Question
Write a mini web server using Python: It should listen on a random port between 10000 and 20000 - using TCP - on all interfaces
Write a mini web server using Python:
It should listen on a random port between 10000 and 20000 - using TCP - on all interfaces (IE: host = ) You can pick a random socket, but it does not need to be randomly generated.
Initially, the server app MUST display (print to the screen) the host and port it is listening on, and the services (keywords) it supports. If you would like to build on your assignment 1, it can list echo and exit among the services it supports, but new ones need to be added.
The app should be capable of receiving information from a web browser. It will be tested using Firefox, so make sure it works with at least Firefox.
The web browser will still send a message that your server can receive, only this time it will have a special format from the browser. It will be in the form of GET /
See example below:
$ python http-server.py
listening on: All
port: 10000
Available items: download, info, links, page1, exit
Waiting for client:
Recv Data: GET /links HTTP/1.1
Host: 127.0.0.1:10000
User-Agent: Mozilla/5.0 (X11; Linux x
Client requested downloads page
All the lines shown are generated from the server, but the information to the right of Recv Data: is the message received from the browser, with the following URL entered into FF:
http://localhost:10000/links
Your server should have the same output as my example above.
In addition, it needs to:
Output all browser requests with a Recv Data: output line on the server side
Output items back to the browser in the proper format, based on requests. See examples below. Proper format means that the HTML formatting should work, not just display the raw output in the browser. If there is RAW output then something is wrong with the header or communication with the browser. This is one of the main functions for this assignment, and is worth significant points.
The server must output the IP/port combination and the available items listed in the example.
The server must keep listening for new browser requests without needing to be restarted between requests.
The server should process the request and then close the client connection so that another browser request could be served immediately.
The app should check for the following words in the GET line from the browser, all the browser messages should be lowercase for ease of testing. See words below:
info : when info is received, the server should display an info page in HTML which will describe your program. Include your name, the class name, the server name, etc. You can make an info.html file containing your HTML data, or include the HTML data directly in your python server app. If you use a file, be sure to submit it with your assignment. This needs to work or points will be deducted.
page1 : when the page1 message is received, the server should output the contents of page1.html to the browser. This should produce the proper headers to display properly in the web browser.
link : when link is received, the server should output a download link for the download.zip file. See my hints section below for this link.
download : when download is received, the server should stream the download.zip file to the browser.
I will provide some header information and other hints which will GREATLY reduce the difficulty level of this assignment. Only skip them if you would like a significantly more difficult challenge completing this assignment. See the hints section below.
The only import lines should be import socket, import os and import sys. If you want to use something like time or regex, you are welcome to use that, but nothing network/internet/html/http based. Any other import will result in a major reduction in grade.
Comments are going to be a part of the grade on this assignment, so be sure to include comments to show what your code is doing.
Hints:
My code for reading a file in and creating the proper response header is below:
# read html file in
with open(filename.ext, 'r') as myfile:
filedata=myfile.read().replace(' ', '')
# obtain length of data to be sent
content_len=str(len(filedata))
# prepare HTML header
header="HTTP/1.0 200 OK Content-Length:"+content_len+" "
# Now, find a way to output all of it back to the browser, including the file
# contents. Be sure to send the header first.
This assignment requires a few files, including HTML and ZIP files. Make sure you have all the needed files in the same directory as your program, this will make things easier.
When sending data to the browser, you will likely need to shutdown the socket before closing it, with something like this:
It is also possible you might need:
time.sleep(0.01)
Without the shutdown (and possibly a sleep) you might receive a reset error or some other error in Firefox and other browsers, preventing the page from working. I found it did not affect all versions of FF, but it did for most.
To produce the download link:
# initial header
header="HTTP/1.0 200 OK Content-Length:200 "
# html link for download
header+=" Download File: download.zip"
note: I pulled the above lines from a function and modified slightly. They may require slight tweaking to work, but will probably work as-is.
When steaming the download.zip file, you will need to include a proper header.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
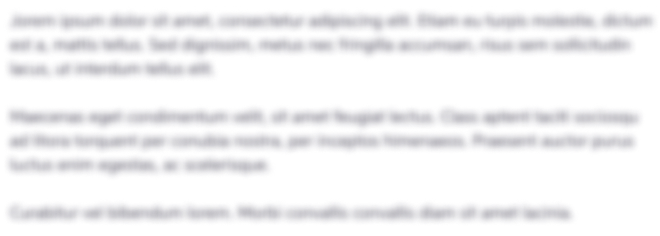
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started