Question
Write a program called MostAnagrams to find the word with the most anagrams. The input to your program is a single line containing a single
Write a program called MostAnagrams to find the word with the most anagrams.
The input to your program is a single line containing a single positive integer n, which is the maximum number of lines to read from words.txt. Your program should print a single integer, the maximum number of anagrams of the words in the first n lines of words.txt. (If n is greater than the number of lines in words.txt, then the output is the same as for n equal to the number of lines in words.txt.)
For this problem you may find the String method toCharArray(), the String con- structor that takes a character array argument, and the method java.util.Arrays.sort() useful. You may also use the Collections.sort() method.
Here is the starter code:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; import java.util.List; import java.util.ArrayList; import java.util.LinkedList; import java.util.Collections; public class MostAnagrams { public static void main(String[] args) { File file = new File("../resource/asnlib/publicdata/words.txt"); Scanner input = new Scanner(System.in); int maxWords = input.nextInt(); // the maximum number of lines to read int n=0; // for counting the number of words read try { Scanner scanner = new Scanner(file); while (scanner.hasNext()) { String line = scanner.next(); // read in the next word ++n; if(n >= maxWords) break; // Now do something with the word // } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } // compute max, the maximum number of analgrams for any word int max = 0; System.out.println( max); } }
Here is supplement code:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; import java.util.ArrayList; import java.util.List; import java.util.Collections; public class MostVowels { /** * Print out words in words.txt in order of the number of vowels. * */ public static void main(String[] args) { // We could change ArrayList to LinkedList with no other changes. ListwordList = new ArrayList (); File file = new File("words.txt"); try { Scanner scanner = new Scanner(file); while (scanner.hasNext()) { String line = scanner.next(); wordList.add(new Word(line)); } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } Collections.sort(wordList); for (Word w : wordList) { System.out.println(w.getWord()); } } }
public class Word implements Comparable{ private String word; private static final String vowels = "aeiou"; public Word(String wd) { word = wd; } public String getWord() { return word; } // Since this class implements Comparable, we must supply a compareTo method. public int compareTo(Word ow) { int thisVowelCount = 0; for (int i = 0; i < word.length(); ++i) { if (vowels.indexOf(word.charAt(i)) > -1) ++thisVowelCount; } String otherWord = ow.getWord(); int otherVowelCount = 0; for (int i = 0; i < otherWord.length(); ++i) { if (vowels.indexOf(otherWord.charAt(i)) > -1) ++otherVowelCount; } return thisVowelCount - otherVowelCount; } }
I cannot use what is not mentioned here. No hashmap or map.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
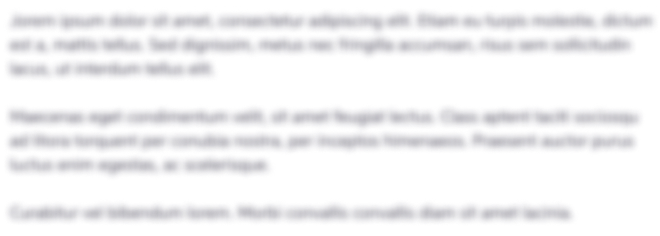
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started