Question
Write a program Caesar.java to perform a specific kind of encryption and decryption. In the classical Caesar cipher, each letter in the original text (also
Write a program Caesar.java to perform a specific kind of encryption and decryption.
In the classical Caesar cipher, each letter in the original text (also known as the cleartext) is replaced by a letter a certain number of letters down the alphabet to give the encrypted text (ciphertext). We will be using a Caesar cipher that substitutes each letter with the letter 13 positions down the alphabet, a scheme known as Rot13 (short for \"rotate\" 13 positions). In Rot13, an A in the cleartext becomes an N in the ciphertext, a B becomes an O, a C becomes a P, a D becomes a Q, and so on, according to the following table:
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
N O P Q R S T U V W X Y Z A B C D E F G H I J K L M
The nice thing about using 13 positions is that the same rot13 operation can be used for encrypting as for decrypting: HELLO WORLD encrypts to URYYB JBEYQ, and encrypting again gives back HELLO WORLD.
Specifically, your program must:
- have a rot13 method which uses a large switch statement to convert each of the uppercase alphabetic characters to its rot13 equivalent (returning unchanged any non-uppercase or non-alphabetic character).
- repeatedly request user input
- convert the input to upper case: if your input is in variable line, the expression line.toUpperCase() returns the upper case version of the line
- if the user input, after conversion to uppercase, is the string \"DONE\", exit the program.
- otherwise, call your rot13 method for each character in the string, and print it
Here is an example interaction
enter the cleartext: yes
the ciphertext is: LRF
enter the cleartext: ICS
the ciphertext is: VPF
enter the cleartext: Uryyb Jbeyq
the ciphertext is: HELLO WORLD
enter the cleartext: Done
Code I have so far:
importjava.util.Scanner;
publicclassCaesar {
publicstaticcharrot13(charc) {
switch(c) {
case'A' :return'N';
case'B' :return'O';
case'C' :return'P';
default:returnc;
}
}
publicstaticvoidmain(String[] args) {
Scanner in =newScanner(System.in);
System.out.println(\"Enter\");
String input = in.nextLine();
String trueinput = input.toUpperCase();
for(inti = 0; i
System.out.print(rot13(trueinput.charAt(i)));
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
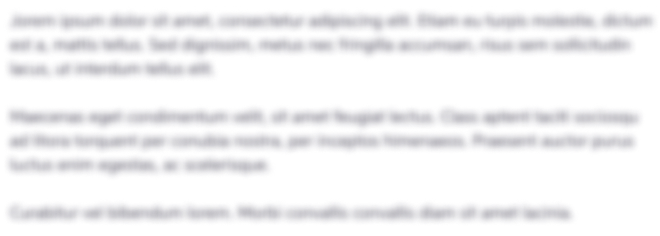
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started