Question
write a method in java that goes inside: public void removeAt(int pos) { } in the LinkedList class given below Given Linked List class import
write a method in java that goes inside:
public void removeAt(int pos) {
}
in the LinkedList class given below
Given Linked List class
import java.util.Iterator;
import java.util.NoSuchElementException;
public class LinkedList
private Node
/**
* Constructs an empty list
*/
public LinkedList() {
this.head = null;
}
/**
* Returns size of the list
*/
public int size() {
int size = 0;
if(head != null) {
size ++;
Node
while(current.next!= null) {
size ++;
current = current.next;
}
}
return size;
}
/**
* Returns true if the list is empty
*
*/
public boolean isEmpty() {
return head == null;
}
/**
* Removes all nodes from the list.
*
*/
public void clear() {
this.head = null;
}
/**
* Returns the data at the specified position in the list.
*/
public T get(int pos) {
if(head == null) throw new IndexOutOfBoundsException();
int currentIndex = 0;
Node
while(currentIndex
currentIndex ++;
current = current.next;
}
if(current != null) {
return current.data;
} else{
throw new IndexOutOfBoundsException();
}
}
/**
* Returns true if this list contains the specified element.
*
*/
public boolean contains(T x) {
for(T temp: this) {
if(temp.equals(x)) {
return true;
}
}
return false;
}
/**
* Returns the first element in the list.
*
*/
public T getFirst() {
if(head == null) throw new NoSuchElementException();
return head.data;
}
/**
* Inserts a new node at the beginning of this list.
*
*/
public void addToFirst(T data) {
this.head = new Node
}
/**
* Removes the first element in the list.
*
*/
public T removeFirst() {
T temp = getFirst();
head = head.next;
return temp;
}
/**
* Returns the last element in the list.
*
*/
public T getLast() {
if(head == null) throw new NoSuchElementException();
Node
while(current.next != null) {
current = current.next;
}
return current.data;
}
/**
* Inserts a new node to the end of this list.
*
*/
public void addToLast(T data) {
if(head == null) {
head = new Node
} else{
Node
while(current.next != null) {
current = current.next;
}
current.next = new Node
}
}
/**
* Removes the first occurrence of the specified element in this list.
*
*/
public void remove(T key) {
if(head == null) throw new NoSuchElementException();
if(head.data.equals(key)) {
head = head.next;
return;
}
Node
Node
while(current != null && !current.data.equals(key)) {
prev = current;
current = current.next;
}
if(current != null) {
prev.next = current.next;
} else{
throw new NoSuchElementException();
}
}
/**
* The Node class
*/
private static class Node
private T data;
private Node
public Node(T data, Node
this.data = data;
this.next = next;
}
}
/**
*
* The Iterator class
*/
@Override
public Iterator
return new LinkedListIterator();
}
private class LinkedListIterator implements Iterator
private Node
public LinkedListIterator() {
this.nextNode = head;
}
@Override
public boolean hasNext() {
return nextNode != null;
}
@Override
public T next() {
if(!hasNext()) throw new NoSuchElementException();
T d = nextNode.data;
nextNode = nextNode.next;
return d;
}
}
/**
* Returns a string representation
*
*/
@Override
public String toString() {
StringBuffer result = new StringBuffer();
for(Object x : this)
result.append(x + " ");
return result.toString();
}
/*
* *************************
* Homework Problems
*
* *************************
*/
/**
* Inserts a new node at pos.
*/
public void insertAt(int pos, T toInsert) {
}
/**
* Removes the item at position pos in this list.
*
*/
public void removeAt(int pos) {
}
/**
* Inserts a new node after a node containing the key.
*
*/
public void insertAfter(T key, T toInsert) {
}
/**
* Inserts a new node before a node containing the key.
*/
public void insertBefore(T key, T toInsert) {
}
/**
* sort the list
*/
public void sortList() {
}
/**
* removes duplicate elements from the list
*/
public void removeDuplicates() {
}
}
removeAt(pos): remove element at position pos. If position exceeds the length of the list throw an exception. Example: List: 467 259 removeAt(2) List: 462 59 removeAt(0) List: 62 59 removeAt(-1) IllegalArgumentException List: 6 2 59 removeAt(7) IndexOutOfBoundsException List: 62 59Step by Step Solution
There are 3 Steps involved in it
Step: 1
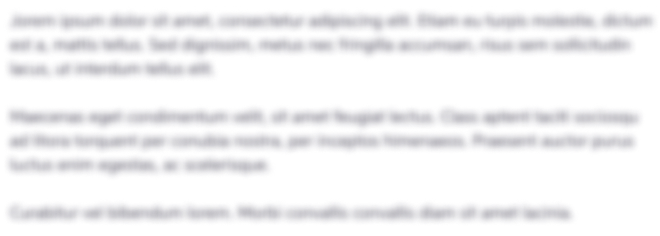
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started