Question
Write a Java application using class to simulate a Coffee Dispenser Machine. Your application should be able to create a coffee dispenser machine object to
Write a Java application using class to simulate a Coffee Dispenser Machine. Your application should be able to create a coffee dispenser machine object to keep track of the number of cups of coffee served from the machine and other operations related to it.
You will start with creating a class called CoffeeMachine to represent a coffee dispenser machine that may be located at a particular premise. A CoffeeMachine object should have the following fields:
machineNo (a unique integer value representing the coffee dispenser machine number)
location (a string representing the location of the machine)
coffeePowderAmt (the total amount of coffee powder available in the machine in unit gram)
amtPerServing (the amount of coffee powder required to make a cup of coffee measured in gram)
numOfServing (the total number of cups of coffee that have been purchased from the machine)
pricePerServing (the price for a cup of coffee sold from the machine)
under University Policy Directory and in Faculty handbooks and subject guides.under University Policy Directory and in Faculty handbooks and subject guides.
The class should have the following methods:
a default constructor that will initialize a CoffeeMachine object with 9999 for machineNo, "None" for location, 0.0 for coffeePowderAmt, amtPerServing, and pricePerServing, and 0 for numOfServing.
mutator methods to set the values each attributes individually.
accessor methods to retrieve each attributes.
a method called dispenseCoffee() that will receive the number of cups of coffee to be dispensed, update the CoffeeMachinestate by reducing the coffeePowderAmt, and adding the numOfServing, and display the message to indicate how many cups of coffee have been successfully dispensed. If the CoffeeMachine does not have enough coffee powder to make the required coffee, it should display a message indicating the state and request that the coffee powder to be replenished.
a method called getTotalServings() that will return the total number of cups of coffee that can still be purchased from the machine. a method called addCoffeePowder() that will receive the amount (in grams) of coffee powder to be added to the machine.
a method called totalSales() to calculate and return the sales made by the machine by multiplying the numOfServing with pricePerServing.
Next, write a class called CoffeeMachineApp that will have a main() method to perform several operations for a CoffeeMachine object. When the application starts, create a CoffeeMachine object, request the user to enter the initial details of a coffee machine, and then display a menu for the user to choose from. A sample menu is shown below:
Coffee Dispensing Machine
#5372 Location: Subang Parade ---------------------------------
1 - Dispense Coffee
2 - Show Coffee Machine Details
3 - Show Total Servings Available
4 - Show Current Total Sales
5 - Replenish Coffee
6 - Reset Machine
0 - Quit
Enter your option:
The option to show coffee machine details should display the coffee machine's number, location, amount of coffee powder, the amount per serving, the number of servings that have been purchased, and price per servings. The option to replenish coffee will add coffee powder to the machine, and the option to reset the machine will let the user to change all the values of the attributes.
Allow the user to repeatedly perform various operations until the user chooses to quit from the application.
Make sure you include necessary validations in your CoffeeMachine class and main() method. Use meaningful comments and identifiers in your code. Avoid repetitive codes as much as possible and make your program readable. You should also be creative in presenting your output. You may display your output on the console or use JOptionPane dialog boxes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
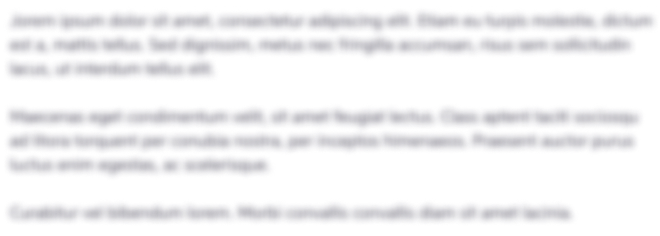
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started