Question
Write a program called RSA.java to implement RSA encryption/decryption. Specifically, your program should read several parameters, plaintext and ciphertext from a file named input.txt (in
Write a program called "RSA.java" to implement RSA encryption/decryption.
Specifically, your program should read several parameters, plaintext and ciphertext from a file named input.txt (in the same directory). Then your program should create a file named output.txt (in the same directory) and prints the private key information, encryption, and decryption results to output.txt. (Please check the attached sample files input.txt and output.txt.) For input.txt file: The first line is a prime number p (Hint: You may need use BigInteger datatype)
The second line is another prime number q
The third line is a randomly selected integer e, satisfying gcd(e, (n)) = 1, where (n) = ( p-1 ) * ( q-1 )
The fourth line is the plaintext M
The fifth line is the ciphertext C
For output.txt file: The first line is d satisfying ed mod (n) = 1
The second line is the ciphertext of the plaintext M in input.txt
The third line is the plaintext of the ciphertext C in input.txt
Here is my inputt.xt:
Does not work this code, Can I get an upgrade code please.
import java.io.DataInputStream;
import java.io.IoException;
import java.math.BigInteger;
import java.util.Random
public class RSA
{
private BigInteger p;
private BigInteger q;
private BigInteger n;
private BigInteger phi;
private BigInteger e;
private BigInteger d;
private int bitlength =1024;
private Random r;
public RSA()
{
r=new Random();
p=BigInteger.probableprime(bitlength, r);
q=BigInteger.probableprime(bitlength, r);
n=p.multiply(q);
phi=p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE));
e=BigInteger.probableprime(bitlength/2, r);
while (phi.gcd(e).compareTo(BIgInteger.ONE)> 0&& e.cmpareTo(phi)<0)
{
e.add(BigInteger.ONE);
}
d=e.modInverse(phi);
}
public RSA (BigInteger e, BigInteger d,BigInteger n)
{
this.e=e;
this.d=d;
this.n=n;
}
public static void main(string[] args)throws IoException
{
RSA rsa =new RSA();
DataInputStream in=new DataInputStream(System.in);
string teststring;
system.out.println("enter the plain text");
teststring=in.readline();
system.out.println("encrypted string:"+ teststring);
system.out.println("string in bytes"+bytesTostring(teststring.getbytes());
byte[] encrypted=rsa.encrypt(teststring.getbytes());
byte[] decrypted=rsa decrypt(encrypted);
system.out.println("decrypting bytes"+bytesTostring(decrypted));
system.out.println("decrypted string"+new string(decrypted));
}
private static string bytesTostriing (byte[] encrypted)
{
string test=" ";
for(byte b: encrypted)
{
test+=byte.tostring(b);
}
return test;
}
public byte [] encrypt (byte[] message)
{
return(new BigInteger(message)).modPow(e,n).tobytearray();
}
public byte [] decrypt (byte[] message)
{
return(new BigInteger(message)).modPow(d,n).tobytearray();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
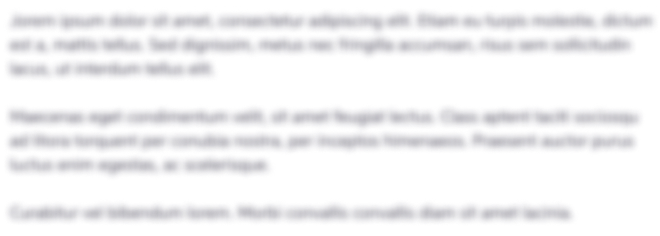
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started