Question
Write a Java class called Song to represent a song in a music library. The instance variables of the Song class are String objects named
Write a Java class called Song to represent a song in a music library. The instance variables of the Song class are String objects named title, artist, and album. These instance variables are to have private access modifiers. You will need to write the appropriate getter and setter methods to allow another class or object to access and modify the data values. Song should have a parameterized constructor which takes three string parameters: aTitle, anArtist, and anAlbum (in that order). In accordance with good programming practice, you are to override the equals and toString methods inherited from Object. The toString method should return in the following format: Title - Artist - Album. A Song is equal to another Song object if its title, artist, and album instance variables are identical. In addition, this class is going to be used in a Collection that is to be sorted, so it is to implement the Comparable interface. When comparing two song objects, they should be sorted lexicographically by Artist. If the Artists are the same, they should be sorted lexicographically by Album. If the Artists are the same, they should be sorted by Title. If the titles are the same, the Songs have the same ordering.
Write a Java class called MusicLibrary. MusicLibrary should have a single private instance variable named library of type ArrayList. MusicLibrary must implement the following methods:
A default constructor, which initializes the library instance variable.
addSong, which takes a single Song object and adds it to the library instance variable. addSong returns no value
removeSong, which takes a single Song object and removes it from the library instance variable. removeSong returns no value
listSongs, which takes no parameters and prints every song in the music library to the console. There is no required ordering for the outputted content. listSongs must use an Iterator object to iterate over the library instance variable. (The expected behavior can be accomplished without using an iterator, but no credit will be given to solutions which do not utilize iterator. Make sure to import Iterator from java.util and not some other location). listSongs returns no value.
listSortedSongs, which takes no parameters and prints every song in the music library to the console. The outputted content must be sorted lexicographcally from highest to lowest (e.g. from AZ). Do NOT sort the library instance variable. You should make a copy of library and sort that. (Dont reinvent the wheel here. Peruse the Java API for ArrayList for ideas) listSortedSongs returns no value.
When you have completed your implementation of both the Song and the MusicLibrary classes, use the provided test cases to test your work. When complete, submit your Song and the MusicLibrary classes to Blackbaord. I advise implementing and testing your Song class before starting your MusicLibrary class. The test cases for MusicLibrary will not pass if you do not have a working Song class.
List Lab Test Java
--------------------------
import static org.junit.Assert.*; import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.InputStream; import java.io.PrintStream; import java.lang.reflect.Field; import java.lang.reflect.Modifier; import java.util.ArrayList; import org.junit.After; import org.junit.Before; import org.junit.Test; public class ListLabTest { private final ByteArrayOutputStream outContent = new ByteArrayOutputStream(); private final ByteArrayOutputStream errContent = new ByteArrayOutputStream(); @Before public void setUpStreams() { System.setOut(new PrintStream(outContent)); System.setErr(new PrintStream(errContent)); } @Test public void SongInstanceVariablesTest() { Song testSong = new Song("Hurt Me Soul","Lupe Fiasco","Food & Liquor"); @SuppressWarnings("rawtypes") Class c = testSong.getClass(); try { c.getDeclaredField("title"); c.getDeclaredField("artist"); c.getDeclaredField("album"); assertEquals("You must make your instance variables private.",true,Modifier.isPrivate(c.getDeclaredField("title").getModifiers())); assertEquals("You must make your instance variables private.",true,Modifier.isPrivate(c.getDeclaredField("artist").getModifiers())); assertEquals("You must make your instance variables private.",true,Modifier.isPrivate(c.getDeclaredField("album").getModifiers())); } catch(NoSuchFieldException e) { fail("Could not find the " + e.getLocalizedMessage().toString() + " instance variable"); } catch(Exception e) { fail("Something weird went wrong"); } } @Test public void SongConstructorTest() { Song testSong = new Song("What Do I Do","A. Savage","Thawing Dawn"); @SuppressWarnings("rawtypes") Class c = testSong.getClass(); try { Field title = c.getDeclaredField("title"); title.setAccessible(true); String titleValue = (String) title.get(testSong); assertEquals("When checking the value of title we","What Do I Do",titleValue); Field artist = c.getDeclaredField("artist"); artist.setAccessible(true); String artistValue = (String) artist.get(testSong); assertEquals("When checking the value of title we","A. Savage",artistValue); Field album = c.getDeclaredField("album"); album.setAccessible(true); String albumValue = (String) album.get(testSong); assertEquals("When checking the value of title we","Thawing Dawn",albumValue); } catch (Exception e) { fail(e.toString()); } } @Test public void SongGettersTest() { Song testSong = new Song("In One Ear","Cage The Elephant","Cage the Elephant"); assertEquals("When checking the value of title we","In One Ear",testSong.getTitle()); assertEquals("When checking the value of artist we","Cage The Elephant",testSong.getArtist()); assertEquals("When checking the value of album we","Cage the Elephant",testSong.getAlbum()); } @Test public void SongSettersTest() { Song testSong = new Song("In One Ear","Cage The Elephant","Cage the Elephant"); testSong.setTitle("Birthday");testSong.setArtist("The Beatles");testSong.setAlbum("The Beatles (White Album)"); assertEquals("When checking the value of title we","Birthday",testSong.getTitle()); assertEquals("When checking the value of artist we","The Beatles",testSong.getArtist()); assertEquals("When checking the value of album we","The Beatles (White Album)",testSong.getAlbum()); } @Test public void SongToStringTest() { Song testSong = new Song("Distopian Dream Girl","Built to Spill","There's Nothing Wrong With Love"); assertEquals("When checking the return value of toString we","Distopian Dream Girl - Built to Spill - There's Nothing Wrong With Love",testSong.toString()); } @Test public void SongEqualsTest() { Song testSongOne = new Song("Clampdown","The Clash","London Calling"); Song testSongTwo = new Song("Walking Alone","Green Day","Nimrod"); Song testSongThree = new Song("Clampdown","The Clash","London Calling"); assertEquals("When checking the return value of equals given the same song object we",true,testSongOne.equals(testSongOne)); assertEquals("When checking the return value of equals given another song object with different values we",false,testSongOne.equals(testSongTwo)); assertEquals("When checking the return value of equals given another song object with the same values we",true,testSongOne.equals(testSongThree)); } @Test public void SongCompareToTest() { Song testSongOne = new Song("Frozen","Surfer Blood","Snowdonia"); Song testSongTwo = new Song("Jinx","Green Day","Nimrod"); Song testSongThree = new Song("Haushinka","Green Day","Nimrod"); Song testSongFour = new Song("Haushinka","Green Day","Nimrod"); Song testSongFive = new Song("St. Jimmy","Green Day","American Idiot"); //SurferBlood.GreenDay -1 assertEquals("When checking the return value of compareTo (comparing artist S to artist G) we",true,testSongOne.compareTo(testSongFive) <= -1); //GreenDay.SurferBlood 1 