Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a program in C + + to create a Binary Search tree ( BST ) of integers. The program will perform these operations: Insert
Write a program in C to create a Binary Search tree BST of integers. The program will perform these operations: Insert nodes Traverse Postorder, Search node, Delete node, Leaf Count, Children of a node and Quit.
Use the header file like this:
#include
#ifndef BTH
#define BTH
using namespace std;
class BT
private:
struct node
int data;
node left;
node right;
;
node root;
public:
BT; Constructor
bool isEmpty const return root NULL; Check for empty
void insertint; Insert item in BST
void printpostorder; Inorder traversing driver
void postorderTravnode; Inorder traversing
void searchBSTint; Searches BST for a specific node
void deleteNodeint; Delete item from BST
int count; Count driver
int leafCountnode; Counts number of leaves in BST
void nodeChildrenint; Finds children of a node
;
#endif
Use the following menu in your program. Must place your name in the menu heading.
MENU YOUR NAME
Insert nodes
Traverse Postorder
Search node
Delete node
Leaf Count
Children of a node
Quit
Enter your choice:
Use the following characters in the given order to form the BST tree:
Hints: Before you start working on the program, you draw the BST for the given order of the above numbers. It will help you check your output for the options in the menu. While working on the program, test with few items such as You work on one menu at a time then it will be easier to test and manage the program instead of writing the whole program for entire menu then test your program.
Option : Inserts nodes in a BST
Enter Your Choice
Enter number of items to insert:
Enter node:
Inserted.
Enter node:
Inserted.
Enter node:
Inserted.
Enter node:
Inserted.
Option : Traverse the tree in Postorder and display the node info and its left child info and right child info. If a node does not have a child, then display NIL.
Enter Your Choice
Sample output:
Traversing Postorder
Node Info Left Child Info Right Child Info
NIL NIL
NIL
NIL NIL
Option : Search for the item in the BST
It will prompt: Enter item you want to search for:
If the item is found, then display the message is found in the BST If the item is not found then it will display is not found in the BST
is found in the BST
Option : Deletes a node from the BST
It will prompt: Enter item you want to delete:
If the item is found then delete the item from the BST and displays the message is deleted If the item is not in the BST then it will display is not found in the BST
is deleted
Option : Counts the number of leaves in the BST and displays
There are number of leaves in the BST
There are number of leaves in the BST
Option : Enter the item and it will display the children of that item.
It will prompt: Enter the item you want to display the children of:
Left child:
Right child:
If the item has no children, then it displays:
has no children.
Ex: Enter the item you want to display the children of:
Left child: NIL
Right child:
Option : Quit the program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
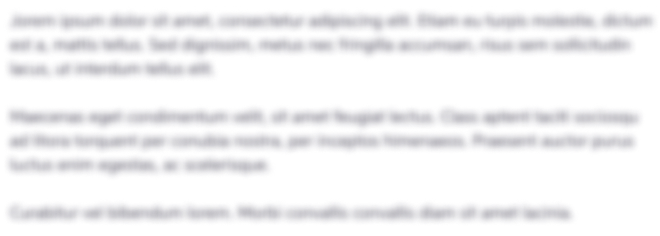
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started