Question
Write a complete Java program in a file called Module1Program.java that reads all the lyrics from a file named lyrics.txt that is to be found
Write a complete Java program in a file called Module1Program.java that reads all the lyrics from a file named lyrics.txt that is to be found in the same directory as the running program. The program should read the lyrics for each line and treat each word as a token. Embedded in each line will be the line number for that line. The number may occur anywhere within the line and each line is guaranteed to have one number in it.
Your job is to read each line, remove the integer within the line and put the integer into one array. For all the words that occur in the line, put them into a separate String array. After reading all the lines, use the Selection Sort algorithm that sorts the two arrays in parallel. After sorting all the lines, display the lyrics in order corresponding to the number embedded within each line. The output should have the first character of the first word at the beginning of the line. The output should contain one space between each word. A word is a continuation of characters until it reaches a space.
Vivir mi 6 vida, la la la la Voy a reir, voy a 3 gozar A veces llega 9 la lluvia Voy a reir, 1 voy a bailar 10 Para limpiar las heridas Vivir mi vida, 2 la la la la Voy a reir, 7 voy a gozar Vivir mi vida, 8 la la la la 4 Vivir mi vida, la la la la Voy 5 a reir (Ay eso!), voy a bailar
Then your program should print to the screen the following:
Voy a reir, voy a bailar Vivir mi vida, la la la la Voy a reir, voy a gozar Vivir mi vida, la la la la Voy a reir (Ay eso!), voy a bailar Vivir mi vida, la la la la Voy a reir, voy a gozar Vivir mi vida, la la la la A veces llega la lluvia Para limpiar las heridas
- If the file does not exist, then your program should instead display, File not found and exit.
- If the lyrics.txt contains more than 200 lines, it should display, The song is too long and exit.
- If the lyrics file is empty, the program should display, Empty file and exit.
A little starter code:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; /** * Program that reads lyrics from file lyrics.txt and prints the lyrics * in order. * * @author Your Name * @version date of submission */ public class Module1Program { public static final int MAX_LINES = 200; // parallel swap is provided for you public static void swap(int[] list, String[] lines, int i, int j) { int temp = list[i]; String tempStr = lines[i]; list[i] = list[j]; lines[i] = lines[j]; list[j] = temp; lines[j] = tempStr; } // Modify the array to do parallel sort. // post: array is in sorted (nondecreasing) order public static void selectionSort(int[] a) { for (int i = 0; i < a.length - 1; i++) { // find index of smallest element int smallest = i; for (int j = i + 1; j < a.length; j++) { if (a[j] < a[smallest]) { smallest = j; } } swap(a, i, smallest); // swap smallest to front } } public static void main(String[] args) { File theSong = new File("lyrics.txt"); } }
Testing: You should test your program in a variety of situations missing file, empty file, file too long.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
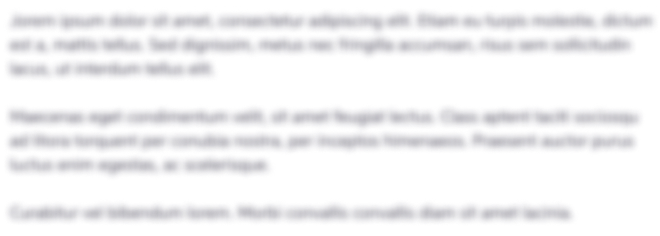
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started