assertEquals("When checking the return value of compareTo (comparing artist G to artist S) we",true,testSongFive.compareTo(testSongOne) >= 1); //Nimrod.AmericanIdiot -1 assertEquals("When checking the return value of compareTo (matching artist, comparing album N to album A) we",true,testSongTwo.compareTo(testSongFive) <= -1); //AmericanIdiot.Nimrod 1 assertEquals("When checking the return value of compareTo (matching artist, comparing album A to album N) we",true,testSongFive.compareTo(testSongTwo) >= 1); //Jinx.Haushinka -1 assertEquals("When checking the return value of compareTo (matching artist & album, comparing title J to title H) we",true,testSongTwo.compareTo(testSongThree) <= -1); //Haushinka.Jinx 1 assertEquals("When checking the return value of compareTo (matching artist & album, comparing title H to title J) we",true,testSongThree.compareTo(testSongTwo) >= 1); //Haushinka.Haushinka 0 assertEquals("When checking the return value of compareTo (matching artist & album & title) we",0,testSongThree.compareTo(testSongFour)); } @Test public void MusicLibraryInstanceVariablesTest() { MusicLibrary testLibrary = new MusicLibrary(); @SuppressWarnings("rawtypes") Class c = testLibrary.getClass(); try { c.getDeclaredField("library"); assertEquals("You must make your instance variable private.",true,Modifier.isPrivate(c.getDeclaredField("library").getModifiers())); } catch(NoSuchFieldException e) { fail("Could not find the " + e.getLocalizedMessage().toString() + " instance variable"); } catch(Exception e) { fail("Something weird went wrong"); } } @Test public void MusicLibraryConstructorTest() { MusicLibrary testLibrary = new MusicLibrary(); @SuppressWarnings("rawtypes") Class c = testLibrary.getClass(); try { Field title = c.getDeclaredField("library"); title.setAccessible(true); @SuppressWarnings("unchecked") ArrayListlibraryValue = (ArrayList ) title.get(testLibrary); assertNotNull(libraryValue); assertEquals(0,libraryValue.size()); } catch (Exception e) { fail(e.toString()); } } @Test public void MusicLibraryAddSongTest() { MusicLibrary testLibrary = new MusicLibrary(); Song testSong = new Song("Sexy Sadie","The Beatles","The Beatles (White Album)"); testLibrary.addSong(testSong); @SuppressWarnings("rawtypes") Class c = testLibrary.getClass(); try { Field title = c.getDeclaredField("library"); title.setAccessible(true); @SuppressWarnings("unchecked") ArrayList libraryValue = (ArrayList ) title.get(testLibrary); //Once we have extracted the raw data ArrayList, validate the data assertTrue("We expected " + testSong.toString() + " to be the only song in the library ArrayList",libraryValue.get(0).equals(testSong)); } catch (Exception e) { fail(e.toString()); } } @Test @SuppressWarnings("unchecked") public void MusicLibraryRemoveSongTest() { MusicLibrary testLibrary = new MusicLibrary(); Song testSong = new Song("Vaporize","Broken Bells","Broken Bells"); @SuppressWarnings("rawtypes") Class c = testLibrary.getClass(); try { //Extract the data ArrayList from the Object Field title = c.getDeclaredField("library"); title.setAccessible(true); ArrayList libraryValue = (ArrayList ) title.get(testLibrary); //Add values directly to the ArrayList libraryValue.add(testSong); //Call the method we want to test testLibrary.removeSong(testSong); //Once we have extracted the raw data ArrayList, validate the data assertEquals(0,libraryValue.size()); } catch (Exception e) { fail(e.toString()); } } @Test //This test expects addSong to add songs at position 0 in the arrayList public void MusicLibraryListSongsTest() { MusicLibrary testLibrary = new MusicLibrary(); Song testSong = new Song("Sometime Around Midnight","The Airborne Toxic Event","The Airborne Toxic Event"); Song testSong1 = new Song("Dunes","Alabama Shakes","Sound & Color"); Song testSong2 = new Song("Staring Out The Window At Your Old Apartment","Jeff Rosenstock","WORRY"); Song testSong3 = new Song("We The People....","A Tribe Called