Question
Write a program in a new file called split.cpp which reads a list of numbers into an array, splits the list into a list of
Write a program in a new file called split.cpp which reads a list of numbers into an array, splits the list into a list of even numbers and a list of odd numbers (each in their separate arrays) and then outputs the arrays and how many zeros were encountered in the input array. The program should use the command new to allocate arrays of exactly the correct size. The program should have a function count() for counting the number of even elements, the number of odd elements, and the number of zeros, a function split() for splitting the list into even and odd lists, and a function print_list() for printing an array of numbers. Run split_solution.exe to see an example of the program.
1. Read the lecture notes on Pointers before you start this lab! 2. Write your code incrementally! Compile, run, and test your code one function at a time. 3. A function prototype should be written for each function and placed BEFORE the main procedure. 4. All functions should be written AFTER the main procedure. 5. Each function should have a comment explaining what it does. 6. Each function parameter should have a comment explaining the parameter. You must implement the following algorithm in the main procedure to formulate your solution. Follow these instructions exactly, including specifications for functions, to receive full credit: 1. In the main procedure, prompt for the number of elements of the input list. 2. Allocate an array, using a pointer variable, whose size equals the number of elements specified by the user. 3. Read into the array the elements of the list. 4. Write a function count() which counts the number of even elements, the number of odd elements, and the number of zeros of an input array. The function must take four input parameters, the input array, the number of elements in the input array, the number of even elements (int), the number of odd elements(int). The function returns the number of zeros found in the input array (int). The function sets the number of even and odd elements. 5. In the main procedure, call the function count() to get the number of even elements, the number of odd elements, and the number of zeros in the array. 6. In the main procedure, allocate an array, using a pointer variable, whose size equals the number of even elements. 7. In the main procedure, allocate an array, using a pointer variable, whose size equals the number of odd elements. 8. Write a function split() which takes as input 3 arrays, A, B, and C, and stores the even elements of A in B and the odd elements of A in C. (Use better array names than A, B, and C). The size of array B should be exactly the number of even elements in array A. The size of array C should be exactly the number of odd elements in A. As you copy elements from A to B or C, count the number of elements copied to each array. When the function completes, check that the number copied equals exactly the array size. If it does not, print an error message and exit. The function should take six parameters, array A, the size of array A, array B, the size of array B, array C, and the size of array C. Determine where to use const in your definitions. The function modifies arrays B and C but does not return any value. 9. Write a function print_list() which prints the elements of an array passed into the function. The function should take two parameters, the array and the array length. The function does not modify or return any values. 10. In the main procedure, call the function split(), passing the input array and the arrays whose sizes equal the number of even and odd elements. 11. In the main procedure, print the phrase Even elements: . Print the array of even elements using the function print_list(). 12. In the main procedure, print the phrase Odd elements: . Print the array of odd elements using the function print_list(). 13. In the main procedure, free the three dynamically allocated arrays. (Points will be deducted if you do not free the arrays).
Here is my code, it is mostly correct but I am having trouble debugging it.
#include
// Function prototypes
void count(int A[], int size, int &even, int &odd, int &num_zeros);
void split(int A[], int size, int B[], int even ,int C[], int odd );
void print_list(int A[],int size);
// Main Function
int main() {
// Define Variables
int n;
int *A;
int *B;
int *C;
int even;
int odd;
int num_zeros;
cout << "Enter the number of elements: " ;
cin >> n;
while(n < 0){ cout<<"Can't enter negative numbers:"<
A = new int[n];
cout<<"Enter list:"<
split(A, n, B, even, C, odd);
cout << "Even elements: " << endl; cout << " "; print_list(B, even); cout << endl;
cout << "Odd elements: " << endl; cout << " "; print_list(C, odd); cout << endl;
cout << "There were " << num_zeros << " zeros in the list." << endl;
delete[] A; delete[] B; delete[] C; return 0;
} // Define Functions
// Count function
void count(int A[], int size, int & odd , int & even, int &num_zeros)
{ for (int i=0; i // Split function void split(int A[], int size, int B[], int even, int C[], int odd) { int i; int j; int k; for (int i=0; i void print_list(int A[], int size) { for(int i=0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
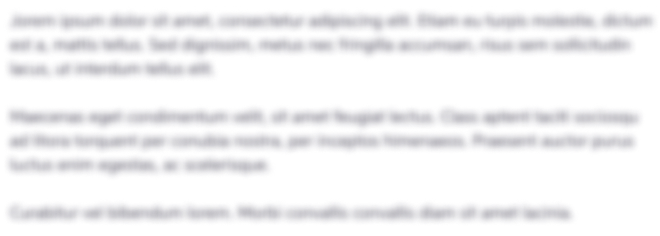
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started