Question
Write a program that reads a text file and computes the area of a polygon from the contents of the text file. This program needs
Write a program that reads a text file and computes the area of a polygon from the contents of the text file. This program needs to be written in python, the goal is:
- For each polygon/rectangle, create the appropriate object, request that each object report its vertices, its perimeter, and its area.
-For the code (that I will paste below), need to modify the Polygon.py code to add a function to compute the area
- modify the constructor in the Polygon.py code to accept a list of vertices as opposed to hard coding the vertices.
-modify the Rectangle code so that length and width are computed, rather than assigned, and so that the area is computed with an improved function for computing the area: 2*(length*width).
Below is the code. I have commented and signaled where my code that I am working on its. However I am not sure that the way I am computing the area is correct. Should I use numpy?
******POLYGON.PY*******
************************************************************************
import math
import itertools
from Point import Point
class Polygon(object):
def __init__(self):
self.vertices=[Point(5.0,6.0),Point(5.0,10.0),Point(11.0,10.0),Point(11.0,6.0)]
def translate(self,a=0.0,b=0.0):
for p in self.vertices:
Point.translate(p,a,b)
def scale(self,sx=0.0,sy=0.0):
for p in self.vertices:
Point.scale(p,sx,sy)
def rotate(self,theta_in_degrees=0.0):
for p in self.vertices:
Point.rotate(p,theta_in_degrees)
####### My Modified Code ########
def polygon_area(self):
area = 0
for index in range(len(self.vertices)-1):
v1 = self.vertices [index]
v2 = self.vertices [index + 1]
area += ((v1[0] * v2[1]) - (v2[0] * v1[1]))
area = 0.5 * area
####### End modified code ########
def __str__(self):
s=""
for p in self.vertices:
# s=s+" "+str(p.x)+" "+str(p.y)+" "
s=s+" "+"{:.2f}".format(p.x)+" "+"{:.2f}".format(p.y)+" "
return(s)
#using itertools.cycle
def perimeter(self):
""" convert a list of points into a list of distances """
print ("Using Polygon's perimeter routine")
distances = []
circular_buffer = itertools.cycle(self.vertices)
previous_point = next(circular_buffer)
for i in range(len(self.vertices)):
point = next(circular_buffer)
d = point.distance(previous_point)
distances.append(d)
previous_point = point
return "{:.2f}".format(sum(distances))
***********************************
DATA.TXT
P 5
1.7 4.9
6.1 6.2
7.0 2.8
4.8 0.1
1.5 1.4
R 4
7.0 5.0
1.0 5.0
1.0 3.0
7.0 3.0
P 4
4.1 5.4
6.9 2.5
2.9 0.8
0.9 2.5
P 3
1.2 4.7
6.5 4.2
4.0 1.7
************************************************
RECTANGLE.PY
*************************************************
from Polygon import Polygon
class Rectangle(Polygon):
def __init__(self):
super(Rectangle,self).__init__()
# self.length=6.0 this is the original code
# self.width=4.0 this is the original code
self.length = in_file.readline(0).split() #this is what I modified
slef.width = in_file.readline(0).split() #what i modified
def perimeter(self):
print ("Using Rectangle's perimeter routine")
return 2*self.length+2*self.width
************************************************************
RECTANGLEROOT.PY
************************************************************
from Rectangle import Rectangle
######MODIFIED
myrectangle = Rectangle()
in_file = open(project01data.txt)
line = in_file.readline()
###### /MODIFY
print ()
print ("the four vertices of the 'hard-coded' myrectangle are ...")
print(myrectangle)
print ("the manually computed length of myrectangle is ... 6")
print ("the manually computed width of myrectangle is ... 4")
print ()
print ("translating myrectangle by (2.0,3.0)...")
Rectangle.translate(myrectangle,2.0,3.0)
print(myrectangle)
print ("translating myrectangle by (-2.0,-3.0)...")
Rectangle.translate(myrectangle,-2.0,-3.0)
print(myrectangle)
print ("scaling myrectangle by (0.5,0.5)...")
Rectangle.scale(myrectangle,0.5,0.5)
print(myrectangle)
print ("scaling myrectangle by (2.0,2.0)...")
Rectangle.scale(myrectangle,2.0,2.0)
print(myrectangle)
print ("rotating myrectangle by 60 degrees...)")
Rectangle.rotate(myrectangle,60)
print(myrectangle)
print ("rotating myrectangle by -60 degrees...")
Rectangle.rotate(myrectangle,-60)
print(myrectangle)
print ("perimeter of myrectangle is ...")
print (myrectangle.perimeter())
******************************************************************
Please help me figure out what my next steps are. I have created a few modifications but I am unsure how to edit the hard code. The program needs to read the .txt file to get the info that it needs to do all of it's computing!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
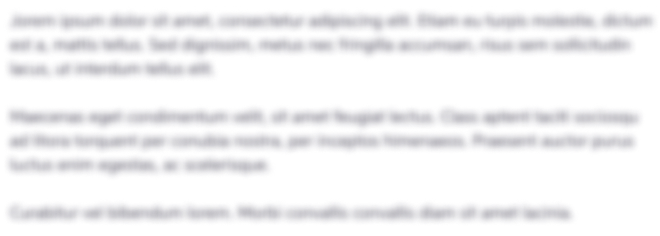
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started