Question
Write a Java program to load the flight database stored in a file called flightdb.txt , as exemplified in the following: In flightdb.txt: Air Canada,AC102,YVR,2019-03-04T06:45:00,YYZ,2019-03-04T14:12:00
Write a Java program to load the flight database stored in a file called flightdb.txt, as exemplified in the following:
In flightdb.txt: Air Canada,AC102,YVR,2019-03-04T06:45:00,YYZ,2019-03-04T14:12:00
Air Canada,AC104,YVR,2019-03-04T08:00:00,YYZ,2019-03-04T15:27:00
Air Canada,AC107,YYZ,2019-03-04T10:00:00,YVR,2019-03-04T12:16:00
Air Canada,AC3,YVR,2019-03-04T13:45:00,NRT,2019-03-04T16:40:00
In this file, each line refers to the information of a flight. Each field of a flight is separated by a comma (,).
The fields are: airline, flight number, departure airport code, departure datetime, arrival airport code, arrival datetime.
For example, the first flight in this file is
Airline: Air Canada
Number: AC102
Departure airport code: YVR
Departure datetime: 2019-03-04, 06:45:00
Arrival airport code: YYZ
Arrival datetime: 2019-03-04, 14:12:00
Your program should read this file and save the flights in an array of flight.
After that, your system should support the following functions:
1. Print all flight information
2. Search flights by arrival city
3. Search flights by departure city
4. Search flights by flight number
About LocalDateTime
LocalDateTime is a built-in class in Java to represent a date-time without a time-zone in the ISO-8601 calendar system, such as 2007-12-03T10:15:30.
For example:
String timeString = "2007-12-03T10:15:30";
LocalDateTime aTime = LocalDateTime.parse(timeString);
System.out.println(aTime);
// output will be "2007-12-03T10:15:30"
1. You MUST create a Flight class to store the information of each flight.
2. All member variables in the Flight class MUST be private.
3. The Flight class MUST contain at least one useful method other than the setter/getter.
4. You MUST create a class containing the main method and using the Flight class.
5. The format to display a flight MUST follow the following example (in character level):
Airline: Air Canada
Flight Number: AC102
YVR(2019-03-04T06:45) --> YYZ(2019-03-04T14:12)
Sample Program
A sample run of the program.Bold and Italic texts refer to user inputs.
Database loaded successfully
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
1
--------------------
Airline: Air Canada
Flight Number: AC102
YVR(2019-03-04T06:45) --> YYZ(2019-03-04T14:12)
--------------------
Airline: Air Canada
Flight Number: AC104
YVR(2019-03-04T08:00) --> YYZ(2019-03-04T15:27)
--------------------
Airline: Air Canada
Flight Number: AC107
YYZ(2019-03-04T10:00) --> YVR(2019-03-04T12:16)
--------------------
Airline: Air Canada
Flight Number: AC3
YVR(2019-03-04T13:45) --> NRT(2019-03-04T16:40)
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
2
Arrival city airport >> YYZ
Airline: Air Canada
Flight Number: AC102
YVR(2019-03-04T06:45) --> YYZ(2019-03-04T14:12)
Airline: Air Canada
Flight Number: AC104
YVR(2019-03-04T08:00) --> YYZ(2019-03-04T15:27)
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
2
Arrival city airport >> NRT
Airline: Air Canada
Flight Number: AC3
YVR(2019-03-04T13:45) --> NRT(2019-03-04T16:40)
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
2
Arrival city airport >> DTW
No such a flight
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
3
Departure city airport >> YVR
Airline: Air Canada
Flight Number: AC102
YVR(2019-03-04T06:45) --> YYZ(2019-03-04T14:12)
Airline: Air Canada
Flight Number: AC104
YVR(2019-03-04T08:00) --> YYZ(2019-03-04T15:27)
Airline: Air Canada
Flight Number: AC3
YVR(2019-03-04T13:45) --> NRT(2019-03-04T16:40)
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
3
Departure city airport >> NRT
No such a flight
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
4
Flight number >> AC3
Airline: Air Canada
Flight Number: AC3
YVR(2019-03-04T13:45) --> NRT(2019-03-04T16:40)
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
4
Flight number >> AC5083
No such a flight
Please choose >>
1. Print all flight info
2. Search flight by arrival city
3. Search flight by departure city
4. Search flight by flight number
0. Exit
0
Thank you for using the system!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
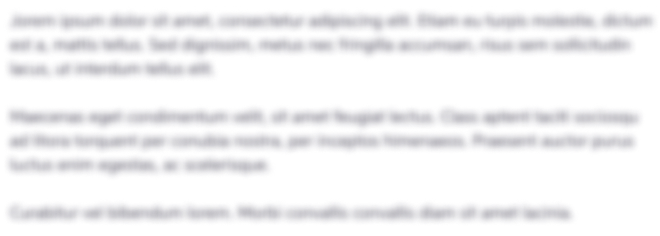
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started