Question
Write a Java application with a class name of Payroll, for the Travel Agency, that willCalculate the weekly paycheck for both Salaried and Hourly employees.
Write a Java application with a class name of Payroll, for the
Travel Agency, that willCalculate the weekly paycheck for both Salaried and Hourly employees. Salaried employees will be paid 1/52 of their annual pay minus 18% withheld for federal and state taxes, as well as 4% for retirement pension.
Salaried employees do not collect overtime pay.
There are two types of Hourly employees; permanent employees and temporary weekly employees.
Permanent weekly employees will be paid their hourly rate minus 18% withheld for federal and state taxes.
Temporary weekly employees will be paid their hourly rate and are Tax Exempt.
Hourly employees are paid time and a half if an employee works more than 40hours per week.
Assignment Task(s):
***********DO NOT USE CHAR TYPE************
You must define an enumerated type specifying the different employee types. You must define an enumerated type of EMPLOYEE_TYPE that defines the different types; Salaried, Hourly, Temporary, Quit and Unknown.
You must define a MAXIMUM_EMPLOYEE constant and set it to 4
. You must define a static method called getEmployeeType which accepts the user string input and returns the enumerated employee type.
Your application must implement a while loop to determine if the program should continue to prompt for employee information or Quit, based on the employee type entered.
The while loop must also exit automatically after the maximum number of employees have been entered.
After the employee name has been entered, you Must use a switch statement to determine the next course of action dependent on the employee type
. Your switch statement will use the enumerated employee type as the controlling variable.
Depending on the employee type, you will prompt the user for either their yearly or hourly salary. Your application will calculate the weekly gross salary, any deductions and the weekly net salary.
Your switch statement for each employee type should only prompt for the information it needs to calculate the appropriate wages, taxes and pension (if applicable).
The output will consist of the employees name, their weekly gross pay, any deductions and their weekly net pay. If the employee is a Temporary employee, the string No Taxes Deducted should appear in the weekly net pay column.
program must have the following
Properly defining enumerated employee type
Properly defining MAXIMUM_EMPLOYEE constant, setting to 5
Properly defining static getEmployeeType method, providing a String parameter and returning the proper enumerated type
Implementation of while loop to control properly exiting the application when quit is entered or when the maximum number of 5 employees has been entered.
Implementation of switch statement for properly prompting, reading and displaying appropriate employee information
Proper implementation of Salaried employee
Proper implementation of Hourly employee
Proper implementation of Temporary employee
please follow sample run
sample run:
EnterEmployee Type [Salaried], [Hourly], [Temporary] or [Quit]: salaried
Enter Employee First Name: Robert
Enter Employee Last Name : Mitchum
Robert Mitchum's Yearly Salary: $63500
Employee : Robert Mitchum
Gross Wages : $1221.15
Taxes Withheld : $219.81
Retirement Withheld : $48.85
Net Wages : $952.50
Enter Employee Type [Salaried], [Hourly], [Temporary] or [Quit]: Hourly
Enter Employee First Name: Danny
Enter Employee Last Name : Kaye
Danny Kaye's Hourly Salary: $10.50
Enter Danny Kaye's Number of Hours: 50
Employee : Danny Kaye
Gross Wages : $577.50
Taxes Withheld : $103.95
Net Wages : $473.55
Enter Employee Type [Salaried], [Hourly], [Temporary] or [Quit]: temporary
Enter Employee First Name: Cornelius
Enter Employee Last Name : Ryan
Cornelius Ryan's Hourly Salary: $8.50
Enter Cornelius Ryan's Number of Hours: 46
Employee : Cornelius Ryan
Gross Wages : $416.50
NO Taxes Deducted
Enter Employee Type [Salaried], [Hourly], [Temporary] or [Quit]: sal
Unknown Employee Type, Please Reenter: quit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
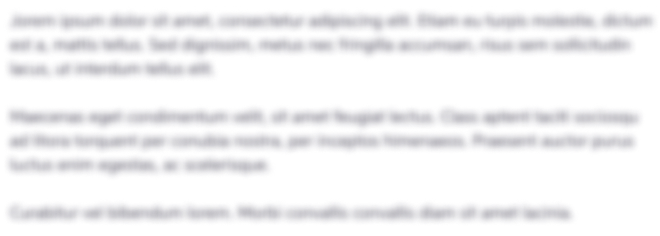
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started