Quest","We got it from Here... Thank You 4 Your service"); Song[] testSongs = {testSong,testSong1,testSong2,testSong3}; testLibrary.addSong(testSong); testLibrary.addSong(testSong1); testLibrary.addSong(testSong2); testLibrary.addSong(testSong3); String input = ""; InputStream in = new ByteArrayInputStream(input.getBytes()); System.setIn(in); testLibrary.listSongs(); String rawOutput = outContent.toString(); String[] outputLines = rawOutput.split(System.lineSeparator()); for(int i = 0; i < outputLines.length; i++) { assertEquals("At position " + i,testSongs[i].toString(),outputLines[i]); } } @Test public void MusicLibraryListSortedSongsTest() { MusicLibrary testLibrary = new MusicLibrary(); Song testSong = new Song("Point","The Districts","Popular Manipulations"); Song testSong1 = new Song("Everything Now","Arcade Fire","Everything Now"); Song testSong2 = new Song("Hard Feelings / Loveless","Lorde","Melodrama"); Song testSong3 = new Song("Tear It Down","Spoon","Hot Thoughts"); Song testSong4 = new Song("Los Ageless","St. Vincent","MASSEDUCTION"); Song testSong5 = new Song("Moonlight On The River","Mac DeMarco","This Old Dog"); Song testSong6 = new Song("American Dream","LCD Soundsystem","American Dream"); Song testSong7 = new Song("4:44","Jay-Z","4:44"); Song testSong8 = new Song("DNA.","Kendrick Lamar","DAMN"); Song testSong9 = new Song("Run For Cover","The Killers","Wonderful Wonderful"); Song testSong10 = new Song("Emotional Haircut","LCD Soundsystem","American Dream"); Song testSong11 = new Song("Jenny Was A Friend Of Mine","The Killers","Hot Fuss"); Song testSong12 = new Song("USA","Jeff Rosenstock","POST-"); Song testSong13 = new Song("Festival Song","Jeff Rosenstock","WORRY"); Song testSong14 = new Song("All This Useless Energy","Jeff Rosenstock","POST-"); Song testSong15 = new Song("Black Mirror","Arcade Fire","Neon Bible"); Song[] testSongs = {testSong1,testSong15,testSong7,testSong14,testSong12,testSong13,testSong8,testSong6, testSong10,testSong2,testSong5,testSong3,testSong4,testSong,testSong11,testSong9}; Song[] testSongsTwo = {testSong,testSong1,testSong2,testSong3,testSong4,testSong5,testSong6,testSong7,testSong8,testSong9,testSong10, testSong11,testSong12,testSong13,testSong14,testSong15}; testLibrary.addSong(testSong);testLibrary.addSong(testSong1);testLibrary.addSong(testSong2); testLibrary.addSong(testSong3);testLibrary.addSong(testSong4); testLibrary.addSong(testSong5);testLibrary.addSong(testSong6);testLibrary.addSong(testSong7); testLibrary.addSong(testSong8);testLibrary.addSong(testSong9);testLibrary.addSong(testSong10);testLibrary.addSong(testSong11); testLibrary.addSong(testSong12);testLibrary.addSong(testSong13);testLibrary.addSong(testSong14);testLibrary.addSong(testSong15); String input = ""; InputStream in = new ByteArrayInputStream(input.getBytes()); System.setIn(in); testLibrary.listSortedSongs(); String rawOutput = outContent.toString(); String[] outputLines = rawOutput.split(System.lineSeparator()); for(int i = 0; i < outputLines.length; i++) { assertEquals("At position " + i,testSongs[i].toString(),outputLines[i]); } @SuppressWarnings("rawtypes") Class c = testLibrary.getClass(); try { Field title = c.getDeclaredField("library"); title.setAccessible(true); @SuppressWarnings("unchecked") ArrayList libraryValue = (ArrayList ) title.get(testLibrary); //Once we have extracted the raw data ArrayList, validate the data boolean unchanged = true; for(int j = 0; j < libraryValue.size(); j++) { if(libraryValue.get(j).equals(testSongsTwo[j]) == false) { unchanged = false; } } assertTrue("We expected the library ArrayList to be unchanged after calling listSortedSongs",unchanged); } catch (Exception e) { fail(e.toString()); } } @After public void cleanUpStreams() { System.setOut(null); System.setErr(null); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
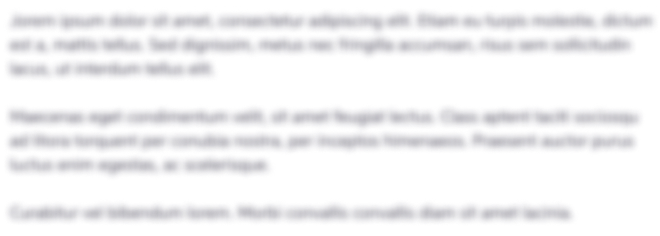
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started