Question
Write a complete Java program that creates the following classes: class PizzaOrder which implements the Comparable interface and contains the following attributes and member methods:
Write a complete Java program that creates the following classes:
class PizzaOrder which implements the Comparable interface and contains the following attributes and member methods:
customerName ( String )
dateOrdered ( Date )
pizzaSize (final static int SMALL=1, MEDIUM=2, LARGE=3)
numberOfToppings ( int )
toppingPrice ( double )
Appropriate constructors ( default and non default ) as well as the appropriate setter and getter methods.
A toString( ) method.
Method calculateOrderPrice() which calculates the price of the pizza order as follows:
( numberOfToppings * toppingPrice ) * pizzaSize
Method printOrderInfo () which prints only the customers name and the calculated order price on one line to the screen.
class Delivery which extends PizzaOrder and contains the following attribute and methods:
tripRate ( double )
zone ( int 1-4)
Appropriate constructors ( default and non default ) as well as the appropriate setter and getter methods.
toString() method that overrides the method in PizzaOrder.
A calculateOrderPrice() method which overrides the method in PizzaOrder and adds the ( tripRate/100 * totalprice * zone ) to the price.
class ToGo which extends PizzaOrder.
Class Seated which extends PizzaOrder and contains the following attribute and methods:
serviceCharge ( double )
numberOfPeople ( int )
toString() method that overrides the method in PizzaOrder.
A calculateOrderPrice() method which overrides the method in PizzaOrder and adds the ( serviceCharge * numberOfPeople ) to the price.
A Driver class which includes the following methods:main method that does the following:
creates an ArrayList called orders of type PizzaOrder and adds five different orders to it ( two Delivery, one ToGo, and two Seated ).
You must NOT ask the user to enter the attributes but you should fill them up directly in the main method. MAKE SURE YOUR CONSTRUCTORS FOLLOW THE SAME ORDER OF ATTRIBUTES AS SPECIFIED ABOVE AS FOLLOWS:
PizzaOrder (customerName, pizzaSize, numberOfToppings, toppingPrice)
Delivery (customerName, pizzaSize, numberOfToppings, toppingPrice, tripRate, zone)
ToGo (customerName, pizzaSize, numberOfToppings, toppingPrice)
Seated (customerName, pizzaSize, numberOfToppings, toppingPrice, serviceCharge, numberOfPeople)
Asking the user to enter the attributes or not following the constructor order as above will result in your assignment not getting graded and you will receive a zero grade.
Sorts the orders based on their calculated order price.
Prints the sorted orders customer names and prices.
Prints the total sum of all order prices.
Prints a report ( all properties and order price ) for the second Delivery order ( orders.get(1) ).
Method sortOrders which takes an ArrayList of type PizzaOrder as an argument and sorts it. You may use the java.util.Collections.sort method to do the actual sorting.
Method calculateTotalOrdersPrice which takes an ArrayList of type PizzaOrder as an argument and returns the total price of all the orders in that ArrayList.
Please note the Following:
Your program should be well commented based on Java formal documentation.
What you need to turn in:
Your project folder ( containing all your project .java files) should be compressed (.rar) and saved as proj_I_youridnumber_yourLabsectionnumber.rar ( for example if your student id number is 1211234 and your lab section is section 9 then the project folder should be called proj_I_1211234_s9.rar ). Turn in your project by replying to the course coordinators message on Ritaj and attaching your code .rar file (proj_I_youridnumber_yourLabsection.rar).
then For this assignment, you will use the same classes you created in Project phase I ( PizzaOrder, Delivery, ToGo, and Seated) and build a javafx GUI to provide input and output as follows:
Your Driver class should first build and display a GUI containing the following items:
customerName ( String ) default empty
orderType (ToGo, Delivery, or Seated) default ToGo
pizzaSize (int 1 = SMALL, 2=MEDIUM, and 3 = LARGE) default 1 (SMALL)
List of Toppings ( at least three: Onions, Olives, and Green Peppers ) default none
toppingPrice ( double ) - default (10)
orderPrice ( double ) default (0.0)
You are free to design your interface, but you need to at least use ONE OF EACH of the following ( you may use more that one of each if you like):
Label
Button
CheckBox
RadioButton
ComboBox
TextField
The default GUI displayed should NOT have any Labels or Text fields for tripRate (double), zone (int), ServiceCharge (double), nor numberOfPeople (int). As soon as the user selects a pizza order of type Delivery, a tripRate label and text field as well as a zone label and text field should appear appropriately and if he/she selects a pizza order of type Seated then the tripRate and zone Labels and Textfields should disappear and get replaced by a serviceCharge and numberOfPeople Labels and Textfields appropriately. When the user selects a ToGo pizza order then tripRate + zone OR serviceCharge + numberOfPeople labels and textfields should disappear from the GUI.
Your GUI should include three buttons at the bottom labeled ProcessOrder, PrintOrders, and Reset respectively.
After the user fills the form and presses the button ProcessOrder your program should do the following:
Your program should create an appropriate object based on the pizza order type selected ( ToGo, Delivery, or Seated) using the data provided by the user in the GUI.
Add the created pizza order object to an ArrayList of type PizzaOrder called orders.
Use the created object to calculate the orderPrice and display it to the GUI.
You do NOT need to check for valid text field inputs (double, int, ). You may assume that the user enters valid data for each of the text fields.
Pressing the button labeled PrintOrders should sort and print a list of customer names and order prices for orders saved in ArrayList orders to a separate stage ( different from the original GUI ).
Pressing the Reset button should reset ALL items and fields to their default values as specified above as well as remove all the orders from the ArrayList orders.
Please note the Following:
Your program should be well commented based on Java formal documentation.
What you need to turn in:
Your project folder ( containing all your project files) should be compressed (.rar) and saved as proj_II_youridnumber_yourLabsectionnumber.rar ( for example if your student id number is 1211234 and your lab section is section 9 then the project folder should be called proj_II_1211234_s9.rar ). Turn in your project by replying to the course coordinators message on Ritaj and attaching your code .rar file (proj_II_youridnumber_yourLabsection.rar).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
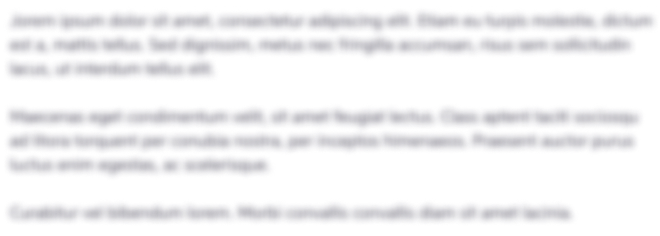
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started