Question
Write a Java program that constructs an Applet. The Applet (JApplet) of your program should contain a check box Filled, JRadioButtons to choose a color,
Write a Java program that constructs an Applet.
The Applet (JApplet) of your program should contain a check box "Filled", JRadioButtons to choose a color, and a drawing panel where a rectangle is drawn. A button JRadioButtons, and a JCheckBox are located at the top side of the applet. The background of the drawing panel is black and the initial color of the rectangle should be white.
(The size of the applet here is approximately 400 X 400)
A user can choose a color using a radio button. Until the color is changed, any rectangle will be drawn with that color. The default color is white.
A user can also check or uncheck the check box for "Filled". If it is filled, you will see a filled rectangle with a chosen color, and if it is un-checked, you should see an unfilled rectangle.
A user can move a mouse into the drawing area and press the mouse at the left, right, top, or bottom side of the rectangle, and drag the mouse to move and change the location of one side. Once the mouse is released, that will define the final location of the side that determines the new shape of the rectangle.
In the below picture, the mouse is dragging the right side of this rectangle towards right to make its width wider (it can be dragged to left too).
In the below, the mouse is dragging the bottom side of the rectangle to change its height.
Note that a user should not able to make the height or width of the rectangle smaller than 10 pixels. Also, the mouse pressed method can react to the location plus or minus 5 pixels (for instance, the left side is at x =100 pixels, then mouse pressed should be able to work in the x-range of [95,105]
-------------------------------------------------------------
Class description
WholePanel class
The WholePanel class organizes all components in the applet. It extends JPanel defined in javax.swing package. It should contain at least the following instance variable:
More components such as JRadioButton need to be added. This class should have a constructor:
public WholePanel()
This is where all components are arranged. Add as many instance variables as you like to this class, and initialize them in this constructor. One way to define this class is to contain an object of CanvasPanel, the check box "Fill" and JRadioButtons
CanvasPanel class (defined as a nested class of WholePanel class)
The CanvasPanel class extends JPanel defined in javax.swing package. This is where a rectangle is drawn. The Background of this panel is black. It must contain the following methods.
public CanvasPanel( )
The constructor of the class. It should initialize this panel including its background color and any listeners that it should be listening to.
public void paintComponent(Graphics page)
Using the parameter, the Graphics object, this method will draw a rectangle with a selected color. Remember that this method need to call paintComponent method defined in its parent class.
FillListener class (defined as a nested class of WholePanel class)
The FillListener class implements ItemListener interface defined in java.awt.event package. It must implement the following method:
public void itemStateChanged(ItemEvent event)
This method is executed in case the "Fill" check box is checked or unchecked. If it is checked, then the rectangle should be filled with a selected color, and if it is not, the rectangle should be unfilled.
ColorListener class (defined as a nested class of WholePanel class)
The ColorListener class implements ActionListener interface defined in java.awt.event package. It must implement the following method:
public void actionPerformed(ActionEvent event)
In this method, the color chosen by a user using JRadioButtons is assigned as a color to be used to draw a rectangle.
PointListner class (defined as a nested class of WholePanel class)
The PointListener class implements MouseMotionListener interface (it can also implement MouseListener). It must implement the following method:
public void mouseDragged(MouseEvent event)
This method should be written so that a user can press the mouse at the left, right, top, or bottom side of the rectangle, drag the mouse to move, and change the location of one side. Once the mouse is released, that will define the final location of the side that determines the new shape of the rectangle.
You can also define mousePressed and mouseReleased if it helps to achieve the goal.
Other methods from MouseListener and MouseMotionListener can be left as blank
--------------------------------------------------------------------------
Given Codes:
Assignment7.java
--------------------------------------------------------------------------
import javax.swing.*;
public class Assignment7 extends JApplet {
public void init() { // create a WholePanel object and add it to the applet WholePanel wholePanel = new WholePanel(); getContentPane().add(wholePanel);
//set applet size to 400 X 400 setSize (400, 400); }
}
----------------------------------------------------------------------------
WholePanel.java
-----------------------------------------------------------------------------
import java.awt.*; import javax.swing.*; import java.awt.event.*; // to use listener interfaces
public class WholePanel extends JPanel { private Color currentColor; private int currentWidth, currentHeight; private CanvasPanel canvas; private JPanel menuPanel; private JCheckBox fillCheck; private int x1, y1;
public WholePanel() { //white is the default color currentColor = Color.WHITE;
//default x-y cooridnate, width, and height of a rectangle currentWidth = currentHeight = 100; x1 = 100; y1 = 100;
fillCheck = new JCheckBox("Filled");
menuPanel = new JPanel(); menuPanel.add(fillCheck);
canvas = new CanvasPanel();
JSplitPane sPane = new JSplitPane(JSplitPane.VERTICAL_SPLIT, menuPanel, canvas);
setLayout(new BorderLayout()); add(sPane, BorderLayout.CENTER);
//to be completed
}
//insert ColorListener and FillListener classes
//CanvasPanel is the panel where pressed keys will be drawn private class CanvasPanel extends JPanel { //Constructor to initialize the canvas panel public CanvasPanel( ) { // make this canvas panel listen to mouse addMouseListener(new PointListener()); addMouseMotionListener(new PointListener());
setBackground(Color.BLACK); }
//this method draws all characters pressed by a user so far public void paintComponent(Graphics page) { super.paintComponent(page);
//set color, then draw a rectangle page.setColor(currentColor);
page.drawRect(x1, y1, currentWidth, currentHeight); }
// listener class that listens to the mouse public class PointListener implements MouseListener, MouseMotionListener { //in case that a user presses using a mouse, //record the point where it was pressed. public void mousePressed (MouseEvent event) {} public void mouseClicked (MouseEvent event) {} public void mouseReleased (MouseEvent event) {} public void mouseEntered (MouseEvent event) {} public void mouseExited (MouseEvent event) {} public void mouseMoved(MouseEvent event) {} public void mouseDragged(MouseEvent event) { //to be filled }
} // end of PointListener
} // end of Canvas Panel Class
} // end of Whole Panel Class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
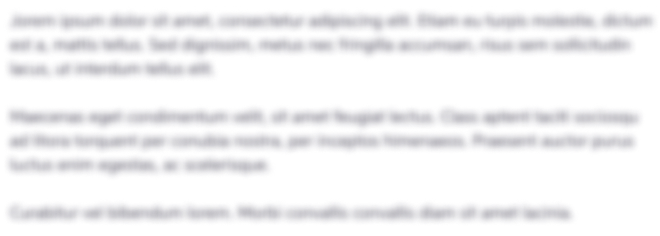
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started