Question
Write a program that prompts the user to enter a number. The number will always be >= 0 and
Write a program that prompts the user to enter a number. The number will always be >= 0 and <= 999. You can assume that the user will always supply you with an integer within this range.
Next, identify each digit in the number. Your program should be able to identify the number that exists in the ones place, the hundreds place and the thousand's place.
After this your program should generate a table that shows all possible combinations of the number if the digits were to be shifted around. For example, if the number is123your program should be able to produce the numbers132,213,231,321and312. Present this information in a tabular format with each combination appearing in the left column of the table. Finally, add two additional columns that shows the number in that row doubled and tripled. Here are a few sample runnings of your program. User input is underlined for your reference - you do not need to generate underlines in your own program:
Enter a 3 digit number between 000 and 999: 123 Digit in the 1's place: 3 Digit in the 10's place: 2 Digit in the 100's place: 1 Combination Doubled Tripled 123 246 369 132 264 396 213 426 639 231 462 693 321 642 963 312 624 936 Enter a 3 digit number between 000 and 999: 972 Digit in the 1's place: 2 Digit in the 10's place: 7 Digit in the 100's place: 9 Combination Doubled Tripled 972 1944 2916 927 1854 2781 792 1584 2376 729 1458 2187 279 558 837 297 594 891 Enter a 3 digit number between 000 and 999: 43 Digit in the 1's place: 3 Digit in the 10's place: 4 Digit in the 100's place: 0 Combination Doubled Tripled 043 86 129 034 68 102 403 806 1209 430 860 1290 340 680 1020 304 608 912 Enter a 3 digit number between 000 and 999: 1 Digit in the 1's place: 1 Digit in the 10's place: 0 Digit in the 100's place: 0 Combination Doubled Tripled 001 2 3 010 20 30 001 2 3 010 20 30 100 200 300 100 200 300 Enter a 3 digit number between 000 and 999: 999 Digit in the 1's place: 9 Digit in the 10's place: 9 Digit in the 100's place: 9 Combination Doubled Tripled 999 1998 2997 999 1998 2997 999 1998 2997 999 1998 2997 999 1998 2997 999 1998 2997
Hint: Think about how you isolate the "ones" digit - is there a math operation that you can use to extract this information? Huge hint: what happens when you divide the number by 10? Does that give you any usable information? How about the remainder of this division operation? Once you isolate the "ones" digit you then need to move on to the "tens" digit. One way to do this would be to "throw away" the ones digit so you can focus your attention on the "tens" digit. Is there a way you can do this using a math operation?
You may not use any advanced techniques that we have not covered to solve this problem (i.e. if you've read ahead and you have learned about string or list indexing / slicing you may not use these to solve this problem). You may, however, use thestrfunction if desired. In short, any of the following techniques are allowed:
- Math operations (+, -, *, /, //, %)
- Theprintfunction
- Theformatfunction
- Thestr,intorfloatfunctions
Your program should be formatted exactly as the one above. For your reference, each column in the table is 15 characters wide.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
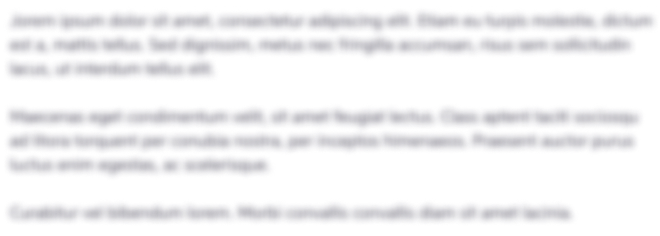
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started