Question
Write a program in C++ that generates and sorts an array of a user-specified size (user's array size) of randomly generated numbers. To keep the
Write a program in C++ that generates and sorts an array of a user-specified size (user's array size) of randomly generated numbers. To keep the array values to a reasonable range, employ the user's array size as the upper bound for generating the random numbers (that is, generate random numbers between 1 and user's array size). Your program should call the individual functions that implement three sorting algorithms (see the lecture slides and Google Drive demos). Each function should keep a count of the number of comparisons/exchanges it makes. Display the array before sorting, the sorted array, and the number of comparisons for each algorithm. NOTE: this is numbers of comparisons that you are counting; not the number of swaps/exchanges or assignments. Note: In this assignment, you will compare the three sorting algorithms (bubble sort or bubble sort++, selection sort, and insertion sort) with each other using the same data for each. Start with getting one to work first. The idea is that once you have one, adding in the other algorithms is relatively simple. Hint: use the algorithms from the slides! Your program MUST include a function for each algorithm and use them appropriately. The main procedural code must: Define a const int that limits the size of array the user can specify program max elements Create function prototypes for each sort (see following) Define three arrays of that size program max elements, one for each sort algorithm Prompt the user for the number of elements to store in the array user's array size ("How many elements in the array?"). Do not accept arrays smaller than 5 or larger than program max elements . Generate user's array size random integers between 1 and user's array size (the number they entered--yes, you will use it twice!) and store them in the same positions (0 to user's array size ) in each array. (Since each function will sort the array, changing the values, you will need to make multiple copies of the generated array to test the same numbers against each algorithm.) Implement each function as a stub that does nothing except Define an int variable for number of comparisons made and initialize appropriately print out the name of the function and that it is running put a comment: // here is the sorting algorithm print out the name of the function and that the function is returning with number of comparisons made return the value number of comparisons made Test that the program runs each of the stub sort functions Implement each algorithm will return the number of comparisons made. This is when one element is compared to another, regardless of whether or not they get swapped. You will need to include at least these functions: void displayArray(int values[], int size); int bubbleSort(int values[], int size); only do one bubble sort, either form is fine int bubblePPSort(int values[], int size); int selectionSort(int values[], int size); int insertionSort(int values[], int size); The output should look something like this: How many elements in the array? 20 BUBBLE SORT (maybe PLUS PLUS) Before sorting: 10 14 6 7 12 18 9 13 4 17 19 2 17 2 4 10 20 3 9 14 After sorting: 2 2 3 4 4 6 7 9 9 10 10 12 13 14 14 17 17 18 19 20 Number of comparisons: 190 SELECTION SORT Before sorting: 10 14 6 7 12 18 9 13 4 17 19 2 17 2 4 10 20 3 9 14 After sorting: 2 2 3 4 4 6 7 9 9 10 10 12 13 14 14 17 17 18 19 20 Number of comparisons: 206 INSERTION SORT Before sorting: 10 14 6 7 12 18 9 13 4 17 19 2 17 2 4 10 20 3 9 14 After sorting: 2 2 3 4 4 6 7 9 9 10 10 12 13 14 14 17 17 18 19 20 Number of comparisons: 186
Step by Step Solution
There are 3 Steps involved in it
Step: 1
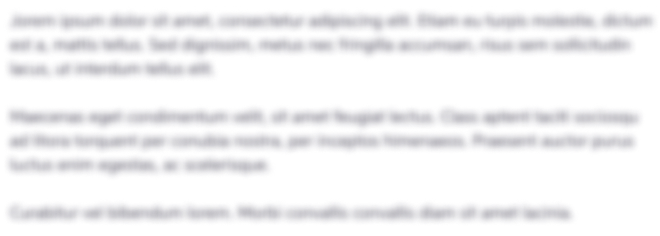
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started