Question
Write a MIPS program to remove a given element from an array. Below is an example of the C code segment to perform the above
Write a MIPS program to remove a given element from an array.
Below is an example of the C code segment to perform the above task. You may use a different code, but you have to write the code that you use as a comment before the MIPS code.
// Declaration of variables int* A; // Base address of array A int n; // Length of the array A int val; // Given value to be removed from array // Remove elements equal to val int i = 0; while (i < n) { if (A[i] != val) // If no match, proceed with next element i++; else { for (int k = i; k < n - 1; k++) // Shift elements if a match is found A[k] = A[k+1]; n = n - 1; // if element deleted, length of array decreases } }
Registers | Variables |
---|---|
$s0 | A |
$s1 | n |
$s2 | val |
Addresses | Contents |
---|---|
$s0 | A[0] |
$s0+4 | A[1] |
$s0+8 | A[2] |
$s0+4*(n-1) | A[n-1] |
You may use any temporary registers from $t0 to $t9 or saved registers from $s3 to $s7. Clearly specify your choice of registers and explain your code using comments.
Example Test: If the values of $s0 through $s2 and array elements are initialized in the simulator as:
(Use the '+' button under the Registers display to initialize register values for $s0, $s1, $s2 and the '+' button under the Memory display to initialize the initial array elements.)
Registers | Data |
---|---|
$s0 | 4000 |
$s1 | 10 |
$s2 | -15 |
Addresses | Contents |
---|---|
4000 | 4 |
4004 | 8 |
4008 | -15 |
4012 | 5 |
4016 | -10 |
4020 | -15 |
4024 | 0 |
4028 | -15 |
4032 | 40 |
4036 | -15 |
The resultant registers are:
Registers | Data |
---|---|
$s0 | 4000 |
$s1 | 6 |
$s2 | -15 |
The resultant array is:
Addresses | Contents |
---|---|
4000 | 4 |
4004 | 8 |
4008 | 5 |
4012 | -10 |
4016 | 0 |
4020 | 40 |
Only the registers $s0, $s1, $s2 and the first '$s1' elements in memory will be checked by the automated tests.
int A[100]; does not work
Step by Step Solution
There are 3 Steps involved in it
Step: 1
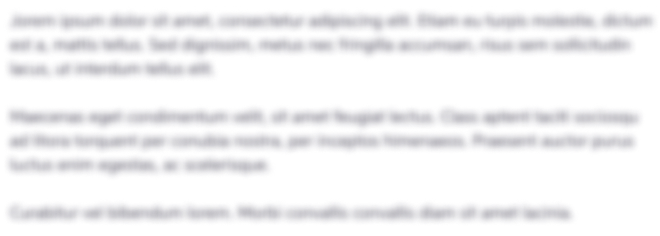
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started