Question
Write a program in C++ that places eight queens on a chessboard (8 x 8 board) such that no queen is attacking another. Queens in
Write a program in C++ that places eight queens on a chessboard (8 x 8 board) such that no queen is "attacking" another. Queens in chess can move vertically, horizontally, or diagonally.
How you solve this problem is entirely up to you. You may choose to write a recursive program or an iterative (i.e., non-recursive) program. You will not be penalized/rewarded for choosing one method or another. Do what is easiest for you.
3.1. Output
Below is one solution to the eight queens problem, there may be others. Your program only needs to find one solution, any solution, and print it. Assuming the name of your program is queens, executing your program should look like this:
z123456@turing:~/csci241/Assign2$ ./queens 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 z123456@turing:~/csci241/Assign2$
where a '1'; means a queen is on that square of the board and a '0' means the square is empty.
Note that it is very important that your output be formatted exactly as shown above. In grading your program, its output will be checked by another program (we wrote) that expects as input a solution in that format.
3.2. File You Must Write
Write the code for this assignment in a single file which must be called queens.cpp.
3.3. Hints
You might want to represent the chessboard using a 2-D array of integers or Boolean variables, e.g., int board[8][8] or bool board[8][8]. This array can be declared in your main() function or as a data member of a class that you write. Initialize the board by filling the array with 0s or false.
A general strategy is to solve the problem by starting with the top row of the chessboard and proceed down the chessboard one row at a time. Only place a single queen in each row. This eliminates the need to check for other queens on the same row (i.e., queens that could attack horizontally). Always start processing a row by attempting to place a queen in the leftmost column and moving to the right as necessary. When placing a queen in a particular row remember that it suffices to only check the rows above you. If the queen is safe, then place it on the board there (board[row][col] = 1; or board[row][col] = true;) and proceed to the next row. If it is not safe, then continue to move one column to the right until you find a safe spot or run out of columns.
There are two general ways you can solve the eight queens problem; recursively or iteratively. Below are some more general hints that use the suggestions above, first in a recursive algorithm and then in an iterative algorithm. Although two general algorithms are discussed below, remember that you are only required to write one solution to this problem. Write as many solutions as you would like, but submit only one program for grading. You may elect to use the hints from either section below, or you may decide to ignore all of them and design a solution on your own. Whatever you decide is acceptable.
3.3.1. Recursive Algorithm
You could write a recursive function called place_queens() that returns a Boolean value and accepts two arguments, the chessboard and a row index. If your chessboard is a data member of a class, then this will be a member function of that class and you will only need to pass it the row index.
The way to think about place_queens() is that it attempts to place all the queens from the row you specified and down. If it can place all the queens from that row down, it returns true and leaves the queens on the chessboard. If it couldn't then it returns false and removes all the queens from that row down. This way, after you initialize the chessboard, you may make the single call from your main() routine like this place_queens(board, 0) (for a function) or this q.place_queens(0) (for a member function, assuming q is an instance of the class you defined). Of course, you need to check the return value of the function for success or failure.
place_queens() always starts by attempting to place a queen in the leftmost column of the row it received as an argument and checking that it is safe from all the queens in the rows above it. If it is not safe, then proceed by moving the queen one column to the right and checking that square. If you get to the right end of the board without finding a safe spot, then return false. If you find a safe spot, then place the queen on the board and call place_queens() again (recursively) asking it to place all the queens on the rows below yours (i.e., passing it the same board it received but incrementing the row index by 1).
Test the return value of that recursive call. Recall that place_queens() will attempt to place all the queens in the rows below and return either false or true based its success. If the return value is true, then simply return true yourself and you are done. If it is false, then there was no way to place the other queens on the board. You must try and find another safe column (to the right) in the same row. Move the queen in this row to the right, one column at a time, until you find another square where the queen is safe from all the queens above it. If you find another safe column, place the queen there and make another recursive call to place_queens(). If there are no more safe columns in this row, then remove the queen from this row and return false.
The stopping condition for this recursive algorithm is when you enter place_queens() and the row that you have been passed is greater than 7 (assuming the top row is row 0).
3.3.2. Iterative Algorithm
Start by placing a queen in the leftmost column of the top row. Since there are no rows above the top row, there are no "attacking" queens to check, so proceed to the second row. As you move from the first row to the second row we call this "approaching a row from the top". This is differs from "approaching a row from the bottom" (described below).
When approaching a row from the top there are no queens on that row. Start by attempting to place the queen in the leftmost column and checking all the rows above. If the queen is safe in that column, place it on the board and proceed to the next row. If it is not safe, try the next column to the right and check there. If you get to the end of the row without finding a safe column for the queen, you must back up a row. This requires you to go back to the previous row and move that queen to the right. This is what is meant by "approaching the row from the bottom".
When approaching a row from the bottom, there is already a queen placed on that row and it is already safe from all the queens above it. The problem is that no more queens could be placed on the board in the rows below it. So, this queen must be moved from its safe spot to another safe spot in the same row. All the columns to the left of this queen have already been checked. The only place for this queen to go is to the right. Start by trying one square to the right and checking the rows above. If it is safe, place the queen there and proceed to the row below approaching it from the top. If it is not safe, keep moving the queen to the right. If you run out of columns, then remove the queen from the board and back up to the row above approaching it from the bottom.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
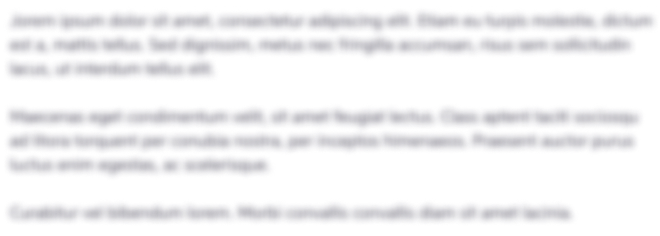
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started