Question
Write a program named FootballDistanceCalculator that calculates and outputs the distance a football travels when thrown at angles ranging from 10 - 90 degrees with
Write a program named FootballDistanceCalculator that calculates and outputs the distance a football travels when thrown at angles ranging from 10 - 90 degrees with initial velocities of 30, 40, 50, and 60 miles per hour from a height of 5 ft above the ground. To keep things simple, you will use the projectile distance formula given below and ignore things like wind resistance and how much the football wobbles.
You will display your output in a table, with each row representing a different angle and columns used to show the distances for the four different initial velocities. Your output format should be similar to that shown below. Your values should exactly match the values given below, but include angles of 10, 20, 30, 40, 50, 60, 70, 80, and 90 degrees.
csc$ java FootballDistanceCalculator Distance Traveled(feet) (when thrown at a height of 5.0 feet) Angle(deg) 30(mph) 40(mph) 50(mph) 60(mph) ---------- ------- ------- ------- ------- 10 36.55 55.34 77.96 104.63 20 49.43 80.50 119.76 167.41 . . . . . . . . . . . . . . . 90 0.00 0.00 0.00 0.00
Projectile Distance Formula
d=vcosg(vsin+(vsin)2+2gy0)d=vcosg(vsin+(vsin)2+2gy0)
v - initial velocity of projectile
y0 - initial height of projectile
g - gravitational acceleration
- angle at which the projectile is thrown
d - horizontal distance traveled by the projectile
Source: http://en.wikipedia.org/wiki/Trajectory_of_a_projectile
Design Your Software
The main method of your program must call the method, calculateDistance(), which must be defined and documented as shown below:
/** * Calculates the distance a projectile travels * * @param angle angle at which projectile is thrown in degrees * @param velocity initial velocity of projectile in miles/hour * @param height initial height of projectile in feet * @return distance traveled by projectile in feet */ public static double calculateDistance(double angle, double velocity, double height) { }
The calculateDistance() method must use the formula given above to calculate the distance based on the given angle, initial velocity, and height. The method will return the distance to the main method, which will output a table listing the angles and distances for initial velocities of 30, 40, 50, and 60 miles per hour, as shown above.
The main method must call the calculateDistance() method from within a nested for loop and output each value that is returned to form the table.
Implement Your Software
Your calculateDistance() method must return the correct distance as a double for all reasonable input values, not just the ones used to create the table above.
Angle Calculation
NOTE that this method must convert the angle from degrees to radians in order to use the Math class trigonometric methods.
Output the distances in the table with 2 decimal places using a printf statement to format the value. For example, the following statement formats the distance so that it has 2 places after the decimal point and takes up a total of 9 spaces:
System.out.printf("%9.2f", distance);
The following statement may be used to output an angle so that it is right-justified and takes up a total of 6 spaces:
System.out.printf("%6d", angle);
Use the following named constants in your program rather than magic numbers - the angles and velocities are given as ints to avoid roundoff error:
public static final double GRAVITATIONAL_ACCELERATION = 32.174; //feet per sec^2 public static final int MIN_ANGLE = 10; //degrees public static final int MAX_ANGLE = 90; //degrees public static final int ANGLE_INCREMENT = 10; public static final int MIN_VELOCITY = 30; //miles/hour public static final int MAX_VELOCITY = 60; //miles/hour public static final int VELOCITY_INCREMENT = 10; public static final double HEIGHT = 5; //feet public static final double FEET_PER_MILE = 5280; public static final double SEC_PER_HOUR = 3600;
You may use numbers for formatting in your printf statements as well as the numbers 0, 1, 2, and 100 without defining them as a class constants.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
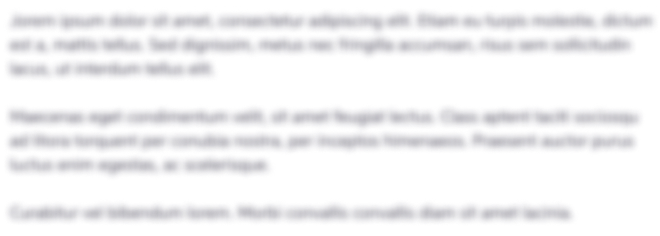
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started