Question
WRITE A JAVA PROGRAM: a text analyzer program that reads a text file and counts the number of lines, number of words (Kings counts as
WRITE A JAVA PROGRAM: a text analyzer program that reads a text file and counts the number of lines, number of words (Kings counts as one word) and the frequency of occurrence of each of the 26 letters of the alphabet.
Note:
- We ignore case: Treat upper and lowercase letters to be the same.
- Assume that there is at least one space between words on the same line.
- Only characters A-Z are to be counted.
For example, if the text file containing this text shown below:
TextAnalyzerData.txt
Baa, baa, black sheep Have you any wool? Yes sir, yes sir Three bags full. One for my master And one for the dame One for the little boy Who lives down the lane. |
The Analyzer should print the following information:
Number of Lines: 8 Number of Words: 34 Letter Counts Frequency of A: 12 Frequency of B: 5 Frequency of C: 1 Frequency of D: 3 Frequency of E: 18 Frequency of F: 4 Frequency of G: 1 Frequency of H: 7 Frequency of I: 4 Frequency of J: 0 Frequency of K: 1 Frequency of L: 8 Frequency of M: 3 Frequency of N: 7 Frequency of O: 12 Frequency of P: 1 Frequency of Q: 0 Frequency of R: 7 Frequency of S: 8 Frequency of T: 7 Frequency of U: 2 Frequency of V: 2 Frequency of W: 3 Frequency of X: 0 Frequency of Y: 6 Frequency of Z: 0
|
So how should you write this program?
To begin with, you need to write a Java class called TextAnalyzer. When thinking about writing this class, note that it must meet the following specifications:
- It should have the following instance variables (sometimes called attributes):
private int lineCount;
private int wordCount;
private int[] frequencies = new int[26];
- It should have get methods for each of the instance variables e.g., it should have this method:
public int getLineCount()
{
return lineCount;
}
Note how we used case in the get method name given the case we used to create the instance variable name i.e, the variable name was lineCount (using camel case as Java expects note that the first lower case l in the variable name), but the get methods name begins with get, and is written as getLineCount(), where the lower case l in the variable name gets transformed to an upper case L. You should use an identical style in all the get methods you write.
- It should have a method called: analyzeText(String fileName) method that
- Opens the specified file
- Reads the data line by line. After it reads each line, the method should update the value in variable: lineCount
- Splits the line just read into words. After this splitting, the method should count the number of words generated. How can it most easily do that? Once you figure this out, use this count to update the value in variable: wordCount
- Scans the line just read character by character and updates the frequency count for each character. Remember: Only alphabetical characters 'A' .. 'Z' are counted; lower-case letters are mapped to upper-case letters, and all other characters are ignored. Think: what is the best way to scan the line just read? Note that the line just read goes into a string, right? Scanning means looking at each character of the string containing the contents of the line. Which of the various string methods we discussed above is most suitable for looking at each character of the line?
- Now, after figuring out how you will look at each character of the line, you need to figure out how to accomplish the following: suppose the character you just accessed from the line is A or a. This means that in the frequencies array that you have declared in your instance variables, the value in frequencies[0]will be increased by 1. If the next character you accessed is a D or d, then the value in frequencies[3]will be increased by 1. Similarly, on reading Z or z, you will increase the value in frequencies[25]by 1. Think of how to do this? You could use an if or switch statement of course, but work with your partner, and think (google if necessary) to figure out how you can do this more efficiently.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
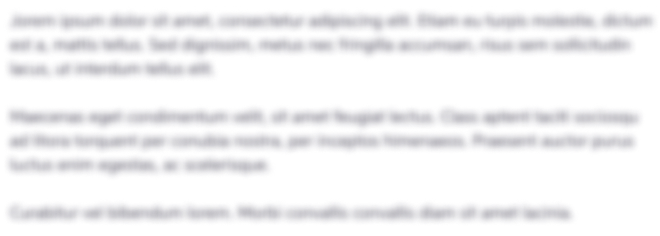
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started