and its dimension as argument to instantiate an object Sudoku named mySudoku. 5. Call the public member function runGame using the object mySudoku. 6. Finish the program by thanking the player for using it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
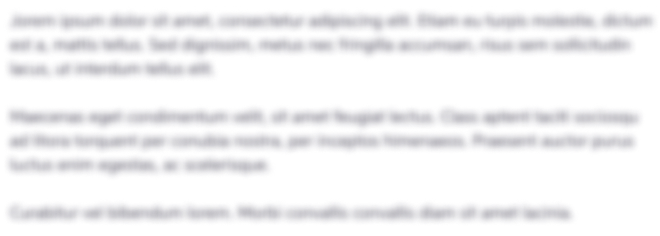
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started